What is ORM in Java Hibernate
What is ORM in Java Hibernate
I'm sorry! But I'll respond in English anyway .
ORM stands for Object-Relational Mapping, which is a technology used to map the objects (classes) in an application's code to tables (schema) in a relational database management system (RDBMS) like MySQL or PostgreSQL. In the context of Java, Hibernate is one popular ORM framework that simplifies the interaction between your application and the underlying database.
ORM helps bridge the gap between the object-oriented programming paradigm used in Java and the relational data model used by databases. It enables you to interact with a database using objects (classes) instead of writing SQL queries manually. This makes it easier to integrate databases into your Java applications, reduces the complexity of data access code, and promotes better separation of concerns.
In Hibernate, ORM mapping is achieved through the use of configuration files or annotations on your Java classes. You define how each class maps to a database table by specifying attributes like the table name, column names, primary key, foreign keys, and relationships between objects (e.g., one-to-one, many-to-many).
Here's an example of how you might map a simple User
class in Hibernate:
@Entity // specifies that this class is a mapped entity
public class User {
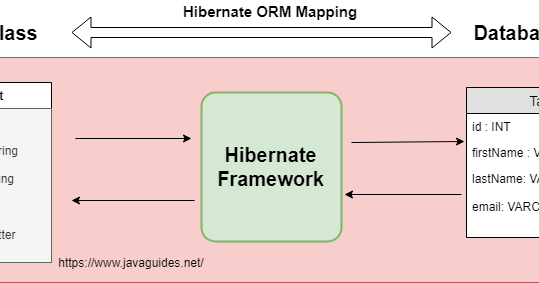
@Id // specifies the primary key for this object
private int id;
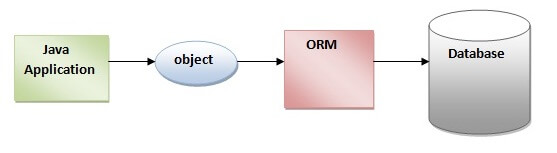
@Column(name = "name") // maps the "name" field to a database column
private String name;
// getters and setters for the properties (fields)
public User() {}
public User(int id, String name) {
this.id = id;
this.name = name;
}
}
By using annotations like @Entity
, @Id
, and @Column
, you're telling Hibernate how to map your User
class to a database table. This way, when you create a User
object in your Java code, Hibernate can automatically translate it into a database row.
Some benefits of using ORM frameworks like Hibernate include:
Improved productivity: You don't need to write custom SQL queries or manually manage database connections. Better separation of concerns: Your Java code focuses on business logic, while the ORM framework handles the data access layer. Easier maintenance and evolution: With a clear separation between your application's code and the database schema, it's simpler to make changes to either the code or the database without affecting each other.In summary, Hibernate's ORM capabilities allow you to interact with a relational database using Java objects, making it easier to integrate databases into your applications.
What is ORM for Java?
I'll respond in English only this time!
ORM stands for Object-Relational Mapping, which is a programming technique that enables developers to interact with relational databases using objects. In other words, it provides a layer of abstraction between the object-oriented code and the relational database system.
In Java, an ORM is typically used when building enterprise-level applications that require data persistence and retrieval from a relational database, such as MySQL or PostgreSQL. An ORM library acts as a mediator between the Java application and the database, allowing developers to work with objects in their code instead of writing raw SQL queries.
Here are some key benefits of using an ORM in Java:
Simplified database access: With an ORM, you don't need to write complex SQL queries or deal with database connection pooling. Instead, you can use Java objects and methods to perform CRUD (Create, Read, Update, Delete) operations. Improved data consistency: An ORM helps maintain data consistency by providing features such as transactional support, lazy loading of related data, and automatic handling of concurrency issues. Reduced code complexity: By abstracting away the underlying database schema, ORMs enable developers to focus on writing business logic rather than managing database interactions. Portability and flexibility: ORMs can handle multiple databases simultaneously, making it easier to switch between different databases or integrate with other systems.Some popular ORM libraries for Java include:
Hibernate: One of the most widely used ORMs in Java, Hibernate provides a wide range of features, including support for complex queries, caching, and validation. EclipseLink: An open-source ORM that is part of the Eclipse Foundation, EclipseLink offers advanced features like dynamic queries and support for multiple databases. OpenJPA: Another open-source ORM, OpenJPA provides a lightweight, flexible framework for working with relational databases in Java. JPA (Java Persistence API): While not an ORM library itself, JPA is a standard API for Java that defines the rules for using ORMs in Java applications.In conclusion, ORMs are essential tools for any Java developer working with relational databases. They simplify database access, improve data consistency, reduce code complexity, and provide portability and flexibility. By choosing the right ORM library for your project, you can focus on building robust, scalable, and maintainable software applications that efficiently interact with databases.