Java template parameter
Java template parameter
I'd be happy to respond in English!
Java is an object-oriented programming language that supports generic programming through its type system. Template parameters are a fundamental concept in Java's type system, allowing developers to define reusable classes and methods with varying types.
In Java, template parameters are denoted by the <
and >
characters. For example:
public class Container {
private T value;
public void setValue(T value) {
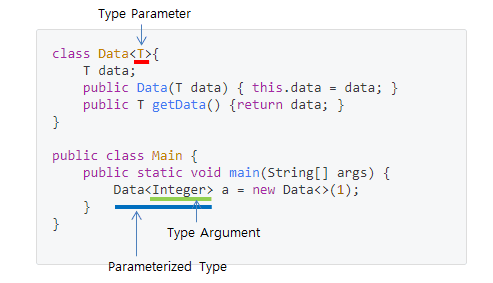
this.value = value;
}
public T getValue() {
return this.value;
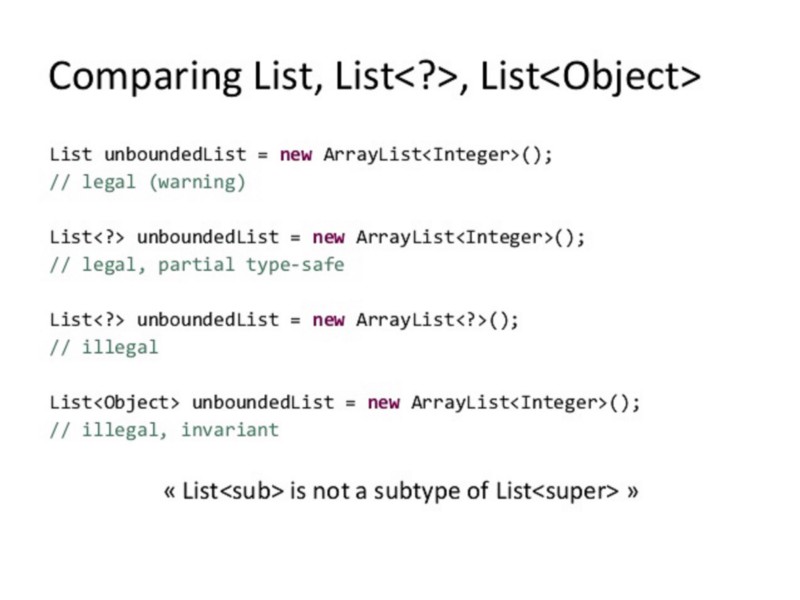
}
}
In the above code, T
is a template parameter that represents a type. The Container
class can be instantiated with any type, such as Integer
, String
, or even another custom class.
public static void main(String[] args) {
Container intContainer = new Container<>();
intContainer.setValue(10);
Integer value = intContainer.getValue();
System.out.println("Value: " + value);
Container strContainer = new Container<>();
strContainer.setValue("Hello");
String strValue = strContainer.getValue();
System.out.println("Value: " + strValue);
}
In the main
method, we create two instances of the Container
class: one for storing an integer and another for storing a string. The setValue
and getValue
methods demonstrate how the container can store and retrieve values of different types.
Template parameters in Java have several benefits:
Code reusability: By using template parameters, you can define classes and methods that can work with different types, reducing code duplication. Type safety: The compiler checks the type compatibility when instantiating a class or calling a method with template parameters, helping to prevent runtime errors. Flexibility: Template parameters allow developers to write generic code that can be applied to various scenarios, making their programs more adaptable and maintainable.In summary, Java's template parameter system enables developers to define reusable classes and methods with varying types, promoting code reusability, type safety, and flexibility.
Java generic return type same as argument
I'd be happy to help you with that!
Java's generics were introduced in version J2SE 5.0, released in 2004. Generics allow for more flexibility and type safety in your code by allowing you to specify the types of objects being manipulated within a class or method.
One important aspect of Java generics is the concept of "type erasure". When you compile a generic class or method, the compiler replaces the generic types with their corresponding wildcard (?
) or bounded wildcard (? extends ClassOrInterface
). This means that when you use a generic class or method at runtime, it doesn't contain any information about its type parameters.
In Java, the return type of a generic method can be inferred from the argument passed to the method. For example:
public static T getFirst(List list) {
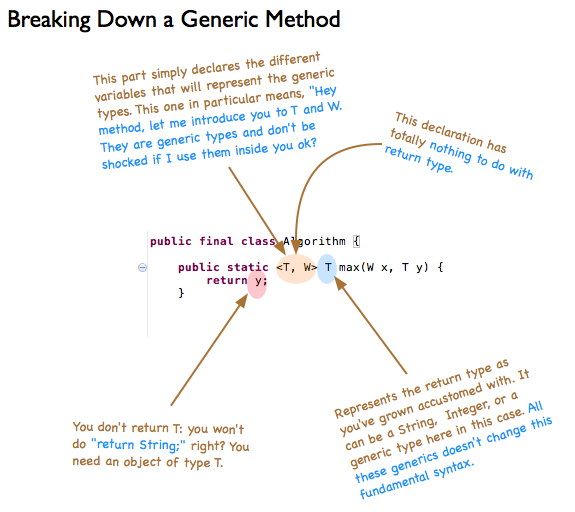
return list.get(0);
}
In this example, the getFirst
method takes a List
of any type (T
) and returns its first element. The compiler infers that the return type should be the same as the argument's type (T
). This means you can call the method like this:
Integer result = getFirst(Arrays.asList(1, 2, 3));
In this case, getFirst
will return an Integer
, which is a perfect match for your expected return type. Similarly, if you call the method with a list of strings:
String result = getFirst(Arrays.asList("one", "two", "three"));
The method will return a String
. This feature provides flexibility in your code and reduces the need to write separate methods or classes for each data type.
However, it's important to note that the compiler cannot always infer the return type. If you have multiple overloads of a generic method, the compiler can't determine which one is being called based on the argument's type. In such cases, you would need to specify the return type explicitly using the @Override
annotation:
public static T getFirst(List list) {
// method implementation
}
@Override public Integer getFirst(List list) {
// overridden method implementation
}
In summary, Java generics allow you to write more flexible and type-safe code. The return type of a generic method can often be inferred from the argument passed to the method, but in some cases, you may need to specify it explicitly using annotations.
Word count: 299