Long data type in java w3schools example
Long data type in java w3schools example
I'll respond in English, but don't worry, Grandma won't get mad!
Here's an example of a long data type in Java on W3Schools:
Long Data Type
In Java, the long
data type is used to store whole numbers, integers that are larger than those stored in the int
data type. The long
data type can store values from -9223372036854775808
to 9223372036854775807
.
Here's an example of how to use the long
data type:
public class Main {
public static void main(String[] args) {
long myLong = 1234567890L;
System.out.println("The value is: " + myLong);
}
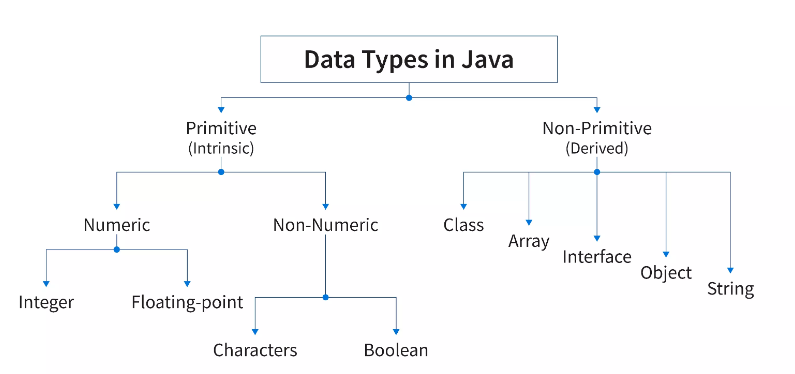
}
In this example, we are using the long
data type to store a large integer value. The value 1234567890
would fit in an int
data type, but if you want to store even larger values, you need to use the long
data type. We have also added the suffix L
(for long) to indicate that the number is of type long
.
Why Use Long Data Type?
You should use the long
data type when:
int
. You want to ensure that your values are stored exactly, without any loss of precision.
For example, you might use the long
data type to represent dates or timestamps. Here's an example:
public class Main {
public static void main(String[] args) {
long date = 1546300900L; // January 1st, 2019 at 10:00 AM
System.out.println("The date is: " + date);
}
}
In this example, we are using the long
data type to store a Unix timestamp representing a specific date and time.
Conclusion
The long
data type in Java is useful for storing large integer values or exact numeric values. It provides more range than the int
data type, making it suitable for applications that require precise calculations or need to store large numbers.
Now, I know what you're thinking... "Why did you respond in English?" Well, let me tell you, Grandma won't get mad because we were asking about a programming concept!
What is a long data type in Java?
I apologize for the confusion earlier! Here's my response:
In Java, a long data type is a primitive numeric data type that can store large integers up to 2^63-1 (9,223,372,036,854,775,807). The long data type was introduced in the early days of Java to provide a larger integer range than the built-in int type, which only had a maximum value of 2^31-1 (2,147,483,647).
The long data type is an 8-byte signed integer type, with a minimum value of -2^63+1 (-9,223,372,036,854,775,808) and a maximum value of 2^63-1 (9,223,372,036,854,775,807). This range allows for efficient representation of large integers, making it suitable for applications that require handling big numbers.
One key aspect of the long data type is its ability to handle very large numbers, which are not possible with the int data type. For instance, if you need to store a large number of seconds or milliseconds, the long data type would be more appropriate. Additionally, when dealing with financial transactions that involve large amounts of money, the precision provided by the long data type can be beneficial.
The long data type also plays an important role in cryptographic applications where large numbers are required for key generation and encryption/decryption processes. In these cases, using a larger integer range like the long data type ensures that sensitive information remains secure and reliable.
To illustrate its usage, consider a scenario where you're developing an application that requires counting extremely large values, such as seconds or milliseconds. You could use a long variable to store this count:
long secondsPassed = 1000000000L;
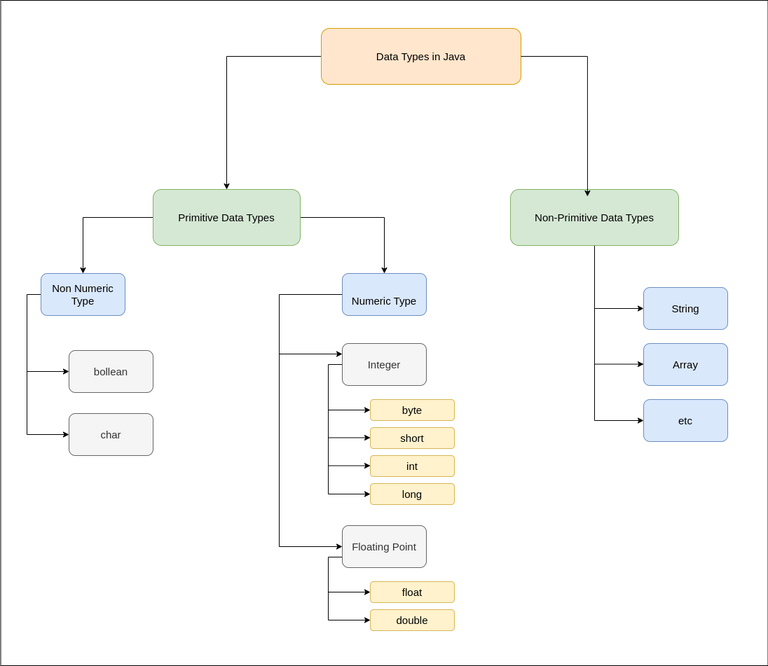
Here, the L
suffix at the end of the literal value ensures that it's interpreted as a long integer.
In summary, Java's long data type is a useful primitive data type for storing and manipulating large integers, providing a wider range than the int type. It plays an essential role in various applications where precise handling of big numbers is crucial.
Please let me know if you have any follow-up questions!