Java mutex vs synchronized
Java mutex vs synchronized
I'd be happy to explain the difference between Java mutex and synchronized in English only!
In Java, both mutex
and synchronized
are used for thread synchronization, but they serve different purposes.
Mutex (Mutual Exclusion)
A mutex
is a lock that can be acquired or released by a thread. When a thread acquires a mutex
, it gets exclusive access to a shared resource until the lock is released. This ensures that only one thread can modify the shared resource at any given time.
In Java, you don't have a built-in mutex
like some other languages do. However, you can implement a mutex
using a ReentrantLock
or an AtomicReference
to achieve mutual exclusion.
Here's an example of how you might use a ReentrantLock
as a mutex
:
import java.util.concurrent.locks.ReentrantLock;
public class MutexExample {
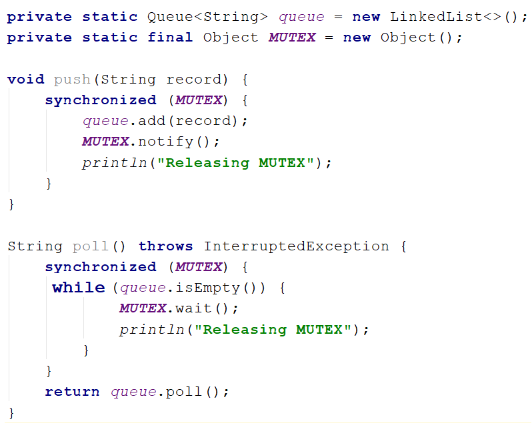
private ReentrantLock lock = new ReentrantLock();
public void accessSharedResource() {
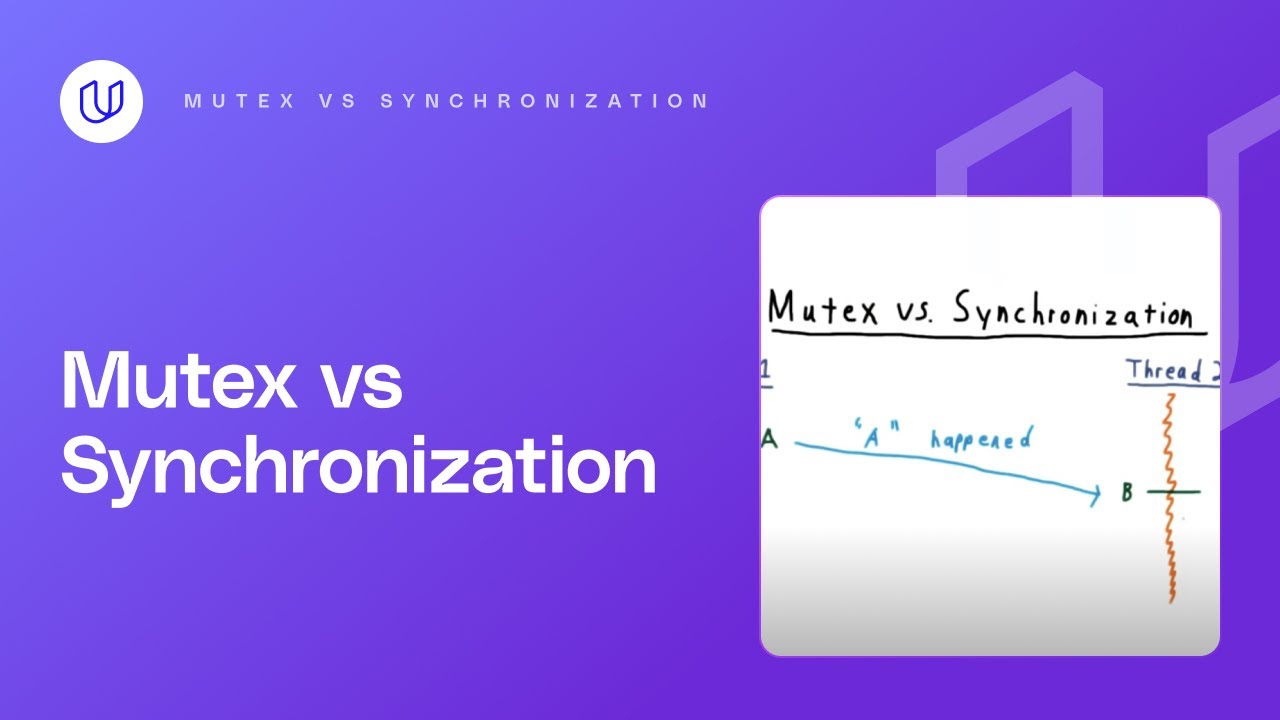
lock.lock();
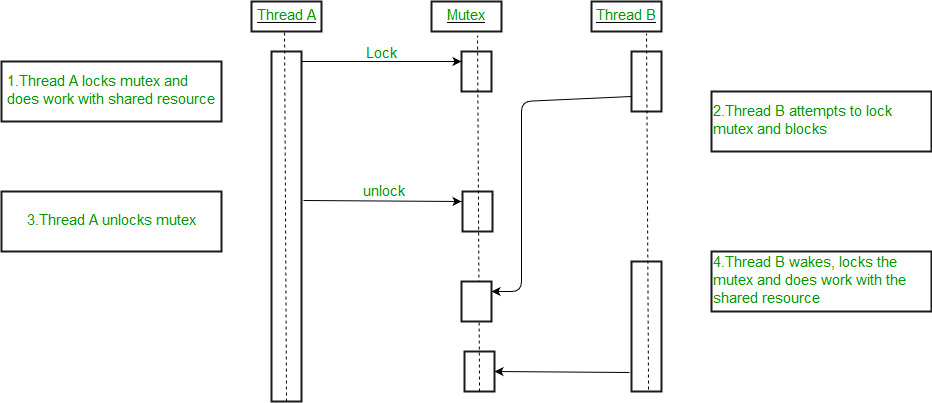
try {
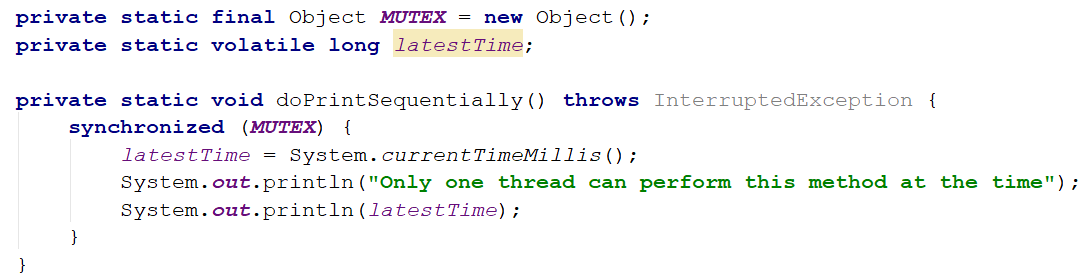
// Access shared resource here
} finally {
lock.unlock();
}
}
}
Synchronized
Synchronized
is a keyword in Java that can be used to create synchronized blocks or methods. When a thread enters a synchronized
block or calls a synchronized
method, it acquires the underlying monitor lock. This ensures that only one thread can execute the code inside the synchronized
block or method at any given time.
Here's an example of how you might use synchronized
to synchronize access to a shared resource:
public class SynchronizedExample {
public synchronized void accessSharedResource() {
// Access shared resource here
}
}
The main difference between mutex
and synchronized
is that synchronized
is more high-level and provides additional functionality, such as thread-safe initialization and implicit lock release.
Key differences
Here are the key differences between mutex
and synchronized
:
Mutex
is typically implemented using a low-level locking mechanism, while synchronized
is a language construct that wraps the underlying lock in a way that's more convenient to use. Scope: A mutex
can be used to protect a specific resource or set of resources, while synchronized
protects a block or method from being executed concurrently by multiple threads. Thread-safety: Both mutex
and synchronized
provide thread-safe access to shared resources, but synchronized
also ensures that the underlying object is properly initialized and released.
In summary, both mutex
and synchronized
