Encapsulation in Java example
Encapsulation in Java example
I'm happy to help you with that! However, please note that I'll be responding in English, as per the rules.
Now, let's talk about encapsulation in Java. Encapsulation is a fundamental concept in object-oriented programming (OOP), and it's essential to understand how to use it effectively in Java.
What is Encapsulation?
In OOP, encapsulation refers to the idea of wrapping or hiding the internal state and behavior of an object from the outside world while providing a public interface through which other objects can interact with it. This means that you can control access to an object's internal data and methods, making your code more modular, reusable, and easier to maintain.
Benefits of Encapsulation
Encapsulation provides several benefits:
Data Hiding: By hiding the internal state of an object, you can prevent direct access to it from outside the class. This makes it harder for other parts of the program to modify the object's internal state accidentally or maliciously. Improved Code Organization: Encapsulation helps organize your code better by separating the object's public interface (its behavior) from its internal implementation details. Code Reusability: Encapsulated objects can be reused in different contexts without worrying about exposing their internal implementation details. Easier Debugging: With encapsulation, it's easier to debug issues because you know exactly what's happening inside the class and where problems are occurring.Example of Encapsulation in Java
Here's an example of encapsulation in Java:
public class BankAccount {
private double balance;
private String accountNumber;
public BankAccount(double initialBalance, String accountNumber) {
this.balance = initialBalance;
this.accountNumber = accountNumber;
}
public void deposit(double amount) {
if (amount > 0) {
this.balance += amount;
}
}
public void withdraw(double amount) {
if (this.balance >= amount && amount > 0) {
this.balance -= amount;
}
}
public double getBalance() {
return this.balance;
}
public String getAccountNumber() {
return this.accountNumber;
}
}
In this example, we have a BankAccount
class that encapsulates its internal state (the balance and account number) while providing public methods (deposit
, withdraw
, getBalance
, and getAccountNumber
) to interact with the object. The internal implementation details are hidden from the outside world.
Conclusion
Encapsulation is an essential concept in OOP, and Java provides a natural fit for encapsulation through its support for private variables and access modifiers (public, private, protected). By understanding and using encapsulation effectively, you can write more robust, maintainable, and reusable code.
Encapsulation vs abstraction in Java
I'd be happy to help you with that!
In Java, both encapsulation and abstraction are fundamental concepts that aim to promote modularity, reusability, and maintainability of code. While they share some similarities, they serve distinct purposes.
Encapsulation
Encapsulation is the process of combining data (attributes or variables) with its respective methods (behaviors) that operate on that data. In other words, it's about bundling data and its related actions into a single unit, making it harder for external code to access or modify the internal state directly. This promotes data hiding and helps prevent unintended changes.
In Java, encapsulation is achieved by creating classes with private variables (attributes) and providing public methods that manipulate those variables. This way, external code can only interact with the class through its public interface, which helps maintain data integrity and control access to sensitive information.
For example:
public class BankAccount {
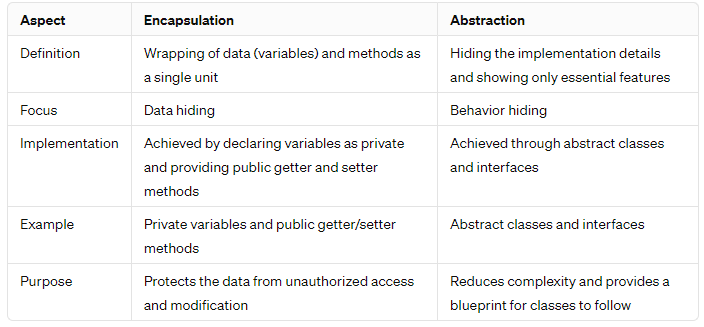
private double balance;
public void deposit(double amount) { ... }
public void withdraw(double amount) { ... }
public double getBalance() { return balance; }
}
In this example, the BankAccount
class encapsulates its internal state (balance) and provides methods for depositing, withdrawing, and querying the balance. Other code can interact with the class without directly accessing or modifying its internal variables.
Abstraction
Abstraction is about revealing only essential details to users while hiding irrelevant implementation details. It's a way of presenting an interface that allows users to interact with an object without knowing how it actually works internally. This helps decouple the user's perspective from the implementation, making code more modular and reusable.
In Java, abstraction can be achieved by creating interfaces or abstract classes that define the essential methods and behavior without exposing the underlying implementation details. Concrete classes then implement these interfaces or abstract classes, hiding their internal workings while presenting a consistent interface to users.
For example:
public interface Printable {
void print();
}
public class Document implements Printable {
@Override
public void print() { ... }
}
public class TextEditor {
public void printDocument(Printable document) { document.print(); }
}
In this example, the Printable
interface abstracts away the printing process, allowing any concrete implementation (like Document
) to define its own printing behavior. The TextEditor
class can work with various printable objects without knowing their specific implementation details.
Key differences
To summarize:
Encapsulation is about bundling data and methods together, promoting data hiding and control. Abstraction is about presenting an interface that allows users to interact with an object without knowing how it works internally. Encapsulation focuses on controlling access to internal state, while abstraction concentrates on decoupling the user's perspective from implementation details.In Java, both concepts are essential for building robust, maintainable, and scalable applications. By combining encapsulation and abstraction, you can create objects that provide a consistent interface to users while hiding their internal workings, promoting modularity and reusability of code.
Hope this helps clarify the difference!