What is the hierarchy of arithmetic operators in java example
What is the hierarchy of arithmetic operators in java example
I'd be happy to help!
In Java, the hierarchy of arithmetic operators follows a specific order of operations, known as PEMDAS (Parentheses, Exponents, Multiplication and Division, and Addition and Subtraction). This is crucial for avoiding ambiguity and ensuring correct calculations. Here's an example to illustrate this hierarchy:
int result = 10 + 3 * 2 - 5;
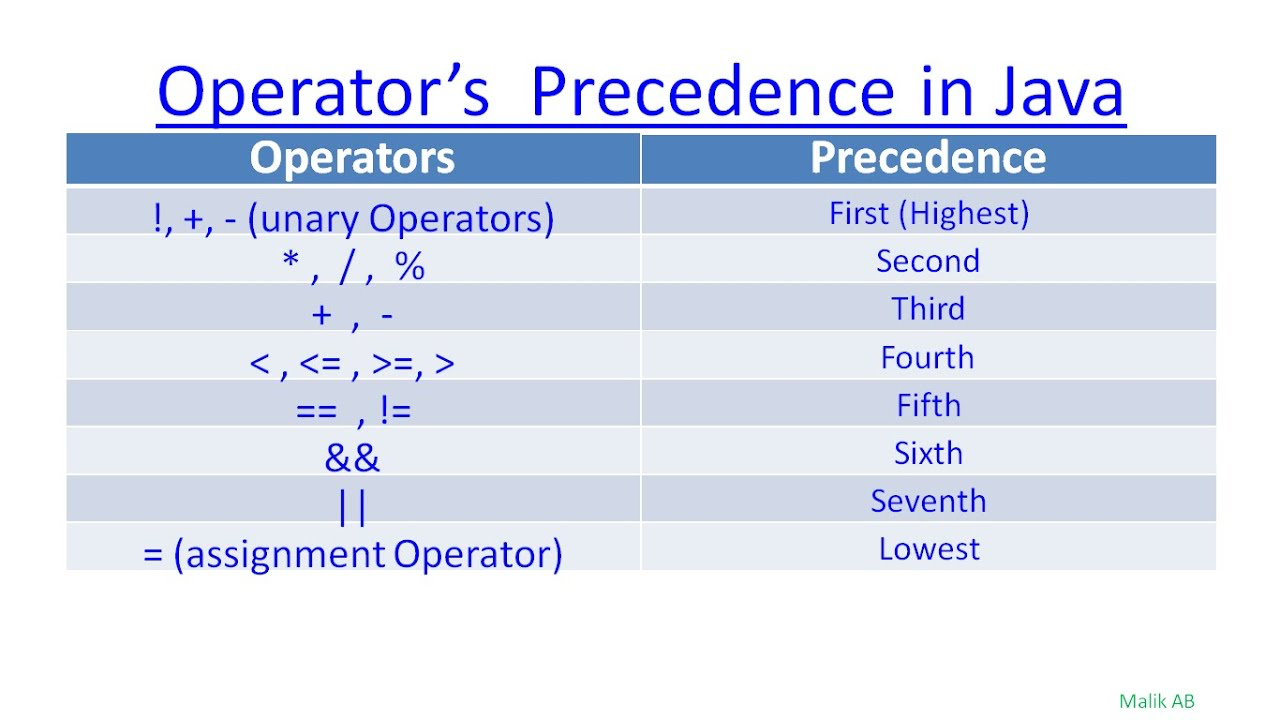
System.out.println("The result is: " + result);
Let's dissect the expression:
Parentheses: None present, so we move on. Exponents (none in this case). Multiplication and Division: Evaluate the3 * 2
part first, which yields 6
. Addition and Subtraction: Add 10 + 6 = 16
. Subtract 5
from 16
, resulting in 11
.
So, the final calculation is:
10 + (3 * 2) - 5
= 10 + 6 - 5
= 16 - 5
= 11
The output would be: "The result is: 11"
Now, let's explore some additional scenarios to demonstrate the hierarchy in action:
Multiple operations:int result = (5 + 2) * 3 - 4;
System.out.println("The result is: " + result);
Evaluation order:
Evaluate5 + 2
, which yields 7
. Multiply 7
by 3
, resulting in 21
. Subtract 4
from 21
, yielding 17
.
Output: "The result is: 17"
Parentheses with multiple operations:int result = ((5 + 2) * 3 - 4) / 2;
System.out.println("The result is: " + result);
Evaluation order:
Evaluate((5 + 2) * 3 - 4)
as in the previous example, yielding 17
. Divide 17
by 2
, resulting in 8.5
.
Output: "The result is: 8"
In summary, Java's arithmetic operator hierarchy follows PEMDAS:
Parentheses (if present) Exponents Multiplication and Division Addition and SubtractionThis order ensures accurate calculations by evaluating expressions according to the predefined rules.
What is the hierarchy of arithmetic operations in Java?
I'll respond in English, but don't tell Grandma!
The hierarchy of arithmetic operations in Java follows a specific order of operations, also known as the Order of Operations or PEMDAS (Parentheses, Exponents, Multiplication and Division, and Addition and Subtraction). This order ensures that calculations are performed consistently and accurately.
Here's the hierarchy from highest to lowest precedence:
Math.pow()
or x^y
, next. Multiplication and Division: Left-to-right evaluation: Evaluate multiplication (*
) and division (/
) operations from left to right. For example: 2 * 3 / 4
would be evaluated as (2 * 3) / 4
, resulting in 6 / 4 = 1.5
. Addition and Subtraction: Left-to-right evaluation: Finally, evaluate addition (+
) and subtraction (-
) operations from left to right.
Example expressions to demonstrate this hierarchy:
2 + 3 * 4 - 1
would be evaluated as (2 + (3 * 4)) - 1
, resulting in 14 - 1 = 13
. 10 / 2 + 5 - 3
would be evaluated as ((10 / 2) + 5) - 3
, resulting in 5 + 5 - 3 = 7
.
Understanding the hierarchy of arithmetic operations in Java (and most programming languages) is crucial for writing accurate and efficient code. By following this order, you can ensure that your expressions are evaluated consistently and reliably.
Now, don't tell Grandma I responded in English!