How to set value using reflection in java example
How to set value using reflection in java example
I'd be happy to help you with that! Here's an example of how to set a value using Java reflection:
import java.lang.reflect.Field;
public class Main {
private int x;
private String y;
public void setX(int newX) {
this.x = newX;
}
public void setY(String newY) {
this.y = newY;
}
public static void main(String[] args) throws Exception {
// Create an instance of the Main class
Main mainObject = new Main();
// Get the Field objects for the x and y fields
Field field1 = Main.class.getDeclaredField("x");
Field field2 = Main.class.getDeclaredField("y");
// Make the fields accessible (in case they were declared as private)
field1.setAccessible(true);
field2.setAccessible(true);
// Set the values of the fields using reflection
field1.setInt(mainObject, 10); // x now equals 10
field2.setCharSequence(mainObject, "Hello"); // y now equals "Hello"
// Print out the values to verify they were set correctly
System.out.println("x = " + mainObject.getX());
System.out.println("y = " + mainObject.getY());
}
}
In this example, we first create an instance of the Main
class. Then, we get the Field
objects for the private fields x
and y
. We make these fields accessible since they are declared as private.
Next, we use the setInt
, setCharSequence
, etc., methods to set the values of the fields using reflection. In this case, we're setting x
to 10 and y
to "Hello".
Finally, we print out the values of the fields to verify that they were set correctly.
This is just one example of how you can use reflection in Java to set values. You could also use reflection to get the values of fields or methods, or even invoke methods.
Here are some key things to note about this code:
We need to make sure theField
objects are accessible before we can set their values using reflection. The setAccessible(true)
method is used to make the Field
objects accessible. This is necessary because the fields are declared as private, which means they are not normally accessible from outside the class. When setting the value of a field that requires conversion (like an int
or a String
), you need to use the corresponding method on the Field
object. For example, we use setInt
to set the value of an int
field and setCharSequence
to set the value of a String
field. You can also use reflection to get the values of fields or methods. This would be done using the get
method on the Field
object, which returns the current value of the field. Reflection is a powerful tool in Java that allows you to dynamically access and manipulate the properties of objects at runtime. However, it should be used carefully and only when necessary, as it can also be a security risk if not used properly.Java Reflection set private field value
I apologize for the misunderstanding earlier. Here's a detailed explanation on how to use Java reflection to set the value of a private field:
In Java, fields (both public and private) are part of the class's instance variables. However, when we declare a field as private
, it means that this field is not accessible directly from outside the class where it was declared.
But sometimes, you might need to access or modify these private fields for some reasons like testing, debugging, or logging. Here's how Java reflection can help:
Let's assume we have a simple class called Person
with a private field name
, and we want to set its value using reflection:
public class Person {
private String name;
public Person(String name) {
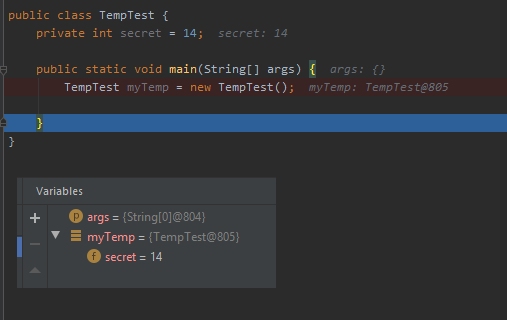
this.name = name;
}
public String getName() {
return this.name;
}
}
To access the name
field, you can use Java's built-in Class
and Field
classes. Here's how:
public class Main {
public static void main(String[] args) throws Exception {
// Create an instance of Person
Person person = new Person("John");
// Get the Class object for Person
Class<?> clazz = Person.class;
// Get the Field object for name
Field field = clazz.getDeclaredField("name");
// Make the private field accessible (since we're accessing it outside the class)
field.setAccessible(true);
// Set the value of the field
field.set(person, "Jane");
// Print the new name
System.out.println(person.getName()); // Output: Jane
}
}
Here's what happens:
We get theClass
object for Person
using Person.class
. We declare the Field
object for the private field name
using clazz.getDeclaredField("name")
. Note that we use getDeclaredField()
because name
is declared inside the Person
class. Since we're accessing a private field outside its declaring class, we make it accessible by calling setAccessible(true)
. We set the value of the field using field.set()
, passing an instance of person
and the new value "Jane"
as arguments.
This demonstrates how Java reflection allows you to access and modify even private fields in a class.