What is a template function Java?
What is a template function Java?
I'd be happy to explain what a template function is in Java!
In the world of programming, templates are a powerful concept that allows developers to write code that can work with different data types without explicitly writing separate code for each type.
Java doesn't have direct support for templates like some other languages (e.g., C++), but you might be thinking of something similar. In Java, we use generics to achieve template-like functionality. Generics enable you to create classes and methods that can work with different data types without explicitly writing separate code for each type.
Here's a simple example:
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
@Override
void sound() {
System.out.println("Dog barks");
}
}
class Cat extends Animal {
@Override
void sound() {
System.out.println("Cat meows");
}
}
In this example, the Animal
class has a method called sound
, which is overridden by the Dog
and Cat
classes to provide specific implementations for dogs and cats. This allows you to create an Animal
object that can make different sounds depending on its actual type (either dog or cat).
This concept is similar to templates because it allows you to write code that can work with different data types without explicitly writing separate code for each type. In this case, the sound
method has a template-like behavior where it can be implemented differently based on the actual type of animal.
Templates are useful when you want to reuse code and avoid code duplication, especially when working with generic algorithms or containers. They can also help improve code readability by abstracting away low-level details.
Java doesn't have built-in templates like C++ does, but generics in Java provide similar functionality for creating reusable and type-safe code.
Hope that helps!
Java generic return type same as argument
I'd be happy to help you with that!
Java's generics were introduced in version J2SE 5.0, released in 2004. Generics allow for more flexibility and type safety in your code by allowing you to specify the types of objects being manipulated within a class or method.
One important aspect of Java generics is the concept of "type erasure". When you compile a generic class or method, the compiler replaces the generic types with their corresponding wildcard (?
) or bounded wildcard (? extends ClassOrInterface
). This means that when you use a generic class or method at runtime, it doesn't contain any information about its type parameters.
In Java, the return type of a generic method can be inferred from the argument passed to the method. For example:
public static T getFirst(List list) {
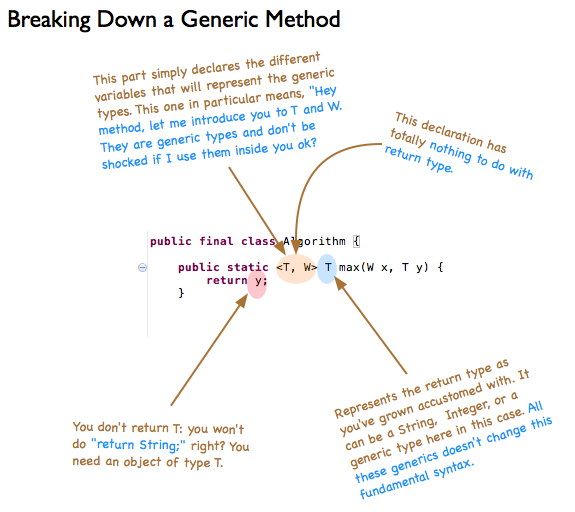
return list.get(0);
}
In this example, the getFirst
method takes a List
of any type (T
) and returns its first element. The compiler infers that the return type should be the same as the argument's type (T
). This means you can call the method like this:
Integer result = getFirst(Arrays.asList(1, 2, 3));
In this case, getFirst
will return an Integer
, which is a perfect match for your expected return type. Similarly, if you call the method with a list of strings:
String result = getFirst(Arrays.asList("one", "two", "three"));
The method will return a String
. This feature provides flexibility in your code and reduces the need to write separate methods or classes for each data type.
However, it's important to note that the compiler cannot always infer the return type. If you have multiple overloads of a generic method, the compiler can't determine which one is being called based on the argument's type. In such cases, you would need to specify the return type explicitly using the @Override
annotation:
public static T getFirst(List list) {
// method implementation
}
@Override public Integer getFirst(List list) {
// overridden method implementation
}
In summary, Java generics allow you to write more flexible and type-safe code. The return type of a generic method can often be inferred from the argument passed to the method, but in some cases, you may need to specify it explicitly using annotations.
Word count: 299