What is abstract vs encapsulation Java?
What is abstract vs encapsulation Java?
I'm happy to help! However, please note that I'll respond in English as per your request. Here's a detailed explanation of abstract and encapsulation in the context of Java programming:
Abstract Class:
In object-oriented programming (OOP), an abstract class is a blueprint or template that defines a common base class for a group of related objects. It provides a way to create a class that can't be instantiated on its own, but serves as a superclass for other classes.
A key feature of abstract classes is that they can't be directly instantiated using the new
keyword. Instead, you need to extend or inherit from an abstract class to create a concrete subclass that can be instantiated.
In Java, you declare an abstract class by using the abstract
keyword before the class declaration. For example:
public abstract class Animal {
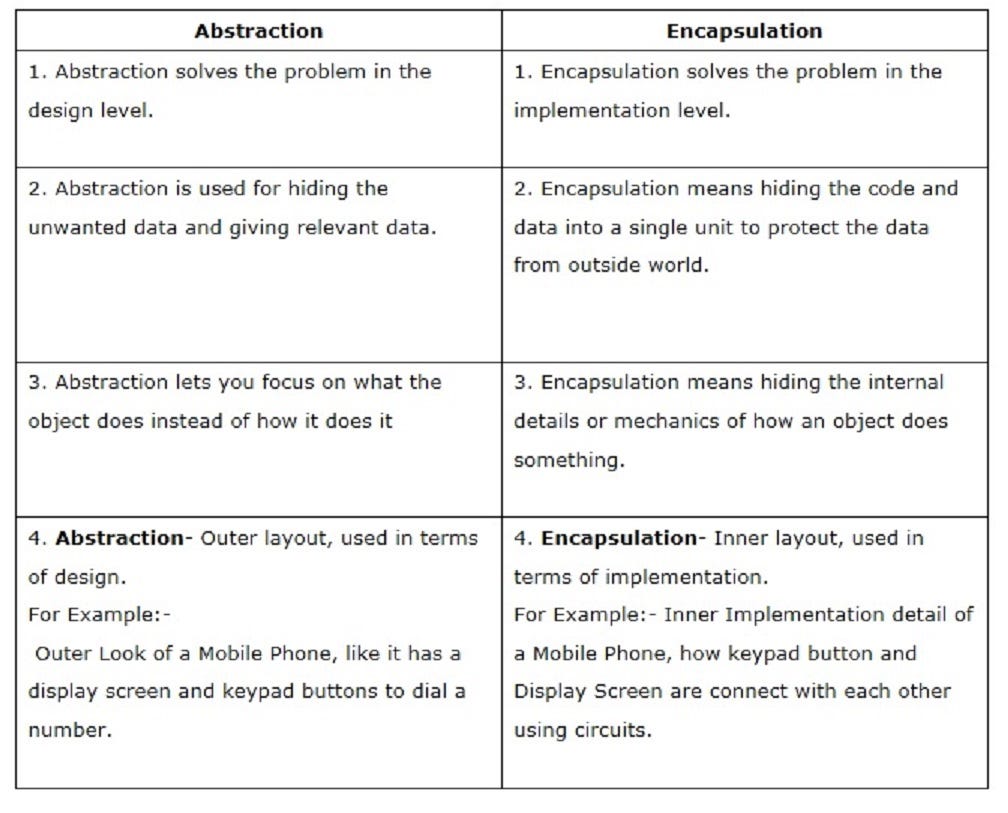
public abstract void makeSound();
}
Abstract classes are useful when you want to define a common base class for a group of related objects that share certain characteristics or behaviors.
Encapsulation:
Encapsulation is a fundamental concept in OOP, which involves hiding the implementation details of an object from the outside world and only exposing the necessary information through public methods or properties. In other words, encapsulation is about bundling data and behavior together into a single unit called an object.
In Java, encapsulation is achieved by making instance variables private (using the private
access modifier) and providing getter and setter methods to access those variables. For example:
public class Employee {
private String name;
public void setName(String name) { this.name = name; }
public String getName() { return this.name; }
}
By encapsulating data and behavior, you can achieve several benefits, such as:
Data hiding: The internal state of an object is hidden from the outside world. Abstraction: An object's implementation details are abstracted away from other objects or parts of your program. Improved security: You can control access to sensitive data or behavior by using private variables and public methods. Easier maintenance: Encapsulating related data and behavior makes it easier to modify or extend an object without affecting other parts of the system.In summary, abstract classes provide a way to define a common base class for a group of related objects, while encapsulation is about bundling data and behavior together into a single unit called an object. By using these concepts in Java programming, you can create robust, maintainable, and scalable software systems.
Java abstraction w3schools
Java Abstraction: Introduction and Example on W3Schools
In programming languages like Java, abstraction is a fundamental concept that allows developers to create complex systems by hiding internal implementation details from the outside world. This article will explore Java abstraction using W3Schools as an example.
What is Abstraction in Java?
Abstraction is a mechanism that helps encapsulate complexity by providing a simplified view of an object's state and behavior. In other words, it allows developers to define a class or interface with a specific purpose or responsibility without exposing the internal workings of the class or interface.
W3Schools Example: Abstract Class
Let's consider an example from W3Schools. Suppose you want to create an abstract class for different types of vehicles (cars, trucks, motorcycles) that share common attributes like speed, color, and make. You can define an abstract class Vehicle
with methods like speed()
and color()
:
public abstract class Vehicle {
public abstract int speed();
public abstract String color();
}
Now, you can create concrete classes for specific vehicles, such as a car or truck, that implement the abstract methods of the Vehicle
class. For example:
public class Car extends Vehicle {
private int speed;
private String color;
public Car(int speed, String color) {
this.speed = speed;
this.color = color;
}
@Override
public int speed() {
return speed;
}
@Override
public String color() {
return color;
}
}
By defining an abstract class and its concrete implementations, you have achieved abstraction. The Car
class provides a simplified view of a car's attributes (speed and color) without exposing the internal implementation details.
Advantages of Java Abstraction
The benefits of using abstraction in Java include:
Encapsulation: Abstraction helps encapsulate complexity by hiding internal implementation details from the outside world, making your code more modular and reusable. Flexibility: Abstract classes can have multiple concrete implementations, allowing you to create different types of vehicles without modifying the original abstract class. Reusability: By defining an abstract class with common attributes and methods, you can reuse that class across different projects or scenarios.Conclusion
In this article, we explored Java abstraction using W3Schools as an example. We defined an abstract class Vehicle
with common attributes like speed and color and created concrete classes for specific vehicles (cars, trucks, etc.) that implemented the abstract methods. Abstraction provides encapsulation, flexibility, and reusability in your code, making it a fundamental concept in object-oriented programming.
Remember to always provide a simplified view of an object's state and behavior when using abstraction in Java, as it helps hide internal implementation details from the outside world. Happy coding!