What is a nested loop in Java?
What is a nested loop in Java?
A fundamental concept in programming!
In Java, a nested loop refers to a situation where one loop is enclosed within another loop. This means that the outer loop will execute multiple times, and each iteration of the outer loop can contain multiple iterations of the inner loop.
Let's break it down:
Outer Loop: The outer loop is the one that executes first. It's like a parent, giving birth to the inner loop. The outer loop typically has its own conditions for terminating (e.g., i < 5
in for (int i = 0; i < 5; i++)
) and will execute multiple times.
Inner Loop: The inner loop is the one that's enclosed within the outer loop. It's like a child, born from the outer loop. The inner loop has its own conditions for terminating (e.g., j < 3
in for (int j = 0; j < 3; j++)
) and will execute multiple times for each iteration of the outer loop.
Here's an example to illustrate this:
for (int i = 0; i < 5; i++) {
System.out.println("Outer Loop: " + i);
// Inner Loop
for (int j = 0; j < 3; j++) {
System.out.println("Inner Loop: " + j);
}
}
When you run this code, the output will be:
Outer Loop: 0
Inner Loop: 0
Inner Loop: 1
Inner Loop: 2
Outer Loop: 1
Inner Loop: 0
Inner Loop: 1
Inner Loop: 2
Outer Loop: 2
Inner Loop: 0
Inner Loop: 1
Inner Loop: 2
Outer Loop: 3
Inner Loop: 0
Inner Loop: 1
Inner Loop: 2
Outer Loop: 4
Inner Loop: 0
Inner Loop: 1
Inner Loop: 2
As you can see, the outer loop executes five times, and each time it contains three iterations of the inner loop. The inner loop's iteration count is independent of the outer loop's iteration count.
When to use nested loops: You'll often encounter situations where a nested loop is necessary:
Iterating over multiple collections or arrays: When you need to iterate over multiple arrays or collections and perform some operation on each element. Processing hierarchical data structures: Nested loops are useful when working with tree-like data structures, such as XML or JSON files. Implementing algorithms that require nested iterations: For example, algorithms like Breadth-First Search (BFS) or Depth-First Search (DFS) in graph theory often rely on nested loops.Best practices:
Keep it simple: Avoid complex logic within your inner loop. Keep the inner loop's code concise and focused on its specific task. Use meaningful variable names: When using multiple loops, use descriptive variable names to help you (and others) understand what each loop is doing.In conclusion, nested loops in Java are a powerful tool for iterating over data structures and performing operations that require hierarchical or recursive processing. By mastering this concept, you'll be better equipped to tackle complex programming challenges!
W3schools java loops examples
Here are some examples of Java loops from W3Schools:
For Loop
A for loop iterates over a sequence (such as an array or a list) and executes a block of code once for each item in the sequence.
Example:
for (int i = 0; i < 5; i++) {
System.out.println("Hello, world!");
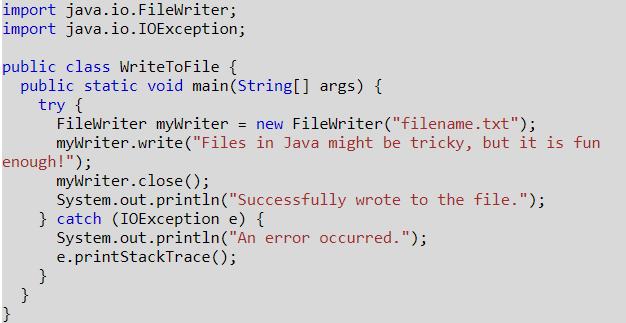
}
Output:
Hello, world!
Hello, world!
Hello, world!
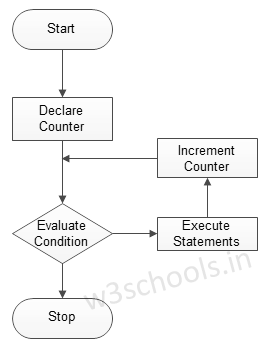
Hello, world!
Hello, world!
While Loop
A while loop executes a block of code as long as a specified condition is true.
Example:
int i = 0;
while (i < 5) {
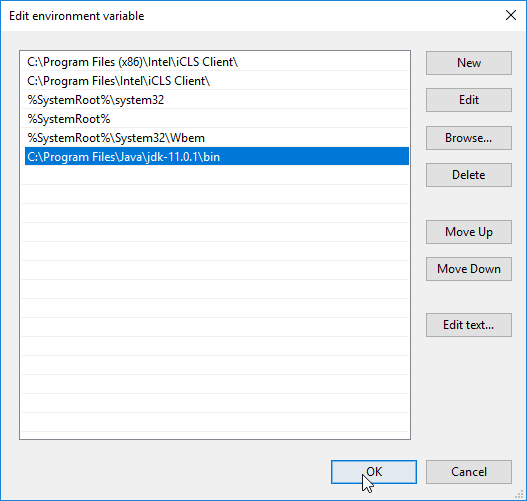
System.out.println("Hello, world!");
i++;
}
Output:
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Do-While Loop
A do-while loop is similar to a while loop, but it executes the block of code at least once before checking the condition.
Example:
int i = 0;
do {
System.out.println("Hello, world!");
i++;
} while (i < 5);
Output:
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Foreach Loop
A foreach loop iterates over a collection (such as an array or a list) and executes a block of code once for each item in the collection.
Example:
String[] fruits = {"Apple", "Banana", "Cherry"};
for (String fruit : fruits) {
System.out.println(fruit);
}
Output:
Apple
Banana
Cherry
Breaking Out of a Loop
Sometimes, you may want to exit a loop early. In Java, you can use the break
statement to do so.
Example:
for (int i = 0; i < 5; i++) {
if (i == 2) {
break;
}
System.out.println(i);
}
Output:
0
1
Loop Labels
You can use a loop label to identify which loop you are referring to when using the break
or continue
statements.
Example:
outer: for (int i = 0; i < 5; i++) {
inner: for (int j = 0; j < 5; j++) {
if (i == 2) {
break outer;
}
System.out.println(i + " " + j);
}
}
Output:
0 0
0 1
0 2
1 0
1 1
These are just a few examples of Java loops from W3Schools. I hope this helps! Let me know if you have any questions or need further clarification.