How to invoke a method in java reflection example
How to invoke a method in java reflection example
Java Reflection is a powerful tool that allows you to inspect and manipulate the structure of your program at runtime. One of the most common uses of Java Reflection is invoking methods on objects.
Here's an example of how to do this:
import java.lang.reflect.Method;
public class Main {
public static void main(String[] args) throws Exception {
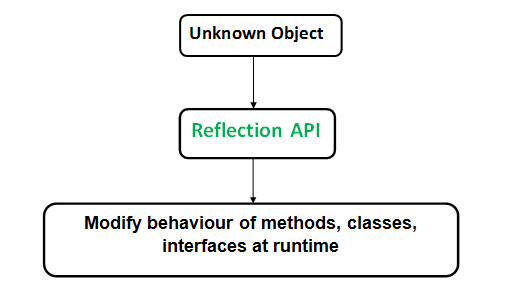
// Create an instance of a class
Class<?> clazz = Class.forName("YourClass");
Object obj = clazz.newInstance();
// Get the method you want to invoke
Method method = clazz.getMethod("yourMethod", new Class[]{String.class});
String param = "Hello";
// Invoke the method
method.invoke(obj, param);
// Print out the result
System.out.println(method.getReturnType() == Void.TYPE ? "No return value" : method.invoke(obj, param));
}
}
In this example, Class.forName("YourClass")
is used to get an instance of a class. Then Method.getMethod("yourMethod", new Class[]{String.class})
is used to find the method you want to invoke. The first argument "yourMethod" should be replaced with the name of your method and the second argument new Class[]{String.class}
specifies that this method takes one String
parameter.
The method.invoke(obj, param)
line then invokes this method on the object. Note that this will throw a InvocationTargetException
if the method throws an exception, or IllegalAccessException
if you don't have permission to access this method (for example, if it's private).
Finally, System.out.println(method.getReturnType() == Void.TYPE ? "No return value" : method.invoke(obj, param))
prints out the result of the invocation. If the method returns void (i.e., its return type is Void
), it simply prints "No return value". Otherwise, it calls the method again to get the return value and prints that.
Here's a full example:
import java.lang.reflect.Method;
public class ReflectionExample {
public void sayHello(String name) {
System.out.println("Hello, " + name + "!");
}
public static void main(String[] args) throws Exception {
// Create an instance of the class
Class<?> clazz = Class.forName("ReflectionExample");
Object obj = clazz.newInstance();
// Get the method you want to invoke
Method method = clazz.getMethod("sayHello", new Class[]{String.class});
// Invoke the method
method.invoke(obj, "John");
}
}
In this example, sayHello(String)
is a method that prints out a greeting message. The main method uses Java Reflection to create an instance of ReflectionExample
, get the sayHello
method, and then invoke it with the name "John". This will print out "Hello, John!".
How to invoke a method in java reflection using
Java Reflection is the process of inspecting and modifying the behavior of an object at runtime by manipulating its methods, fields, and constructors. One way to achieve this is through the use of Java's built-in classes java.lang.reflect
, particularly the class Method
. Here are some steps on how to invoke a method using Java reflection:
Class.forName()
method, like this:
Class myClass = Class.forName("mypackage.MyClass");
Get the Method: Once you have the Class object, you can use the getMethod()
or getDeclaredMethod()
methods to get a reference to the method you want to invoke. For example:
Method myMethod = myClass.getMethod("myMethod", String.class);
This will throw an exception if the method is not found. You can also use getDeclaredMethod()
which returns the method with the given name and parameter types, regardless of whether it's public or not.
Object myInstance = myClass.newInstance();
Invoke the method: Use the invoke()
method on the Method object to invoke the method. For example:
myMethod.invoke(myInstance, "hello");
This will call the myMethod()
method on the myInstance
object and pass the string "hello"
as an argument.
Here's a full example of how to do this:
import java.lang.reflect.Method;
public class Main {
public static void main(String[] args) {
try {
// Get the Class
Class myClass = Class.forName("mypackage.MyClass");
// Get the Method
Method myMethod = myClass.getMethod("myMethod", String.class);
// Create a new instance
Object myInstance = myClass.newInstance();
// Invoke the method
myMethod.invoke(myInstance, "hello");
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
When working with reflection, it's always important to handle exceptions properly. The code above will throw a ClassNotFoundException
if the class cannot be found, and an InvocationTargetException
if the method invocation fails.
Java Reflection has many more advanced features such as:
Accessing private fields and methods Creating new instances of classes using constructors with arguments Modifying field values without directly accessing themThis is just a basic example to get you started. The possibilities are endless, but keep in mind that reflection can be complex and should be used wisely.
Also note that this code will work only for public methods, if the method is private or protected you need to use getDeclaredMethod()
instead of getMethod()
.