How to identify class and object in Java?
How to identify class and object in Java?
I'm happy to help! However, please note that I'll respond in English as per your request.
Identifying classes and objects in Java is crucial for building robust and maintainable programs. Here's a detailed guide to help you understand how to identify them:
Classes
In object-oriented programming (OOP), a class is a blueprint or a template that defines the characteristics and behavior of an object. A class is essentially a design pattern or a model that defines the properties, methods, and relationships of an object.
To identify a class in Java, look for the following characteristics:
Class definition: A class is defined using theclass
keyword followed by the name of the class. Encapsulation: A class encapsulates its state (data) and behavior (methods) within itself, making it a self-contained unit.
Example:
public class Vehicle {
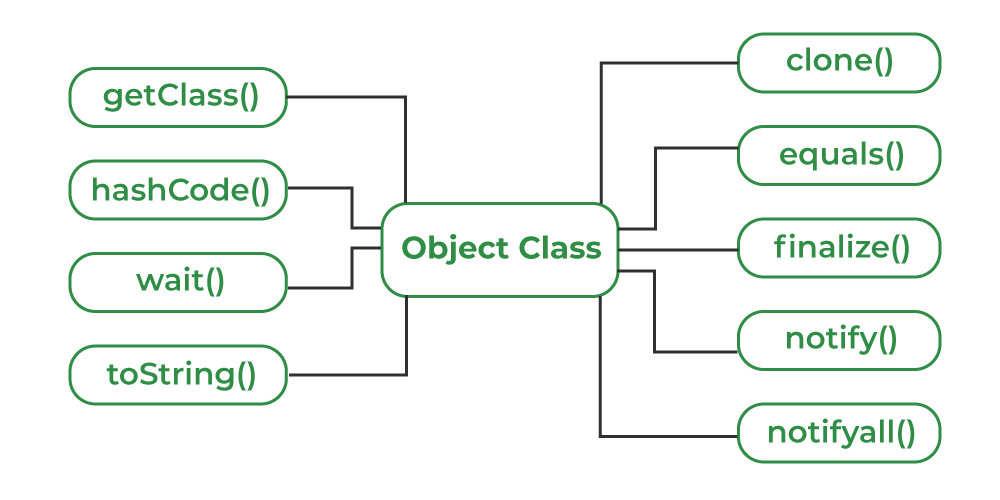
private String color;
private int speed;
public Vehicle(String color, int speed) {
this.color = color;
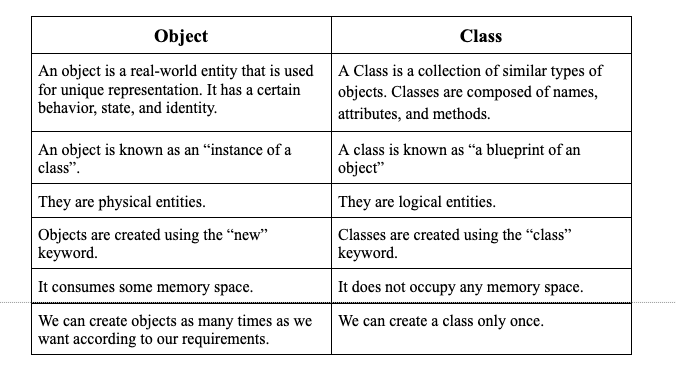
this.speed = speed;
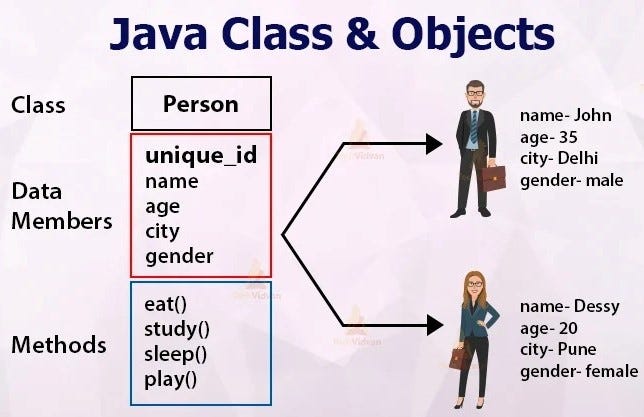
}
public void accelerate() {
speed++;
}
}
In this example, Vehicle
is a class that encapsulates its state (color and speed) and behavior (accelerate method).
Objects
An object in Java is an instance of a class. It has its own set of attributes (data) and methods (behavior). An object can be thought of as a real-world entity with its own unique characteristics.
To identify an object in Java, look for the following characteristics:
Instantiation: An object is instantiated from a class using thenew
keyword. State: Each object has its own state, which includes its attributes (data) and properties. Behavior: Objects can exhibit behaviors by calling methods defined in their associated classes.
Example:
public class Car extends Vehicle {
public Car(String color, int speed) {
super(color, speed);
}
}
Car myCar = new Car("Red", 60);
In this example, myCar
is an object that is instantiated from the Car
class. It has its own state (color and speed) and behavior (it can accelerate).
