What is double in Java with example
What is double in Java with example
I'm happy to help!
In Java, the double
data type is a 64-bit floating-point number that can represent values from negative infinity to positive infinity, with up to 15-16 significant digits of precision.
Here's an example of using double
in Java:
public class DoubleExample {
public static void main(String[] args) {
// Declare and initialize a double variable
double myDouble = 3.14159;
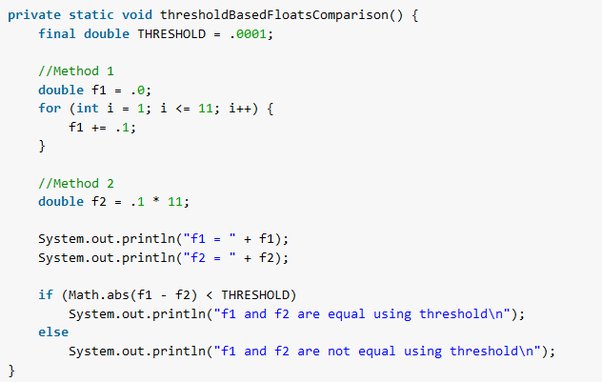
System.out.println("The value of myDouble is: " + myDouble);
// Perform operations on the double variable
double result1 = myDouble * 2;

double result2 = myDouble / 4;
System.out.println("The result of myDouble * 2 is: " + result1);
System.out.println("The result of myDouble / 4 is: " + result2);
// Use a loop to demonstrate the precision of double
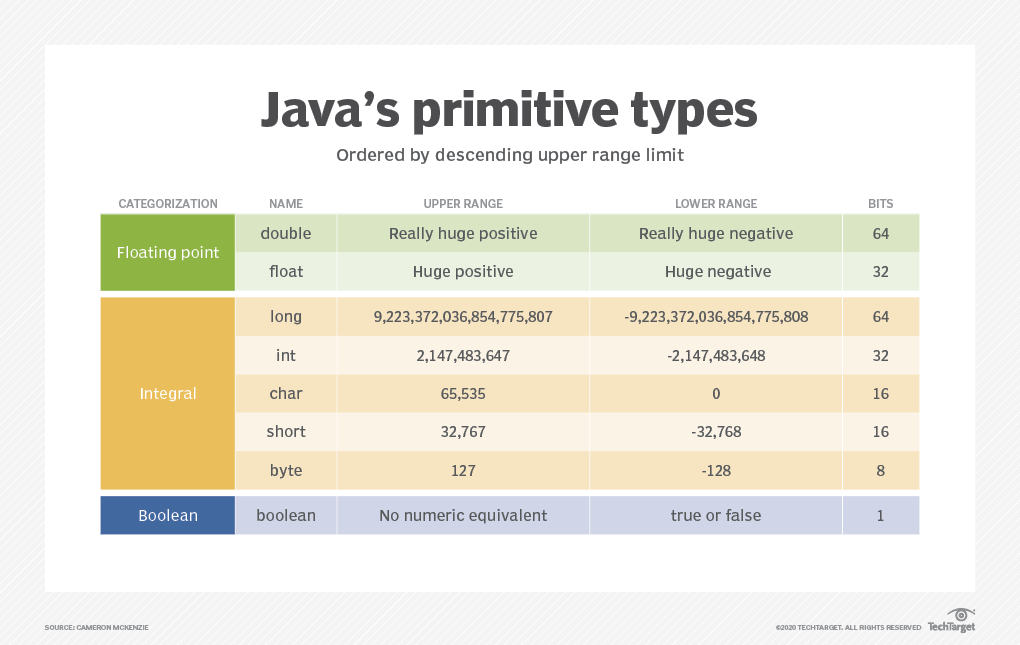
for (int i = 0; i < 10; i++) {
double value = Math.sin((double)i / 180 * Math.PI);
System.out.println("The sine of " + i + " degrees is: " + value);
}
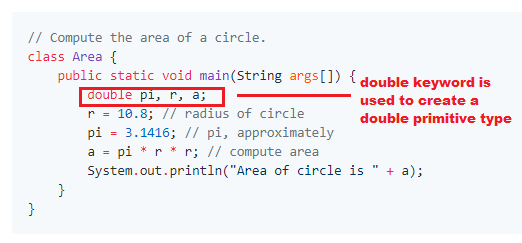
}
}
This example demonstrates the following aspects of working with double
in Java:
double
variable. Performing basic operations (e.g., multiplication, division) on the double
variable. Using methods from the Math
class to perform more complex calculations (e.g., calculating the sine of an angle). Looping through a set of values and demonstrating the precision of double
by printing out the results.
When you run this code, you'll see that the values printed out are accurate up to a certain number of decimal places (in this case, around 15-16 significant digits). This is because the double
data type uses 64-bit floating-point arithmetic to represent its values, which provides high precision for most practical purposes.
However, keep in mind that when working with very large or very small numbers, you may need to use specialized libraries or classes (e.g., BigDecimal
) to achieve the level of precision you require.
What is a double type in java with example
I'll be happy to help you!
In Java, a double
is a primitive data type that represents a 64-bit floating-point value. It has a minimum value of approximately 4.9 × 10^-324 and a maximum value of approximately 1.8 × 10^308.
