How to ignore checked exception in Java?
How to ignore checked exception in Java?
I'd be happy to help you with that!
In Java, a checked exception is an exception that the compiler checks for and requires you to handle or propagate somehow. You can think of it as a warning from the compiler saying, "Hey, I know there's a chance something might go wrong here, so please take care of this!" When you're writing code, it's generally a good idea to listen to the compiler and deal with exceptions properly.
Now, if you want to ignore a checked exception in Java, there are a few ways to do it:
Catch and rethrow: You can catch the exception and then immediately rethrow it using thethrow
statement. This is useful when you want to provide some custom error handling or logging before allowing the original exception to propagate up the call stack.
Example:
try {
// code that might throw an exception
} catch (Exception e) {
// handle the exception in some way
throw; // rethrow the original exception
}
Catch and return: You can catch the exception and then immediately return from the method or function, allowing the exception to propagate up the call stack. This is useful when you don't care about handling the exception yourself, but rather want to let someone else deal with it.
Example:
try {
// code that might throw an exception
} catch (Exception e) {
return; // return from this method
}
Use a try-with-resources statement: If you're using Java 7 or later, you can use a try-with-resources statement with a resource that throws a checked exception. In this case, you don't need to catch the exception explicitly, because the compiler knows that it will be handled automatically.
Example:
try (Resource res = new Resource()) {
// code that might throw an exception
} // resource is closed automatically if an exception is thrown
Use a wrapper class: You can create a wrapper class around some other code that throws checked exceptions, and then ignore the exceptions within the wrapper class. This can be useful when you need to perform some custom error handling or logging before allowing the original exception to propagate up the call stack.
Example:
public class Wrapper {
public void doSomething() {
try {
// code that might throw an exception
} catch (Exception e) {
// handle the exception in some way
}
}
}
Remember, when you're ignoring checked exceptions in Java, it's important to carefully consider whether this is really what you want to do. Ignoring exceptions can make your code more brittle and harder to debug, so use these techniques sparingly and only when you have a good reason for doing so!
Hope that helps! Let me know if you have any questions or need further clarification.
What is checked exception in Java with example?
I'm happy to help you!
In Java, a checked exception is an exceptional condition that must be explicitly handled or declared to be thrown by the method. A checked exception is a subclass of the Exception
class and must be caught using try-catch
block or declared in the method signature.
Here's an example:
import java.io.FileNotFoundException;
public class Example {
public void checkFile(String filename) throws FileNotFoundException {
// Code that checks if the file exists
if (!new File(filename).exists()) {
throw new FileNotFoundException("The file " + filename + " does not exist.");
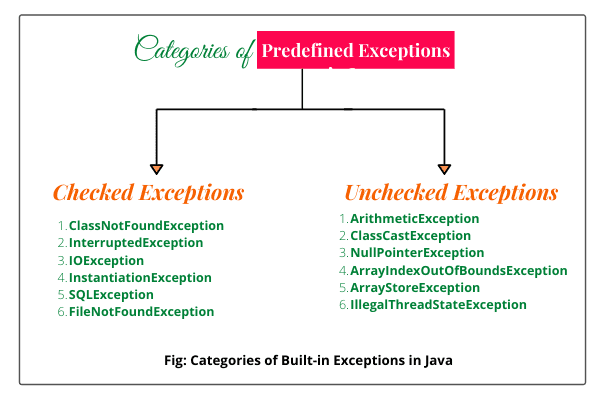
}
}
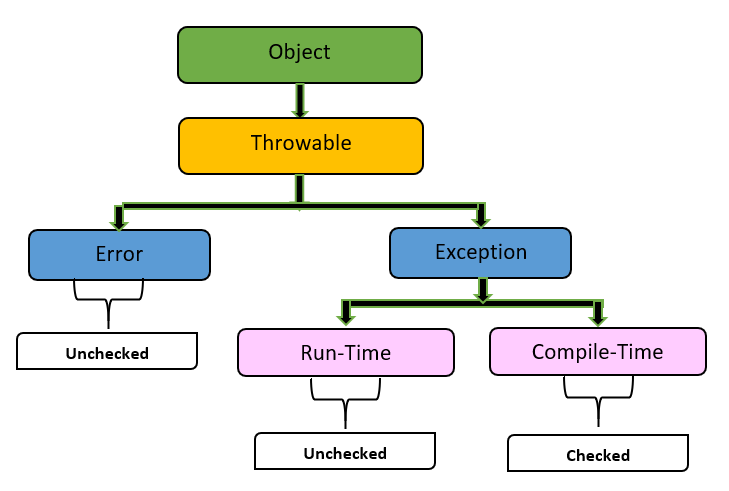
}
In this example, the checkFile
method takes a filename as an argument and checks if the file exists. If the file does not exist, it throws a FileNotFoundException
. Because FileNotFoundException
is a checked exception, we must either catch it using try-catch
block or declare that it may be thrown by the method.
Here's how you can handle this exception:
public class Example {
public void checkFile(String filename) throws FileNotFoundException {
try {
// Code that checks if the file exists
if (!new File(filename).exists()) {
throw new FileNotFoundException("The file " + filename + " does not exist.");
}
} catch (FileNotFoundException e) {
System.out.println("Error: " + e.getMessage());
}
}
public static void main(String[] args) {
Example example = new Example();
try {
example.checkFile("non-existent-file.txt");
} catch (FileNotFoundException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
In the main
method, we call the checkFile
method with a non-existent file. The FileNotFoundException
is thrown, caught by the catch
block, and an error message is printed.
Here are some key points to remember:
A checked exception must be explicitly handled or declared. You can handle a checked exception usingtry-catch
block or declare it in the method signature. Checked exceptions are subclasses of the Exception
class. You cannot catch an unchecked exception (a subclass of RuntimeException
) using a try-catch
block.
In summary, checked exceptions are conditions that must be explicitly handled or declared in Java. They provide a way to handle exceptional situations and ensure that your code is robust and reliable.