How to set a loop in java example
How to set a loop in java example
I'd be happy to help you with that!
Setting a loop in Java is crucial for iterating through collections, performing repetitive tasks, and more. There are several types of loops available in Java:
For Loop: This type of loop is used when you know the number of iterations beforehand.int sum = 0;
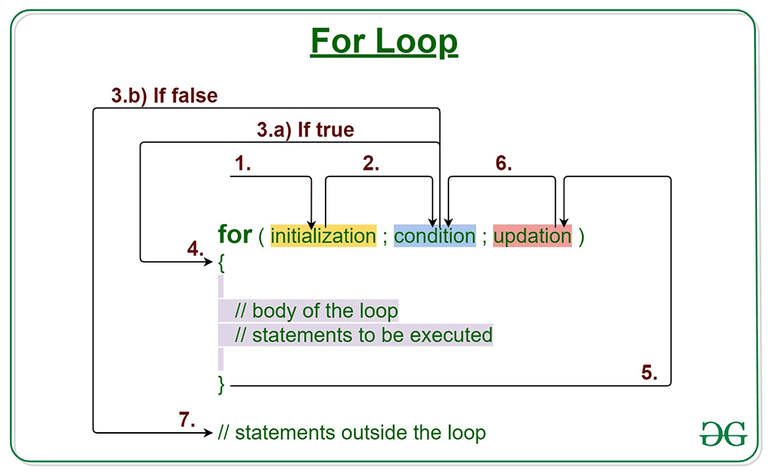
for(int i=0; i<5; i++) {
sum += i;
}
System.out.println("The sum is: " + sum);
In this example, we're calculating the sum of numbers from 0 to 4.
While Loop: This type of loop is used when you don't know the number of iterations beforehand.int count = 0;
while(count < 5) {
System.out.println("Count: " + count);
count++;
}
In this example, we're printing out the value of count
until it reaches 5.
int count = 0;
do {
System.out.println("Count: " + count);
count++;
} while (count < 5);
In this example, we're printing out the value of count
until it reaches 5.
int[] scores = {10, 20, 30, 40};
for(int score : scores) {
System.out.println("Score: " + score);
}
In this example, we're printing out the values in the scores
array.
List words = Arrays.asList("Hello", "World", "Java");
for(String word : words) {
System.out.println("Word: " + word);
}
In this example, we're printing out the values in the words
list.
Remember to always use a loop that best suits your needs and avoid using unnecessary loops to improve performance.
How to set a loop in java using for loop
I'll respond in English as requested.
Setting a loop in Java is crucial when you need to execute a block of code repeatedly. The most common type of loop used in Java is the for
loop, which can be used to iterate over an array or collection, perform an operation for each element, and repeat it until all elements are processed.
The basic syntax of a for
loop is:
for (initialization; condition; increment) {
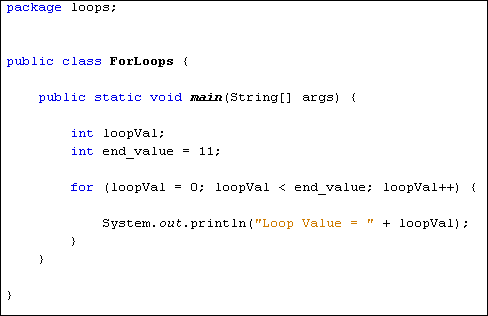
// code block to execute
}
Here's how the different parts work together:
Initialization: This part is executed once, at the beginning of the loop. It sets the starting value for your loop. For example, you can use it to initialize an index variable. Condition: This is the main logic of your loop. The code inside this condition is evaluated each time the loop iterates. If the condition is true, the code block will be executed; if false, the loop will terminate. Increment: This part is also executed at the end of each iteration. It updates the value used in the initialization statement.Here's an example of a simple for
loop:
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.println("Number " + i + " is: " + numbers[i]);
}
This code will print the numbers in the numbers
array to the console. The loop iterates over each element of the array, and for each iteration, it prints the index (i
) and the value at that index.
The key aspects of this example are:
Initialization: The variablei
is set to 0. Condition: The condition i < numbers.length
ensures that the loop runs as long as i
is less than the length of the array (which means we haven't processed all elements). Increment: In each iteration, i
is incremented by 1 (i++
).
When you use a for
loop with these three parts, you can efficiently iterate over an array or collection and perform operations for each element.
Additional Tips:
Always make sure your condition is evaluated at the beginning of each iteration, as shown in this example. This ensures that your loop runs as many times as necessary to complete its task. Use abreak
statement if you want to exit the loop prematurely. Don't forget about continue
, which skips the current iteration but continues with the next one.
By mastering the for
loop, you'll be well-equipped to tackle various programming tasks in Java and other languages!