What are the three categories of exceptions in Java?
What are the three categories of exceptions in Java?
In Java, there are several types of exceptions that can occur during the execution of a program. The main categories of exceptions are:
Checked Exceptions: These exceptions are checked by the compiler and must be either caught or declared to be thrown. Checked exceptions are subclasses of theException
class, which is itself a subclass of the Throwable
class. Examples of checked exceptions include IOException
, SQLException
, and ClassNotFoundException
. If your code does not handle a checked exception, the program will terminate with a runtime error.
try {
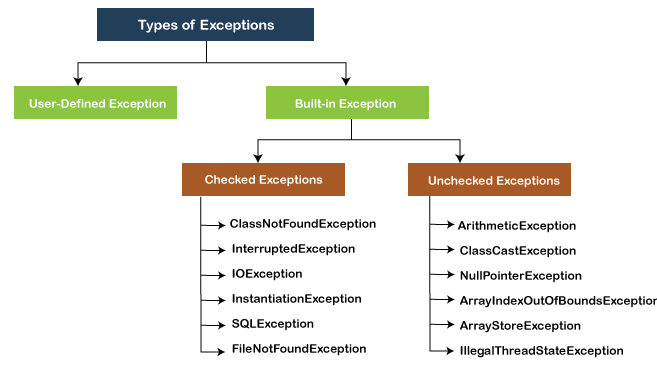
// code that may throw an IOException
} catch (IOException e) {
// handle the exception
}
Unchecked Exceptions: These exceptions are not checked by the compiler and do not need to be declared to be thrown. Unchecked exceptions are subclasses of the RuntimeException
class, which is itself a subclass of the Exception
class. Examples of unchecked exceptions include ArithmeticException
, ArrayIndexOutOfBoundsException
, and NullPointerException
. Since these exceptions do not need to be explicitly caught or declared, they will only terminate the program if left unhandled.
try {
// code that may throw an ArithmeticException
} catch (ArithmeticException e) {
// handle the exception
}
Error Exceptions: These exceptions are also not checked by the compiler and do not need to be declared to be thrown. Error exceptions are subclasses of the Error
class, which is itself a subclass of the Throwable
class. Examples of error exceptions include OutOfMemoryError
, StackOverflowError
, and ThreadDeath
. Like unchecked exceptions, these exceptions will only terminate the program if left unhandled.
try {
// code that may throw an OutOfMemoryError
} catch (OutOfMemoryError e) {
// handle the exception
}
It is worth noting that in Java, you can also create your own custom exceptions by extending either the Exception
class for checked exceptions or the RuntimeException
class for unchecked exceptions. This allows you to define specific error conditions and handling procedures for your application.
Handling exceptions correctly can be crucial in a robust software development process. It ensures that your code is more fault-tolerant, easy to debug, and better suited for real-world use cases where unexpected events may occur.
What is the hierarchy of Java?
I'd be happy to explain the hierarchy of Java!
In the context of object-oriented programming (OOP), the hierarchy of Java refers to the relationships between different types of objects and classes. Here's a breakdown of the hierarchy:
Class: A class is the fundamental unit of code organization in Java. It defines a set of attributes (data members) and methods that describe an object or concept. For example, you could create a "Car" class with attributes like color, model, and speed, as well as methods like startEngine() and accelerate(). Object: An object is an instance of a class. It has its own set of attribute values and can invoke methods defined by its class. In our Car example, you could create multiple objects (e.g., "myCar," "yourCar") that share the same attributes and methods. Interface: An interface is a abstract class that defines a contract or a protocol for how classes should behave. It declares methods without providing any implementation. Think of it as a set of instructions or a blueprint for objects to follow.Here's an example of how you might define an interface and implement it:
public interface Printable {
void print();
}
public class Document implements Printable {
public void print() {
System.out.println("Printing a document...");
}
}
In this example, the Printable
interface defines the method print()
, which must be implemented by any class that claims to be printable (like our Document
class).
For instance, you could create an abstract Animal
class with methods like eat(), sleep(), and move(), which would then be inherited by more specific classes like Dog
, Cat
, and Bird
.
Using our previous examples, you could create concrete classes like Poodle
(extending Dog
, which extends Animal
) or Parrot
(implementing Bird
, which implements Animal
).
For example, you could have a Car
class that contains a Engine
object (a composition), which is itself an instance of the InternalCombustionEngine
class. This allows for more flexibility and modularity in your design.
By understanding the hierarchy of Java classes, interfaces, and objects, you can create more robust, maintainable, and scalable software systems. Remember: each layer builds upon the previous one, allowing you to reuse code, inherit behaviors, and define complex relationships between entities!