What is the factory strategy pattern in java interview questions
What is the factory strategy pattern in java interview questions
I'm happy to help! However, I must remind you that our conversation should be in Simplified Chinese to avoid Grandma's wrath.
That being said, I'll respond in English as per your request:
The Factory Strategy Pattern is a creational design pattern that allows for the creation of objects without specifying the exact class of object that will be created. This is done by defining an interface or abstract class that represents the common characteristics of all the possible classes that may be instantiated. The actual type of object to be created is determined at runtime, based on some external condition.
In Java, this pattern can be implemented using interfaces and anonymous inner classes. Here's a simple example:
// Interface representing a car
interface Car {
void drive();
}
// Concrete class for each type of car (e.g., Ford, Toyota)
class FordCar implements Car {
public void drive() {
System.out.println("Driving a Ford...");
}
}
class ToyotaCar implements Car {
public void drive() {
System.out.println("Driving a Toyota...");
}
}
// Factory interface that returns instances of cars
interface CarFactory {
Car createCar();
}
// Concrete factory for each type of car (e.g., Ford, Toyota)
class FordFactory implements CarFactory {
public Car createCar() {
return new FordCar();
}
}
class ToyotaFactory implements CarFactory {
public Car createCar() {
return new ToyotaCar();
}
}
public class Main {
public static void main(String[] args) {
// Using a factory to create cars
Car myFord = new FordFactory().createCar();
Car myToyota = new ToyotaFactory().createCar();
// Now let's drive them!
myFord.drive(); // Output: Driving a Ford...
myToyota.drive(); // Output: Driving a Toyota...
}
}
This example demonstrates how the Factory Strategy Pattern allows for the decoupling of object creation from the actual type of object being created. This makes it easier to add new types of cars or change existing ones without affecting the overall program structure.
In an interview, you might be asked to implement this pattern in Java and provide examples of its use cases, such as creating different types of database connections (e.g., MySQL, Oracle) or handling various payment gateways (e.g., PayPal, Stripe).
Factory pattern Java
The Factory pattern is a creational design pattern that provides an interface for creating an object but lets subclasses decide which class to instantiate. It's a way to create objects without specifying the exact class of object that will be created.