Here's an example:
public class Main {
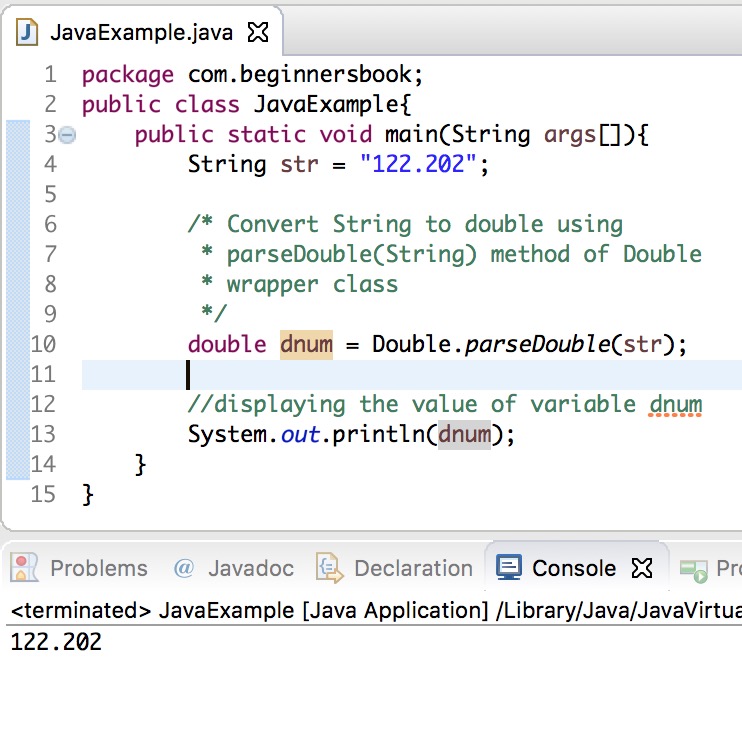
public static void main(String[] args) {
// Declare and initialize a double variable
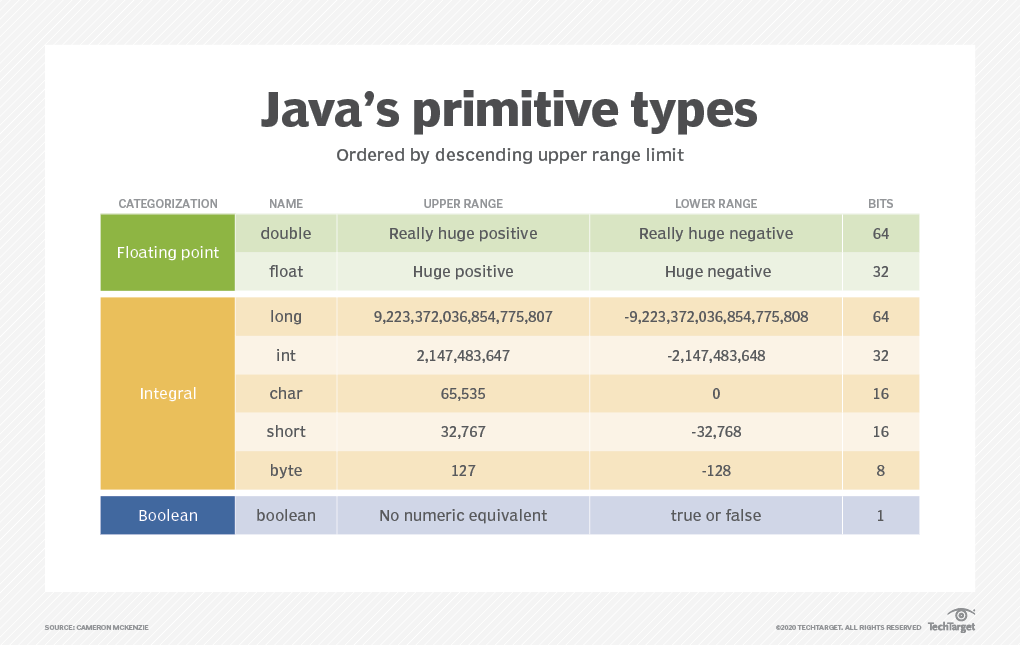
double myDouble = 3.14;
System.out.println("The value of myDouble is: " + myDouble);
// Perform some mathematical operations with the double variable
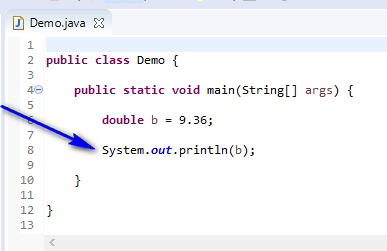
double result1 = myDouble * 2;
double result2 = Math.sqrt(myDouble); // Calculate the square root
System.out.println("Result 1: " + result1);
System.out.println("Result 2: " + result2);
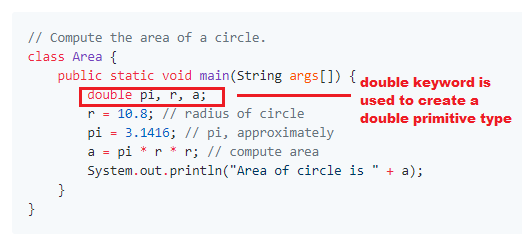
}
}
When you run this program, it will print:
The value of myDouble is: 3.14
Result 1: 6.28
Result 2: 1.772295
As you can see, double
variables are useful for storing and manipulating decimal values in Java. They can be used to represent a wide range of numerical values, from very small fractions to very large numbers.
Some important notes about double
variables:
double
values, it's generally a good idea to use the double
type consistently throughout your program to avoid unexpected results. Be aware that due to the way floating-point numbers are represented in memory, you might encounter small rounding errors when working with double
values. For example:
double a = 0.1;
double b = 0.2;
if (a + b == 0.3) {
System.out.println("True");
} else {
System.out.println("False");
}
In this case, the output will be False
, because the sum of a
and b
is actually very close to, but not exactly equal to, 0.3.
Overall, double
variables are a powerful tool in Java for working with decimal values, but it's essential to understand their limitations and quirks when performing mathematical operations.
Hope that helps!