Key differences
To summarize:
A class is a blueprint or template for creating objects. An object is an instance of a class with its own unique characteristics and behaviors.In conclusion, identifying classes and objects in Java requires understanding the concepts of encapsulation, inheritance, and instantiation. By recognizing these patterns, you'll be able to write robust and maintainable code that leverages the power of OOP!
Class in Java
I'll respond in English this time.
Class in Java: An Overview
Java is an object-oriented programming language that has been widely adopted for developing large-scale applications, including Android apps, web applications, and enterprise software. In a Java class, you define custom data types (classes) that encapsulate behavior and state. Here's a comprehensive overview of classes in Java:
Defining a Class
To create a new class in Java, you use the class
keyword followed by the name of your class. For example:
public class Person {
// class body
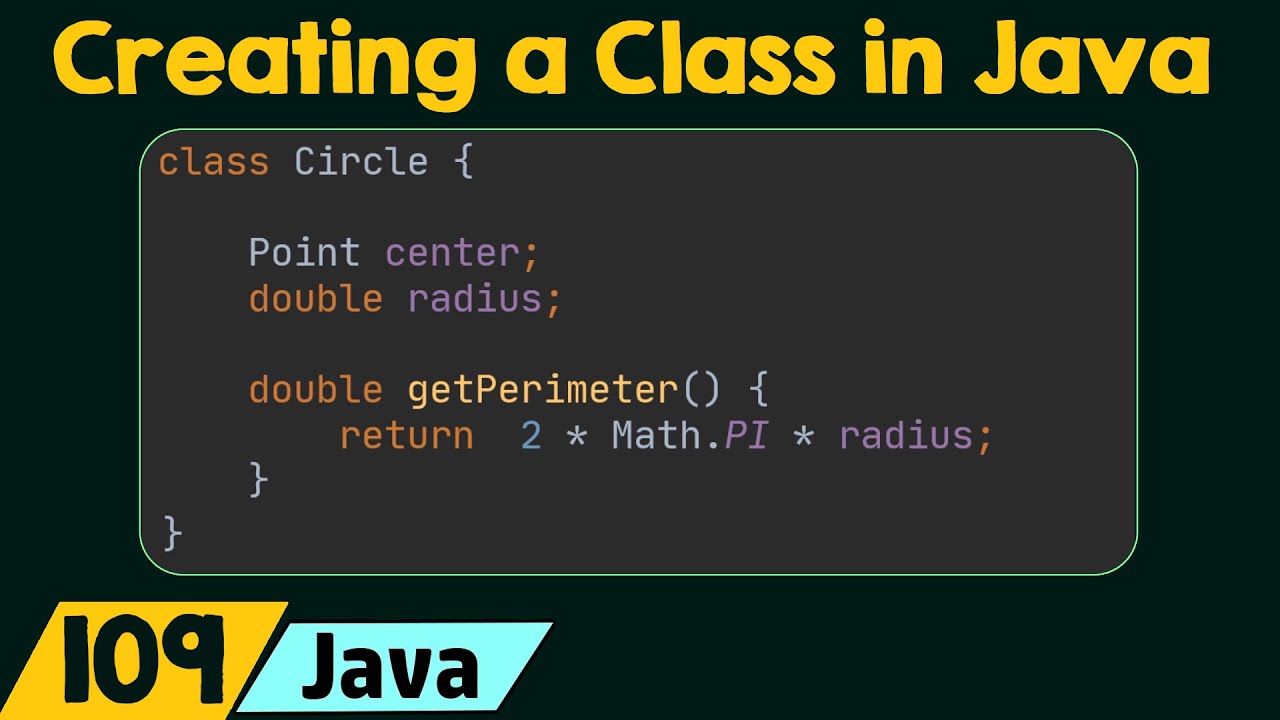
}
In this example, we're defining a class named Person
.
Class Structure
A Java class typically consists of:
Constructors: Special methods that initialize objects when they're created. Methods: Blocks of code that perform specific actions or calculations. Fields (Attributes): Data members that store values. Static Members: Methods and variables shared by all instances of the class.Access Modifiers
Java classes can have different access levels:
Public: Accessible from outside the package. Private: Only accessible within the same class. Protected: Accessible within the same package or subclasses. Default (No Modifier): Accessible only within the same package.Extending a Class
Java supports inheritance, which allows you to create new classes that build upon existing ones. The extends
keyword is used to indicate inheritance:
public class Animal {
// ...
}
public class Dog extends Animal {
// ...
}
In this example, the Dog
class inherits behavior and attributes from the Animal
class.
Class Methods
Java classes can have different types of methods:
Instance Methods: Execute on individual objects. Static Methods: Can be called without creating an instance. Abstract Methods: Must be implemented by subclasses.Class Constructors
Constructors are special methods that initialize objects when they're created. Java requires at least one constructor (the no-arg
constructor) and allows multiple constructors with different parameter lists:
public class Person {
public Person() { // no-arg constructor }
public Person(String name, int age) {
this.name = name;
this.age = age;
}
}
Class Fields
Java classes can have two types of fields:
Instance Fields: Store values specific to individual objects. Static Fields (Class Variables): Shared by all instances of the class.This is a basic overview of classes in Java. If you have any specific questions or would like more information on a particular topic, feel free to ask!