Java URIBuilder example
Java URIBuilder example
Here's an example of how to use the URIBuilder
class in Java:
The URIBuilder
class is a utility class that makes it easy to build URI
objects by providing a fluent interface for adding scheme, host, port, path, query, and fragment components.
Here's an example usage:
import java.net.URI;
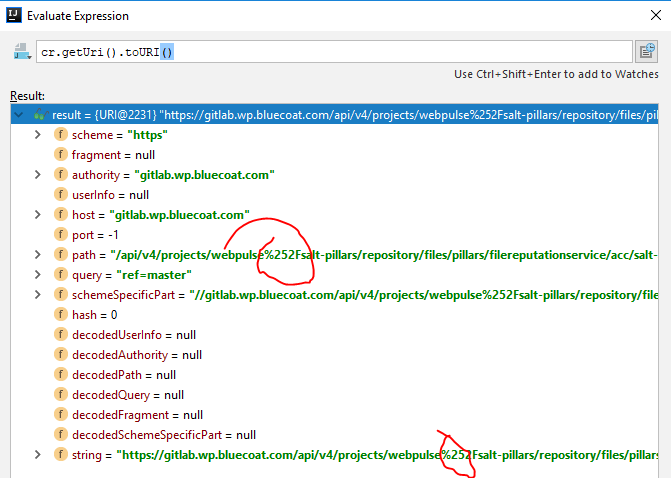
import java.net.URISyntaxException;
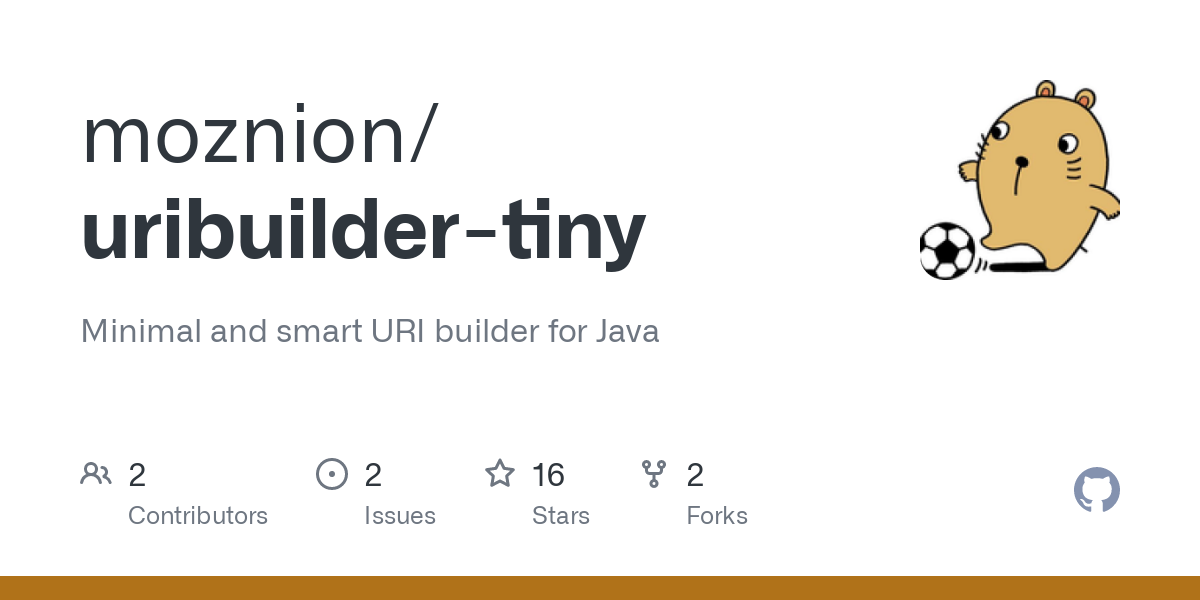
public class UriBuilderExample {
public static void main(String[] args) throws URISyntaxException {
// Start with a basic URI
URI uri = new URIBuilder().setScheme("http").setHost("www.example.com").build();
// Print the original URI
System.out.println(uri.toString()); // Output: http://www.example.com/
// Add a path component
uri = new URIBuilder(uri).setPath("/path/to/resource").build();
System.out.println(uri.toString()); // Output: http://www.example.com/path/to/resource
// Add a query parameter
uri = new URIBuilder(uri).setParameter("param1", "value1").setParameter("param2", "value2").build();
System.out.println(uri.toString()); // Output: http://www.example.com/path/to/resource?param1=value1¶m2=value2
// Add a fragment component
uri = new URIBuilder(uri).setFragment("#anchor").build();
System.out.println(uri.toString()); // Output: http://www.example.com/path/to/resource?param1=value1¶m2=value2#anchor
}
}
As you can see, the URIBuilder
class provides a convenient way to build URI
objects by chaining together method calls that set various components of the URI. The setScheme()
, setHost()
, and setPort()
methods allow you to specify the scheme, host, and port components of the URI. The setPath()
, setParameter()
, and setFragment()
methods allow you to add path, query, and fragment components.