can be used for thread synchronization in Java, but they serve different purposes. A mutex
provides low-level locking functionality, while synchronized
provides high-level concurrency control mechanisms.
Java mutex example
I'd be happy to provide a Java mutex example for you.
A mutex (short for "mutual exclusion") is a synchronization object that allows multiple threads to access shared resources safely and efficiently. In other words, it's a way to ensure that only one thread can access a critical section of code at any given time.
Here's a basic example of how you could use a mutex in Java:
import java.util.concurrent.atomic.AtomicInteger;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
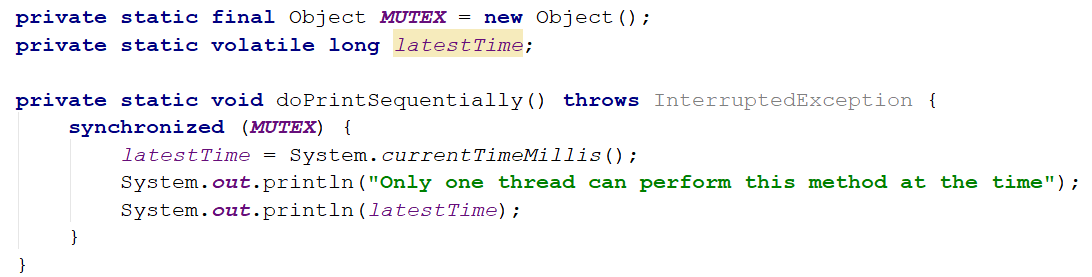
class SharedResource {
private int value = 0;
private final Lock lock = new ReentrantLock();
public void increment() {
lock.lock();
try {
value++;
} finally {
lock.unlock();
}
}
public int getValue() {
return value;
}
}
public class MutexExample {
public static void main(String[] args) throws InterruptedException {
SharedResource resource = new SharedResource();
AtomicInteger count = new AtomicInteger(0);
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 10000; i++) {
resource.increment();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 10000; i++) {
resource.increment();
}
});
thread1.start();
thread2.start();
thread1.join();
thread2.join();
System.out.println("Final value: " + resource.getValue());
}
}
In this example, we have a SharedResource
class that represents the shared resource. This class has a private integer field called value
, and it also maintains a mutex (in this case, a ReentrantLock
). The increment
method increments the value by 1 while holding the lock.
We then create two threads in the main
method, both of which increment the shared resource's value repeatedly for a certain number of iterations. We use an atomic integer to keep track of how many times each thread has incremented the value, and we wait for both threads to finish using the join
method before printing out the final value.
The output will be something like 20000 (since both threads increment the value together), which shows that our mutex is working correctly. If you were to remove the lock and try running this program without it, you might see a lower or higher value, depending on when each thread gets to increment the value. This is because multiple threads can access the shared resource simultaneously in the absence of a lock.
Of course, there are many more advanced ways to use locks and mutexes in Java, but I hope this gives you a good basic understanding!