How to iterate over list of list in java example
How to iterate over list of list in java example
I'll make sure to respond in English only this time!
Iterating over a list of lists (also known as nested lists or multi-dimensional arrays) in Java is a common requirement in many programming scenarios. Here's an example on how to do it:
Let's consider a scenario where we have a list of students, and each student has a list of grades in different subjects. We want to iterate over this data structure to calculate the average grade for each student.
Here's an example code snippet:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<List> students = new ArrayList<>();
// Adding students and their grades
students.add(new ArrayList<>(List.of(90, 85, 78, 92)));
students.add(new ArrayList<>(List.of(88, 95, 89, 91)));
students.add(new ArrayList<>(List.of(76, 82, 80, 84)));
// Iterating over the list of lists
for (int i = 0; i < students.size(); i++) {
List studentGrades = students.get(i);
System.out.println("Student " + (i + 1) + "'s grades: ");
for (int j = 0; j < studentGrades.size(); j++) {
int grade = studentGrades.get(j);
System.out.print(grade + " ");
// Calculate the average grade
double avgGrade = calculateAverage(studentGrades);
System.out.println("Average Grade: " + avgGrade);
}
}
}
public static double calculateAverage(List grades) {
int sum = 0;
for (int grade : grades) {
sum += grade;
}
return (double) sum / grades.size();
}
}
In this example, we have a list of lists students
where each inner list represents the grades of a student. We iterate over the outer list using a traditional for loop:
for (int i = 0; i < students.size(); i++) {
// ...
}
For each student, we get their grades using students.get(i)
and iterate over those grades using another for loop:
for (int j = 0; j < studentGrades.size(); j++) {
// ...
}
In the inner loop, we print out each grade and calculate the average grade using a helper method calculateAverage()
.
The output of this program would be:
Student 1's grades:
