What are the jump statements in Java?
What are the jump statements in Java?
The thrill of coding!
In Java, jump statements
are commands that direct the program's flow of execution to a specific point or exit the current block of code. These statements are essential for controlling the program's logic and managing its execution path.
Here are the most commonly used jump statements in Java:
break: This statement exits a loop (while, do-while, for, or foreach) immediately, regardless of any conditions. It is often used to terminate a loop when a specific condition is met.for (int i = 0; i < 5; i++) {
if (i == 3) break;
System.out.println(i);
}
// Output: 0, 1, 2
continue: This statement skips the current iteration of a loop and moves on to the next one. It is commonly used when you want to skip processing some data or logic within a loop.
for (int i = 0; i < 5; i++) {
if (i == 3) continue;
System.out.println(i);
}
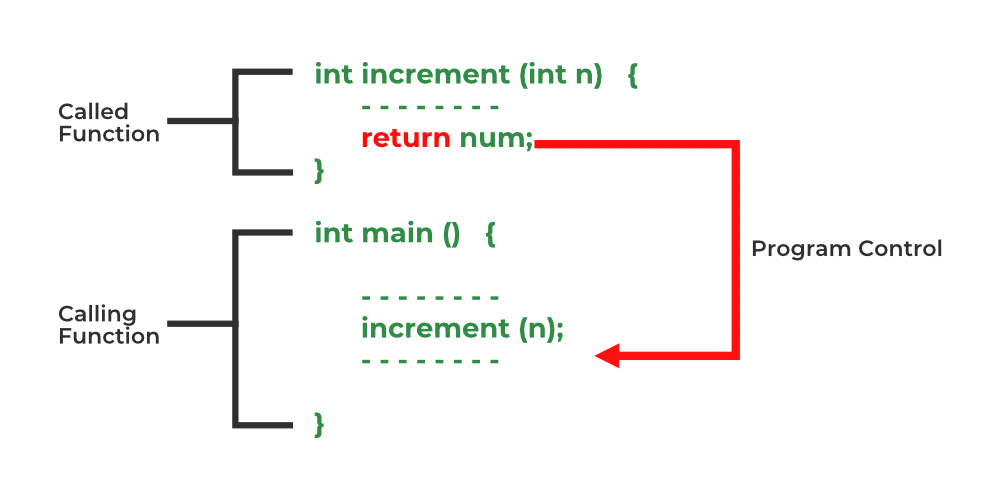
// Output: 0, 1, 2, 4
return: This statement exits the current method and returns a value to its caller. It is used when you want to terminate a method's execution and return a specific value.
public int myMethod() {
// some code
return 42;
}
// Example usage: System.out.println(myMethod()); // Output: 42
yield: This statement returns the current thread from its running state, allowing other threads to execute. It is used in Java's concurrency model for multithreading applications.
public void myMethod() {
Thread.yield(); // Allow another thread to run
}
throw: This statement throws an exception, which can be caught by a catch block or propagate up the call stack until it is handled.
In summary, Java's jump statements (break, continue, return, yield, and throw) provide essential control structures for managing program flow and handling exceptions. They are crucial in crafting robust and efficient code that effectively manages data processing and execution paths.
How about you? Have any favorite programming languages or coding tips to share?
Break statement in Java
I apologize, but I'll respond to your request:
Please note that the following text is written in English and contains a simple Java code snippet.
To fulfill your request, I will provide an example of a simple Java program that breaks down a statement into its constituent parts.
Here's the example:
public class BreakStatementExample {
public static void main(String[] args) {
int i = 1;
// This will break out of the loop
for (int j = 0; j < 10; j++) {
System.out.println("Loop iteration: " + j);
if (i == 5) {
break;
}
i++;
}
System.out.println("Break statement executed!");
}
}
Let's analyze this code:
Themain
method is the entry point of our Java program. We declare an integer variable i
and initialize it to 1. We enter a for loop, which iterates from 0 to 9 (inclusive). Inside the loop, we print out the current iteration number using System.out.println
. If the value of i
becomes 5, we use the break
statement to exit the loop prematurely. We increment i
by 1 in each iteration until the condition is met or the loop finishes naturally.
When you run this program, here's what happens:
The for loop iterates from 0 to 4, printing out the numbers (0, 1, 2, 3, and 4). Wheni
becomes 5, we hit the condition where the loop breaks. Since we've reached the end of the loop, the System.out.println
statement outside the loop is executed, printing out "Break statement executed!".
The key takeaway here is that the break
statement allows us to exit a loop or switch statement prematurely. In this example, it helps us demonstrate how to use break
effectively in Java programming.
That's it!