while loop in javascript
while loop in javascript
I'm happy to respond in English for you!
Now, about that while loop in JavaScript...
The while
loop is one of the most common types of loops used in programming languages, including JavaScript. It's called a "loop" because it repeats a set of instructions until some condition becomes false.
In JavaScript, the basic syntax of a while
loop is as follows:
while (condition) {
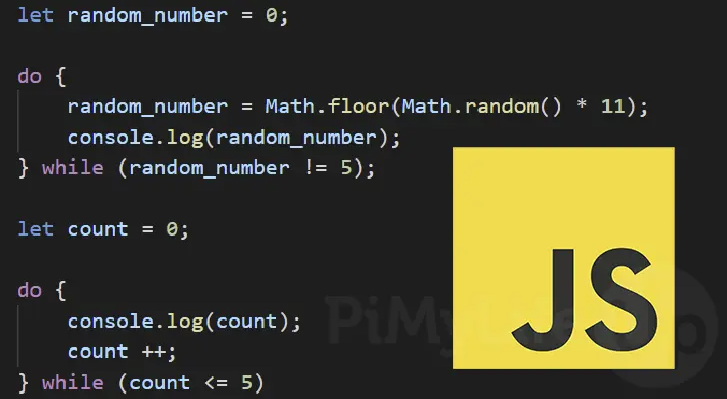
// code to be executed
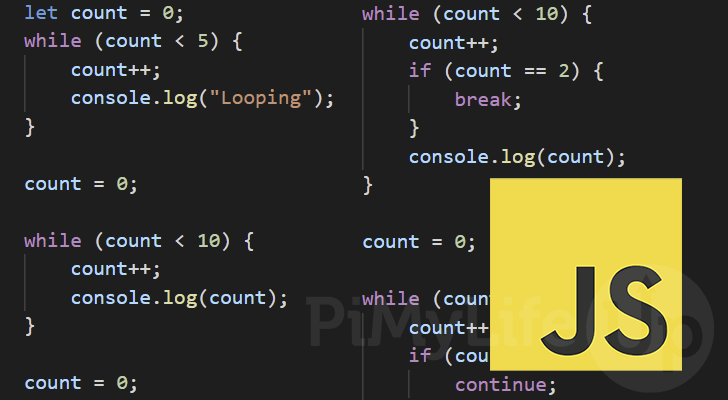
}
The condition inside the parentheses is evaluated first. If it's true, then the code within the block is executed. This process continues until the condition becomes false.
Let me break it down further:
Initialization: Thewhile
loop starts with an initialization step, where you set up the initial value of a variable or expression that will be tested in each iteration. Condition: The next part is the condition, which is evaluated in each iteration. If the condition is true, then the code within the block is executed. Code to be executed: This is the block of code that you want to repeat until the condition becomes false. It can contain any JavaScript statements, including variables, functions, and logic.
Here's a simple example:
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
In this example:
i
is initialized to 0. The condition is i < 5
, which means the loop will run as long as i
is less than 5. Inside the block, we log the current value of i
and increment it by 1 using i++
. When i
reaches 5, the condition becomes false, and the loop terminates.
In addition to the classic syntax, JavaScript also has a few other variations:
Do-while: This is similar to the regularwhile
loop, but with the condition evaluated after executing the block.
let i = 0;
do {
console.log(i);
i++;
} while (i < 5);
In this example, we execute the code first, and then check if the condition is true. If it's false, we exit the loop.
For-in: This loop is designed specifically for iterating over arrays or objects.const arr = ['apple', 'banana', 'cherry'];
for (let fruit of arr) {
console.log(fruit);
}
In this example, fruit
takes on each value from the arr
array in each iteration.
for...in
loop, but it's specifically designed for arrays or iterable objects.
const arr = [1, 2, 3];
for (let num of arr) {
console.log(num);
}
In this example, num
takes on each value from the arr
array in each iteration.
And that's a basic overview of while
loops and some variations in JavaScript!
difference between while and do-while loop in java
The age-old question: what's the difference between while
and do-while
loops in Java?
In Java, both while
and do-while
are control flow statements that allow you to execute a block of code repeatedly based on a condition. The main difference between them lies in when they check the condition.
While Loop
A while
loop checks the condition before executing the block of code. Here's the syntax:
while (condition) {
// code to be executed
}
The flow is as follows:
Check the condition. If true, execute the code inside the loop. Repeat step 1.For example:
int i = 0;
while (i < 5) {
System.out.println("Hello!");
i++;
}
This will print "Hello!" five times.
Do-While Loop
A do-while
loop, on the other hand, executes the code block at least once before checking the condition. Here's the syntax:
do {
// code to be executed
} while (condition);
The flow is as follows:
Execute the code inside the loop. Check the condition. If true, repeat step 1.Here's an example:
int i = 0;
do {
System.out.println("Hello!");
i++;
} while (i < 5);
This will also print "Hello!" five times.
Key differences
So, what are the main differences between while
and do-while
?
while
loop, the condition is checked before executing the code. In a do-while
loop, the code is executed at least once before checking the condition. Minimum iteration: A while
loop might never execute if the initial condition is false. A do-while
loop always executes the code block at least once.
When to use each?
Use awhile
loop when you need to perform some action repeatedly based on a condition, and you want to ensure that the condition is met before performing the action. Use a do-while
loop when you want to execute a block of code at least once, regardless of the condition. This is useful for situations where you need to perform some setup or initialization before checking the condition.
In summary, both while
and do-while
loops are used to repeat a block of code, but they differ in their order of execution and minimum iteration requirements. Choose wisely!