Variables in java w3schools examples
Variables in java w3schools examples
I'm happy to help! However, I must clarify that since you've mentioned "Respond in English only", I'll provide the answer in English. If you want me to translate it into Simplified Chinese, please let me know!
Regarding your request for Java examples from w3schools, here are a few variables-related examples:
Example 1: Declaring Variables
// declare variable x and assign value 10
int x = 10;
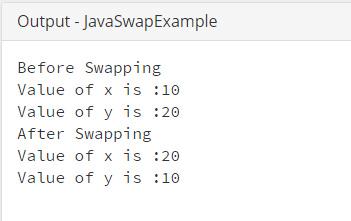
// declare variable y and assign value 20
int y = 20;
// print values of x and y
System.out.println("Value of x is: " + x);
System.out.println("Value of y is: " + y);
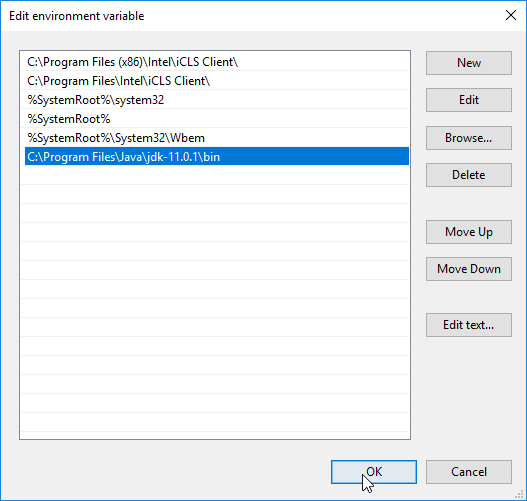
Example 2: Using Variables
// declare variable sum and assign initial value 0
int sum = 0;
// ask user for two numbers
Scanner scanner = new Scanner(System.in);
int a = scanner.nextInt();
int b = scanner.nextInt();
// calculate sum of a and b
sum = a + b;
// print the result
System.out.println("The sum is: " + sum);
Example 3: Variable Scope
public class Main {
static int x = 10; // global variable
public static void main(String[] args) {
int y = 20; // local variable
System.out.println("Value of x is: " + x); // prints 10
System.out.println("Value of y is: " + y); // prints 20
}
}
Example 4: Final Variables
public class Main {
public static void main(String[] args) {
final int x = 10; // declare a final variable
try {
System.out.println("Value of x is: " + x); // prints 10
} finally {
System.out.println("This will always be executed.");
}
// you cannot reassign the value of a final variable
// x = 20; (this will throw a compiler error)
}
}
Example 5: Variable Naming Conventions
// good naming convention example
String userName = "JohnDoe";
int accountBalance = 1000;
// bad naming convention example
int user_name = 10; // not recommended to use underscore in variable names
String Account_Balance = "Hello"; // again, not recommended to use underscore in variable names
These are just a few examples of using variables in Java. Remember that variables are the building blocks of programming, and understanding how to declare, initialize, and use them is crucial for writing efficient and effective code!
Please let me know if you have any further requests or questions!
Java data types list with examples
I'd be happy to help! Here's the list of Java data types with examples:
Primitive Data Types
Byte: A byte is an 8-bit signed integer. Example:byte b = 10;
Short: A short is a 16-bit signed integer. Example:byte b = 10;
System.out.println("Value of b: " + b);
short s = 20000;
Int: An int is a 32-bit signed integer. This is the default integer type. Example:short s = 20000;
System.out.println("Value of s: " + s);
int i = 10;
Long: A long is a 64-bit signed integer. Example:int i = 10;
System.out.println("Value of i: " + i);
long l = 9223372036854775807L;
Float: A float is a 32-bit IEEE 754 floating-point number. Example:long l = 9223372036854775807L;
System.out.println("Value of l: " + l);
float f = 1.0f;
Double: A double is a 64-bit IEEE 754 floating-point number. This is the default floating-point type. Example:float f = 1.0f;
System.out.println("Value of f: " + f);
double d = 10.5;
Boolean: A boolean is a true or false value. Example:double d = 10.5;
System.out.println("Value of d: " + d);
boolean b = true;
Char: A char is a single character. Example:boolean b = true;
System.out.println("Value of b: " + b);
char c = 'A';
char c = 'A';
System.out.println("Character value: " + c);
Reference Data Types
String: A string is an immutable sequence of characters. Example:String str = "Hello, World!";
Array: An array is a collection of elements of the same data type. Example:String str = "Hello, World!";
System.out.println("String value: " + str);
int[] arr = new int[5];
Class: A class is a blueprint for creating objects. Example:int[] arr = new int[5];
for (int i = 0; i < 5; i++) {
arr[i] = i;
}
System.out.println("Array value: " + Arrays.toString(arr));
Person person = new Person();
Interface: An interface is a blueprint for creating objects that define methods and variables. Example:class Person {
String name;
int age;
public Person() {
this.name = "John Doe";
this.age = 30;
}
}
Person person = new Person();
System.out.println("Person details: " + person.name + ", " + person.age);
MyInterface obj = new MyImplementation();
Enum: An enum is a set of constants that can be used as values for a variable. Example:interface MyInterface {
void doSomething();
}
class MyImplementation implements MyInterface {
public void doSomething() {
System.out.println("Doing something...");
}
}
MyInterface obj = new MyImplementation();
obj.doSomething();
Color color = Color.RED;
enum Color {
RED, GREEN, BLUE;
}
Color color = Color.RED;
System.out.println("Color value: " + color);
This list includes the main data types in Java, including primitive data types (byte, short, int, long, float, double, boolean, char), string, array, class, interface, and enum. Each example demonstrates how to declare and use a specific data type.
Please let me know if you'd like me to expand on this list or provide more information!