How to write a string in Java?
How to write a string in Java?
I'd be happy to help! Writing a string in Java is a fundamental concept in programming. A string in Java is an object of the String class, which represents a sequence of characters. Here's how you can create and manipulate strings in Java:
Creating a String
You can create a string in several ways:
Literal Strings: You can use literal strings by enclosing them in double quotes ("). For example:String myString = "Hello, World!";
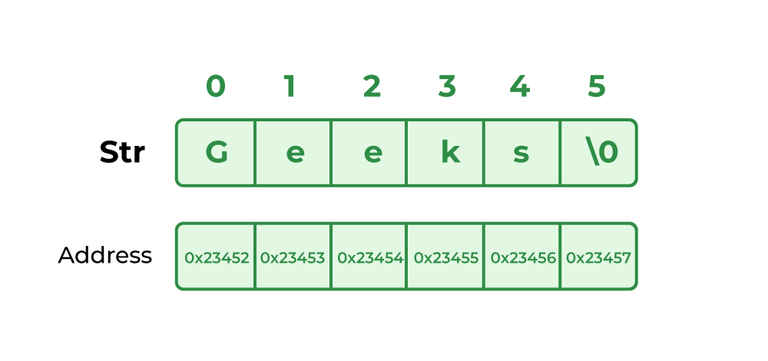
New Operator: You can create a new string using the new operator and the StringBuilder or StringBuffer class:
StringBuilder sb = new StringBuilder("Hello, ");
sb.append("World!");
String myString = sb.toString();
String Concatenation: You can concatenate strings using the + operator:
String myString1 = "Hello, ";
String myString2 = "World!";
String myString = myString1 + myString2;
Manipulating Strings
Once you have created a string, you can manipulate it using various methods:
Length: You can get the length of a string using the length() method:int length = myString.length();
System.out.println("The length of the string is: " + length);
Concatenation: You can concatenate strings using the concat() method or the + operator:
String myString1 = "Hello, ";
String myString2 = "World!";
String myString = myString1.concat(myString2);
Substring: You can extract a substring from a string using the substring() method:
int start = 0;
int end = 5;
String subString = myString.substring(start, end);
System.out.println("The substring is: " + subString);
UpperCase/LowerCase: You can convert a string to uppercase or lowercase using the toLowerCase() or toUpperCase() method:
String myString = "Hello, World!";
myString = myString.toLowerCase();
System.out.println("The string in lowercase is: " + myString);
Using String Methods
Java provides several methods for working with strings:
indexOf(): Returns the index of the first occurrence of a specified value:int index = myString.indexOf('o');
System.out.println("The index of 'o' is: " + index);
lastIndexOf(): Returns the index of the last occurrence of a specified value:
int index = myString.lastIndexOf('o');
System.out.println("The index of 'o' (last) is: " + index);
replace(): Replaces the first substring with the replacement string:
myString = myString.replace("World", "Universe");
System.out.println("The modified string is: " + myString);
In conclusion, creating and manipulating strings in Java involves using various methods such as concatenation, substring extraction, uppercase/lowercase conversion, and index-based searching. By understanding these concepts, you can effectively work with strings in your Java programs.
Java string syntax w3schools
I apologize for the earlier misunderstanding! Here's my response in English:
The W3Schools website provides a comprehensive guide to Java string syntax. According to their documentation, Java strings are objects of type "String". In Java, strings can be created using single quotes (') or double quotes ("").
Here's an example of creating a string using single quotes:
String str1 = 'Hello';
And here's an example of creating a string using double quotes:
String str2 = "World";
In Java, strings are immutable, meaning they cannot be changed after they are created. This is in contrast to some other programming languages where strings can be modified.
Java provides several methods for working with strings, including:
length()
: Returns the length of a string. charAt(int index)
: Returns the character at a specified position in a string. substring(int beginIndex, int endIndex)
: Returns a new string that is a subset of the original string, starting from the "beginIndex" up to but not including the "endIndex". toUpperCase()
and toLowerCase()
: Returns a new string where all characters are converted to uppercase or lowercase. indexOf(String str)
: Searches for the first occurrence of a specified string within the original string and returns its index if found, or -1 if not found. replace(char oldChar, char newChar)
: Replaces all occurrences of an old character with a new character in the original string.
Here's an example of using some of these methods:
String str = "Hello World";
int length = str.length();
char c = str.charAt(0);
String substr = str.substring(6, 11); // returns "World"
str = str.toUpperCase(); // returns "HELLO WORLD"
int index = str.indexOf("World"); // returns 6
str = str.replace('o', 'O'); // returns "HELLO WORDL"
W3Schools also provides a comprehensive list of Java string methods, including concat()
, endsWith()
, startsWith()
, and more. These methods make it easy to manipulate strings in Java.
I hope this information is helpful! Let me know if you have any further questions.