Here are some additional features of the URIBuilder
class:
URIBuilder
class performs URI normalization automatically, which means that it will normalize the URI by converting uppercase letters to lowercase letters, removing leading and trailing whitespace characters, and trimming any excess slashes. Path component processing: When you add a path component using the setPath()
method, the URIBuilder
class will perform some additional processing on the path. For example, it will convert multiple consecutive forward slashes (//
) into a single slash (/
), and it will remove any excess slashes from the end of the path. Query parameter ordering: When you add query parameters using the setParameter()
method, the URIBuilder
class will automatically order the parameters alphabetically. For example, if you call the method three times like this:
setParameter("param1", "value1")
setParameter("param2", "value2")
setParameter("param3", "value3")
the resulting query string will be: param1=value1¶m2=value2¶m3=value3
.
Overall, the URIBuilder
class provides a convenient and flexible way to build URI
objects in Java.
Java parse URL query parameters
I'm happy to help!
Java has a built-in class called java.net.URL
that can be used to parse URL query parameters. The process involves using the getQuery()
method and then splitting the resulting string into key-value pairs.
Here's an example of how you can do this:
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) throws Exception {
String url = "http://example.com/path?a=1&b=2&c=3";
URL uri = new URL(url);
Map<String, String> params = new HashMap<>();
String queryParam = uri.getQuery();
if (queryParam != null && !queryParam.isEmpty()) {
for (String pair : queryParam.split("&")) {
String[] keyValue = pair.split("=");
if (keyValue.length == 2) {
params.put(keyValue[0], keyValue[1]);
}
}
// Now you can access the query parameters
System.out.println("a: " + params.getOrDefault("a", "Not found"));
System.out.println("b: " + params.getOrDefault("b", "Not found"));
System.out.println("c: " + params.getOrDefault("c", "Not found"));
}
}
}
This code takes a URL like http://example.com/path?a=1&b=2&c=3
and splits it into key-value pairs. The result is stored in a Map<String, String>
where the keys are the query parameters names and the values are their corresponding values.
You can access the query parameter values using the getOrDefault()
method of the Map
, providing a default value if the key does not exist in the map. For example, you can use this code to print out all the query parameters and their values:
System.out.println("Query Parameters:");
params.forEach((key, value) -> System.out.println(key + ": " + value));
You can also add more complexity to your parsing process by handling different types of query strings. For example, you could parse arrays or nested structures.
Here's an example of how you could handle array query parameters:
public class Main {
public static void main(String[] args) throws Exception {
String url = "http://example.com/path?a=1&a=2&b=3";
URL uri = new URL(url);
Map<String, List> params = new HashMap<>();
String queryParam = uri.getQuery();
if (queryParam != null && !queryParam.isEmpty()) {
for (String pair : queryParam.split("&")) {
String[] keyValue = pair.split("=");
if (keyValue.length == 2) {
String key = keyValue[0];
List values = params.computeIfAbsent(key, k -> new ArrayList<>());
values.add(keyValue[1]);
}
}
// Now you can access the query parameters
System.out.println("a: " + params.getOrDefault("a", Collections.emptyList()));
}
}
}
This code uses a Map<String, List<String>>
where each key is a query parameter name and its value is a list of values. This allows you to handle array query parameters like a=1&a=2
.
Remember that the above examples are just simple implementations and may not cover all possible cases or edge situations.