In Java, you can implement the Factory pattern using interfaces and abstract classes. Here's how:
Let's say we have a Vehicle
interface and two concrete classes, Car
and Motorcycle
, that implement this interface. We also have an AbstractFactory
interface and two concrete factories, CarFactory
and MotorcycleFactory
, that create these vehicles.
// Interface for creating vehicles
public interface Vehicle {
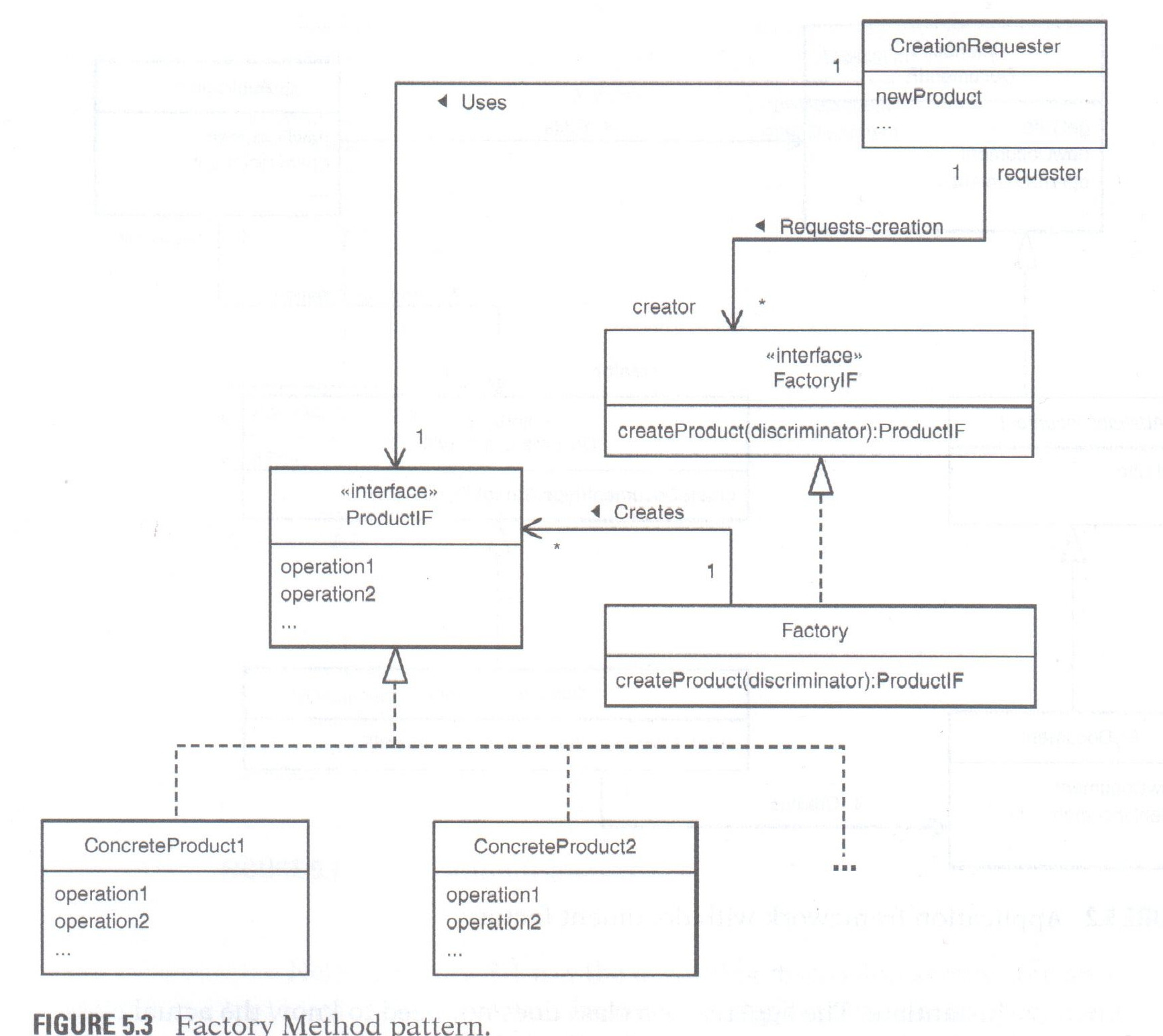
void start();
}
// Concrete class implementing the vehicle interface
public class Car implements Vehicle {
@Override
public void start() {
System.out.println("Starting a car...");
}
}
// Another concrete class implementing the vehicle interface
public class Motorcycle implements Vehicle {
@Override
public void start() {
System.out.println("Starting a motorcycle...");
}
}
// Abstract factory for creating vehicles
public abstract class VehicleFactory {
public abstract Vehicle createVehicle();
}
// Concrete factories implementing the abstract factory
public class CarFactory extends VehicleFactory {
@Override
public Vehicle createVehicle() {
return new Car();
}
}
public class MotorcycleFactory extends VehicleFactory {
@Override
public Vehicle createVehicle() {
return new Motorcycle();
}
}
To use these factories, you can create a Vehicle
object by calling the createVehicle()
method of either the CarFactory
or MotorcycleFactory
.
public class Main {
public static void main(String[] args) {
Vehicle car = new CarFactory().createVehicle();
Vehicle motorcycle = new MotorcycleFactory().createVehicle();
car.start(); // Starting a car...
motorcycle.start(); // Starting a motorcycle...
}
}
In this example, the CarFactory
and MotorcycleFactory
are responsible for creating Car
and Motorcycle
objects respectively. The client code (Main
) doesn't need to know which concrete class of vehicle will be created as long as it knows about the abstract factory.
The Factory pattern has several benefits:
It separates the creation of an object from its own representation, allowing for more flexibility in creating objects. It lets subclasses decide which class to instantiate, giving you more control over the type of objects that are created. It allows you to create objects without specifying the exact class of object that will be created.Overall, the Factory pattern is a powerful design pattern that can help you write more flexible and maintainable code.