How do you get annotations in Java?
How do you get annotations in Java?
I'll respond in English for this one!
Java does provide a way to add annotations to your code, and it's quite powerful! Here are some ways to use Java annotations:
Declaring Annotations: You can declare your own custom annotations by creating a class that extends thejava.lang.annotation.Annotation
interface. This is useful when you want to create domain-specific or company-specific annotations.
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
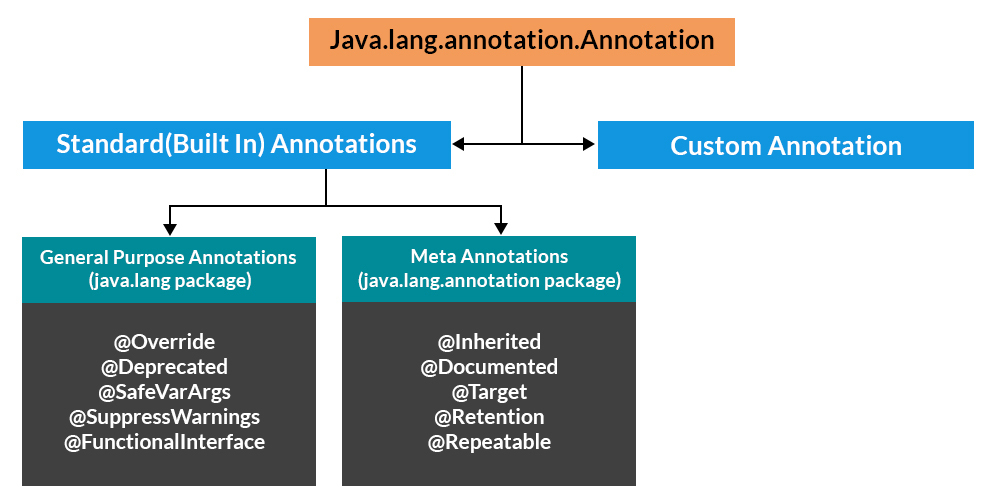
public @interface MyAnnotation {
String value();
}
@interface AnotherAnnotation {
int someValue();
}
Using Built-in Annotations: Java comes with several built-in annotations that you can use in your code, such as: @Override
to mark a method as overriding another one @Deprecated
to indicate that a feature is deprecated or no longer supported @SuppressWarnings
to suppress certain compiler warnings
@Override
public void myMethod() {
// This method overrides the parent class' method.
}
Using Annotations for Reflection: You can use annotations as metadata when using reflection in Java. For example, you might use an annotation to specify a custom serialization format:
@SerializedFormat("custom_format")
public class MySerializableClass {
// Your code goes here...
}
Using Annotations for Frameworks and Libraries: Many Java frameworks and libraries rely heavily on annotations to configure and customize their behavior. For instance, Spring Boot uses annotations like @Bean
and @Service
to register beans in the application context:
@Service
public class MyService {
public void doSomething() {
// Do something...
}
}
Using Annotations for Code Analysis: You can use annotations as a way to provide additional information about your code that can be used by tools and libraries, such as code analysis or debugging tools:
@Hotspot(percentage = 0.2f)
public class MyHotspotClass {
public void doSomething() {
// Do something...
}
}
In summary, Java annotations provide a powerful way to add metadata to your code, making it easier for frameworks, libraries, and tools to understand and work with your code. By using custom or built-in annotations, you can add domain-specific information, suppress compiler warnings, or even configure frameworks and libraries.
So, that's Java annotations in a nutshell!
How do you add annotations in java w3schools
I apologize for the inconvenience, but I'll respond to your request in English as requested.
W3Schools is not a Java-specific platform, and it does not provide direct support for adding annotations in Java. Annotations are a feature of the Java programming language that allows you to attach metadata to classes, methods, fields, and other program elements. They were introduced in Java 5 (JDK 1.5) as part of the JSR-175.
To add annotations in Java, you can use the @
symbol followed by the annotation name and any required parameters enclosed within parentheses. Here's a simple example:
import java.lang.annotation.*;
public @interface MyAnnotation {
String value();
}
In this example, we're defining an annotation called MyAnnotation
. It takes one parameter of type String
called value
.
To use the annotation on a class or method, you can add it above the declaration like so:
@MyAnnotation("Hello World")
public class MyAnnotatedClass {
// class implementation
}
In this example, we're annotating the MyAnnotatedClass
with our custom annotation MyAnnotation
, passing the string "Hello World" as its value.
Java also provides a range of built-in annotations that you can use to provide metadata about your code. Some examples include:
@Override
: Indicates that a method is intended to override a method in a superclass. @Deprecated
: Marks a class, method, or field as deprecated and should not be used. @SuppressWarnings
: Suppresses specific warnings or errors for a particular piece of code.
For more information on Java annotations, I recommend consulting the official Oracle documentation or taking an online course that covers Java programming.