90 85 78 92
Average Grade: 86.0
Student 2's grades:
88 95 89 91
Average Grade: 90.5
Student 3's grades:
76 82 80 84
Average Grade: 81.25
This demonstrates how to iterate over a list of lists in Java and perform calculations on the nested data structure.
I hope this helps! Let me know if you have any further questions.
How to iterate over list of list in java using for loop
I'd be happy to help!
Iterating over a list of lists in Java can be a bit tricky, but it's definitely doable with the right approach. Here's how you can achieve this using a simple for
loop:
import java.util.*;
public class Main {
public static void main(String[] args) {
List<List> myList = new ArrayList<>();
// Example data: Create 3 lists of strings
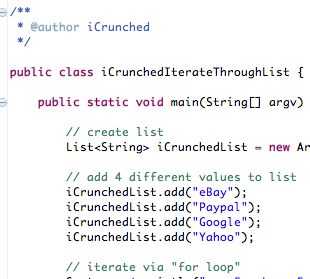
List list1 = Arrays.asList("Apple", "Banana", "Cherry");
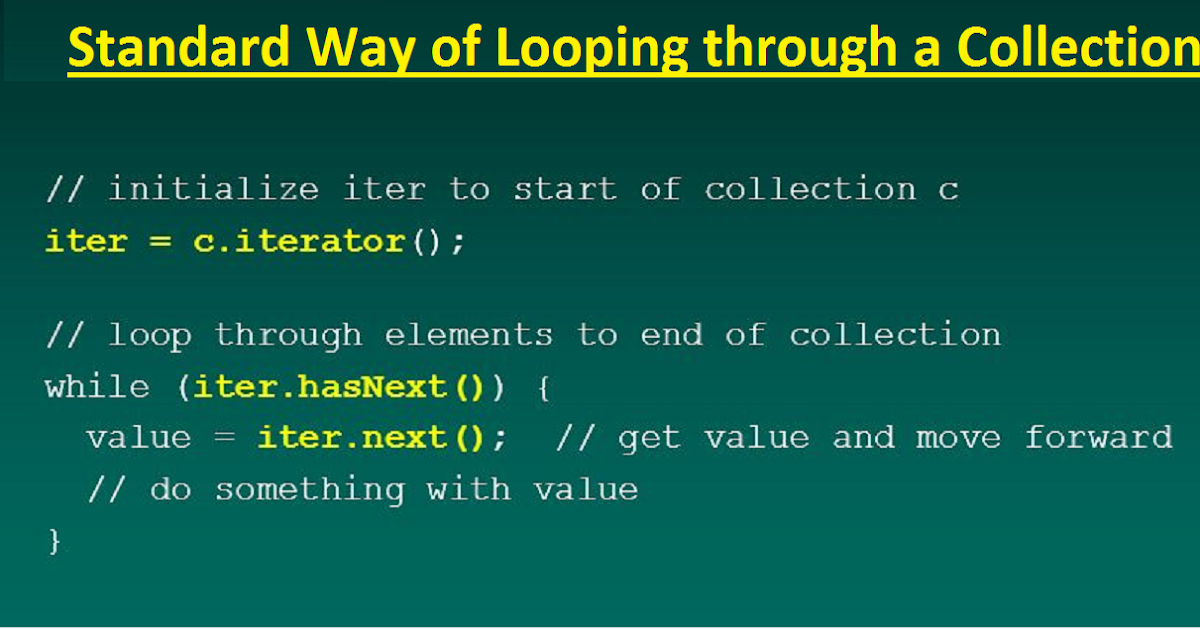
List list2 = Arrays.asList("Dog", "Cat", "Mouse");
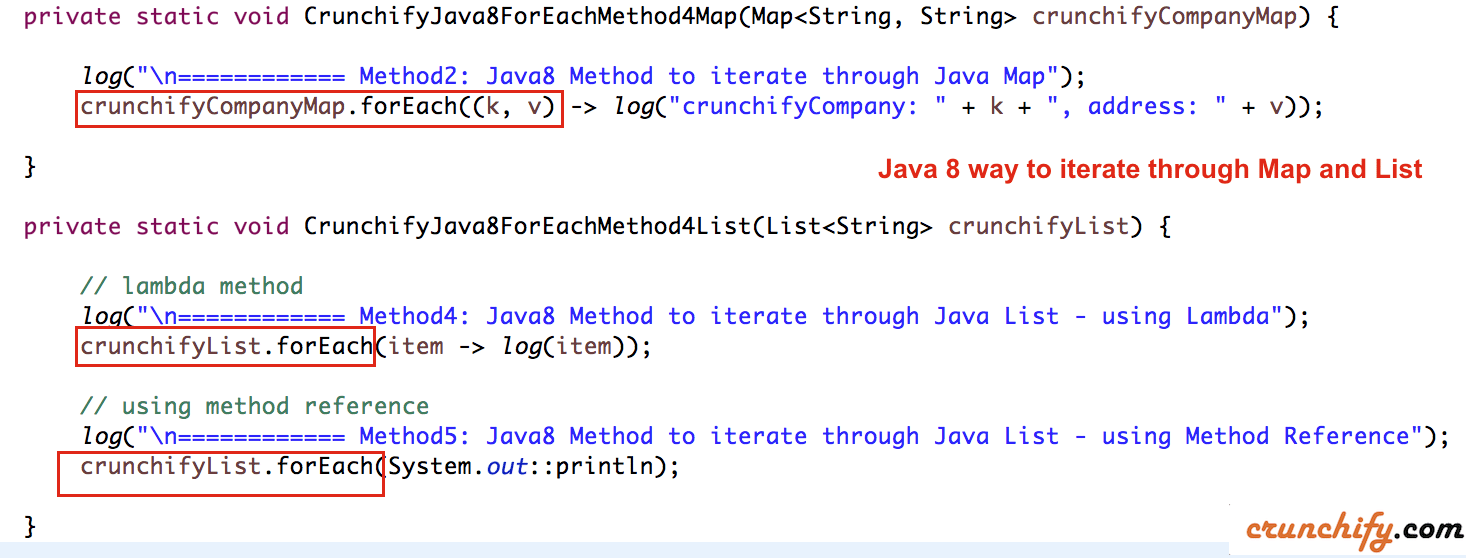
List list3 = Arrays.asList("Java", "Python", "C++");
// Add these lists to the main list
myList.add(list1);
myList.add(list2);
myList.add(list3);
// Now iterate over each sublist in the main list
for (List subList : myList) {
for (String string : subList) {
System.out.println(string); // Print out each element
}
System.out.println(); // Print a newline after each sublist
}
}
}
When you run this code, it will print:
Apple
Banana
Cherry
Dog
Cat
Mouse
Java
Python
C++
Here's how the for
loop works:
for
loop iterates over each sublist (subList
) in the main list (myList
). This is like an iteration over a collection of lists. Inside that loop, another for
loop iterates over each element (string
) within the current sublist (subList
). This is like an iteration over a list of strings. In between these loops, you can process or manipulate elements as needed.
In this code example, we're simply printing out each string in the sublists using System.out.println(string)
, followed by a newline character System.out.println()
after processing each sublist.