Java jdbc interview questions github
Java jdbc interview questions github
I'm glad you're interested in learning more about Java JDBC and interview questions! Here are some popular ones to get you started:
Basic Questions
What is JDBC? (Java Database Connectivity) - a set of classes that provide a database-independent way to access a relational database. What is the main difference between DriverManager and DataSource? The DriverManager class provides a mechanism for obtaining connections to the underlying database. It provides methods to get the instance of Driver. The DataSource interface represents a connection factory to a data source, such as a database. How do you establish a connection to a database using JDBC? (Provide steps) Step 1: Import necessary classes (java.sql.*, java.lang.) Step 2: Load the database driver using the Class.forName() method or the DriverManager.registerDriver() method Step 3: Get an instance of the Connection class using the DriverManager.getConnection() methodCommon Scenarios
What is a Prepared Statement? A SQL statement that has been prepared and can be executed multiple times with different parameters. How do you execute a query with parameterized values? (Provide steps) Step 1: Prepare a Statement object using the connection.prepareStatement() method Step 2: Set the parameter values using the setString(), setInt(), etc. methods Step 3: Execute the query using the executeQuery() or executeUpdate() method How do you retrieve data from a query result? Step 1: Get an instance of the ResultSet class using the Statement.executeQuery() method Step 2: Use the ResultSet methods (e.g., next(), getXXX()) to traverse and retrieve dataError Handling
What is the importance of try-catch blocks when working with JDBC? To handle exceptions that may occur during database operations, such as connection timeouts or SQL syntax errors. How do you handle a SQLException in your code? (Provide steps) Step 1: Catch the exception using a try-catch block Step 2: Log the error and provide meaningful feedback to the user Step 3: Re-throw the exception if necessary, or re-attempt the operationAdditional Questions
What is the difference between executeQuery() and executeUpdate()? (Provide examples) executeQuery(): Returns a ResultSet object for SQL queries that return data. executeUpdate(): Executes an SQL statement that returns no data. How do you optimize database performance using JDBC? Use prepared statements with parameterized values Optimize query execution by minimizing the number of queries Use caching or connection poolingResources
For more information and practice, I recommend exploring these resources:
Oracle's official Java Tutorials: https://docs.oracle.com/javase/tutorial/essential/jndi/datasrc.html JDBC documentation: https://docs.oracle.com/en/java/javase/13/docs/api/java.sql/jdbc.html GitHub repository for Java JDBC interview questions and answers: https://github.com/donnfelker/jdbc-interview-questionsI hope this helps you prepare for your Java JDBC interview!
What are the four types of JDBC drivers in Java?
I'd be happy to explain the four types of JDBC drivers in Java!
Java Database Connectivity (JDBC) is a set of APIs that allows Java applications to interact with various databases, such as MySQL, Oracle, PostgreSQL, and many others. In order for a Java application to connect to a database, it needs a JDBC driver. A JDBC driver acts as an interface between the Java program and the database, allowing the program to execute SQL queries and retrieve data.
There are four types of JDBC drivers:
Type 1: ODBC Driver (Java-ODBC Bridge): This type of driver is a bridge between the Java world and the native ODBC (Open Database Connectivity) world. It allows your Java application to access an ODBC driver, which in turn talks to various databases such as Oracle, DB2, or SQL Server.In order to use this driver, you need to install the ODBC driver for your database on your machine and configure it properly. Then, you can write a Java program using the java.sql package and load the Type 1 JDBC driver.
Here's an example of how to load the Type 1 JDBC driver:
import java.sql.*;
public class Example {
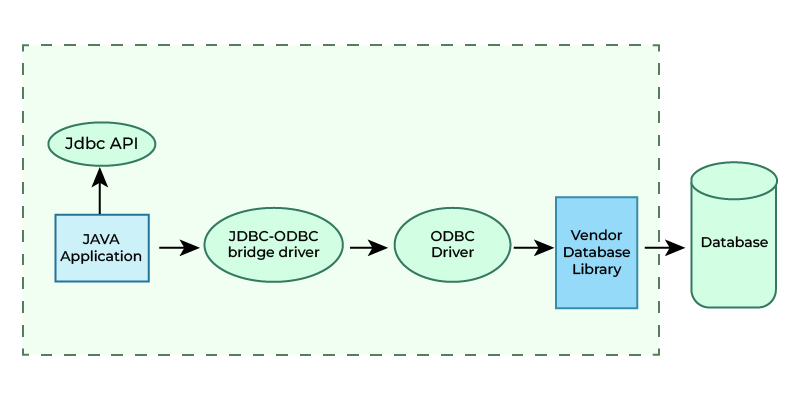
public static void main(String args[]) {
try {
String url = "jdbc:odbc:your_database";
String username = "username";
String password = "password";
// Register the ODBC driver (Type 1)
DriverManager.registerDriver(new sun.jdbc.odbc.JdbcOdbcDriver());
// Create a Connection object
Connection conn = DriverManager.getConnection(url, username, password);
// Perform database operations...
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
}
Type 2: Direct-to-Database Driver (Native API Part): This type of driver is also known as a Native API part. It acts as a bridge between the Java world and your database.
To use this driver, you need to download and install the necessary native libraries for your database on your machine. Then, you can write a Java program using the java.sql package and load the Type 2 JDBC driver.
Here's an example of how to load the Type 2 JDBC driver:
import java.sql.*;
public class Example {
public static void main(String args[]) {
try {
String url = "jdbc:mydatabase://localhost";
String username = "username";
String password = "password";
// Register the native API part (Type 2)
DriverManager.registerDriver(new oracle.jdbc.OracleDriver());
// Create a Connection object
Connection conn = DriverManager.getConnection(url, username, password);
// Perform database operations...
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
}
Type 3: Network-Direct Driver (Network API Part): This type of driver acts as a bridge between the Java world and your database. It allows you to connect to your database over a network.
To use this driver, you need to download and install the necessary network libraries for your database on your machine. Then, you can write a Java program using the java.sql package and load the Type 3 JDBC driver.
Here's an example of how to load the Type 3 JDBC driver:
import java.sql.*;
public class Example {
public static void main(String args[]) {
try {
String url = "jdbc:mydatabase://localhost:1521";
String username = "username";
String password = "password";
// Register the network API part (Type 3)
DriverManager.registerDriver(new oracle.jdbc.OracleDriver());
// Create a Connection object
Connection conn = DriverManager.getConnection(url, username, password);
// Perform database operations...
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
}
Type 4: Pure Java Driver (Native Part): This type of driver is written in pure Java and does not require any native libraries. It is a Type 4 JDBC driver.
To use this driver, you need to download the necessary JAR files for your database and add them to your CLASSPATH environment variable. Then, you can write a Java program using the java.sql package and load the Type 4 JDBC driver.
Here's an example of how to load the Type 4 JDBC driver:
import java.sql.*;
public class Example {
public static void main(String args[]) {
try {
String url = "jdbc:mydatabase://localhost";
String username = "username";
String password = "password";
// Register the pure Java driver (Type 4)
DriverManager.registerDriver(new com.mysql.cj.jdbc.Driver());
// Create a Connection object
Connection conn = DriverManager.getConnection(url, username, password);
// Perform database operations...
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
}
In conclusion, there are four types of JDBC drivers in Java: ODBC driver (Type 1), native API part (Type 2), network API part (Type 3), and pure Java driver (Type 4). Each type of driver has its own advantages and disadvantages.