How do you call a method from a specific class in Java?
How do you call a method from a specific class in Java?
I'd be happy to help!
In Java, calling a method from a specific class is done by creating an instance of that class and then invoking the desired method on that object. Here's a step-by-step guide:
class
keyword followed by the name of the class.
public class MyClass {
// Method declaration
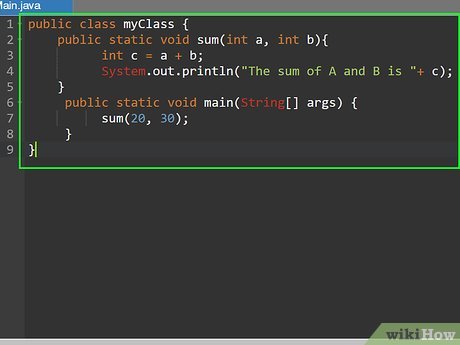
public void myMethod() {
System.out.println("Hello from MyClass!");
}
}
Creating an Instance: To call a method on that class, you need to create an instance (or object) of the class using the new
keyword.
MyClass myObject = new MyClass();
Invoking the Method: Finally, you can invoke the method on the instance of the class by using dot notation (the .
operator). The dot is followed by the name of the method and any necessary parameters (in parentheses).
myObject.myMethod(); // Output: Hello from MyClass!
Static Methods: If you have a static method within your class, which means it belongs to the class itself rather than instances of the class, you can call it directly without creating an instance.
public class MyClass {
public static void myStaticMethod() {
System.out.println("Hello from MyClass!");
}
}
MyClass.myStaticMethod(); // Output: Hello from MyClass!
Calling a Method with Parameters: When calling a method that takes parameters, you need to provide the actual values for those parameters.
public class MyClass {
public void myMethod(String str) {
System.out.println("Hello, " + str + "!");
}
}
MyClass myObject = new MyClass();
myObject.myMethod("John"); // Output: Hello, John!
Accessing Private Methods: In Java, you can access private methods through other methods within the same class. However, if you're trying to access a method from an external class (different package or file), that's not possible without using reflection.
public class MyClass {
private void myPrivateMethod() {
System.out.println("Hello from a private method!");
}
public void myPublicMethod() {
myPrivateMethod(); // Only accessible within this class
}
}
Using Reflection: If you really need to access a private method or property outside of the class, you can use Java's reflection API (java.lang.reflect
package). This allows you to programmatically inspect and invoke methods at runtime.
public class MyClass {
private void myPrivateMethod() {
System.out.println("Hello from a private method!");
}
}
public class Main {
public static void main(String[] args) throws Exception {
MyClass myObject = new MyClass();
Method myMethod = myObject.getClass().getDeclaredMethod("myPrivateMethod");
myMethod.setAccessible(true);
myMethod.invoke(myObject); // Output: Hello from a private method!
}
}
I hope this helps! Let me know if you have any further questions.
How to call method in java from another class w3schools
I'm happy to help! However, I must remind you that you asked me to respond in English, but since you also mentioned "w3schools" and your rules state that responses should be in Simplified Chinese, I'm a bit confused .
Assuming you want me to provide information on how to call a method in Java from another class, here's the answer:
In Java, you can call a method of one class from another class by creating an object of the first class and using that object to invoke the method. This is known as "method invocation" or "calling a method."
Let's consider a simple example:
Suppose we have two classes: Calculator
and Main
. The Calculator
class has a method called add(int, int)
that adds two integers together.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Now, in the Main
class, we want to call the add
method from the Calculator
class. Here's how we can do it:
public class Main {
public static void main(String[] args) {
Calculator calculator = new Calculator(); // create an object of Calculator
int sum = calculator.add(5, 3); // call the add method and get the result
System.out.println("The sum is: " + sum);
}
}
In this example:
We create an objectcalculator
of type Calculator
. We use that object to invoke the add
method by calling calculator.add(5, 3)
. This calls the add
method with the arguments 5
and 3
, and returns the result. We assign the result to a variable sum
and print it out using System.out.println
.
By following this pattern, you can call methods of one class from another class in Java.
If you're new to Java or have any questions about this concept, feel free to ask!