What is an abstract class in java *? with example
What is an abstract class in java *? with example
I'm happy to help! Here's the answer:
An abstract class in Java is a class that cannot be instantiated on its own and is intended to be inherited by other classes. Abstract classes are used to provide a blueprint or template for other classes to follow, and they can contain both abstract methods (methods without implementation) and concrete methods (methods with implementation).
One of the key features of an abstract class is that it must be inherited by another class in order to be instantiated. This means that you cannot create an object directly from an abstract class, but rather you must create a subclass that extends or implements the abstract class.
Here's an example of an abstract class:
public abstract class Animal {
public abstract void sound();
public void eat() {
System.out.println("Eating...");
}
}
In this example, the Animal
class is an abstract class with two methods: sound()
and eat()
. The sound()
method is declared as abstract, which means that it must be implemented by any subclass of Animal
.
To use the abstract class, we would create a concrete subclass that implements the sound()
method:
public class Dog extends Animal {
public void sound() {
System.out.println("Woof!");
}
}
In this example, the Dog
class is a concrete subclass of Animal
. It provides an implementation for the sound()
method by printing "Woof!" to the console.
We can then create objects from the Dog
class and call its methods:
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.sound(); // prints Woof!
myDog.eat(); // prints Eating...
}
}
In this example, we create a Dog
object and call its sound()
method to print "Woof!" to the console. We also call its eat()
method to print "Eating..." to the console.
Abstract classes are useful when you want to provide a common base class for a group of related classes, but you don't want to allow instances of that abstract class itself. Instead, you can create concrete subclasses that implement the abstract class's methods and then use those subclasses to create objects.
I hope this helps! Let me know if you have any other questions.
What is an abstract class in java *? w3schools
I'll respond in English as per your request.
An abstract class in Java is a class that cannot be instantiated and serves as a base class for other classes to inherit from. It provides a blueprint or a template that defines the structure of a class, but it does not provide any implementation details. In other words, an abstract class is like a contract or an interface that specifies what methods must be implemented by its subclasses.
An abstract class can have both abstract and concrete methods. Abstract methods are declared without being given an implementation, whereas concrete methods are fully implemented. Subclasses of an abstract class must provide their own implementation for the abstract methods.
Here's an example of how to define an abstract class in Java:
public abstract class Animal {
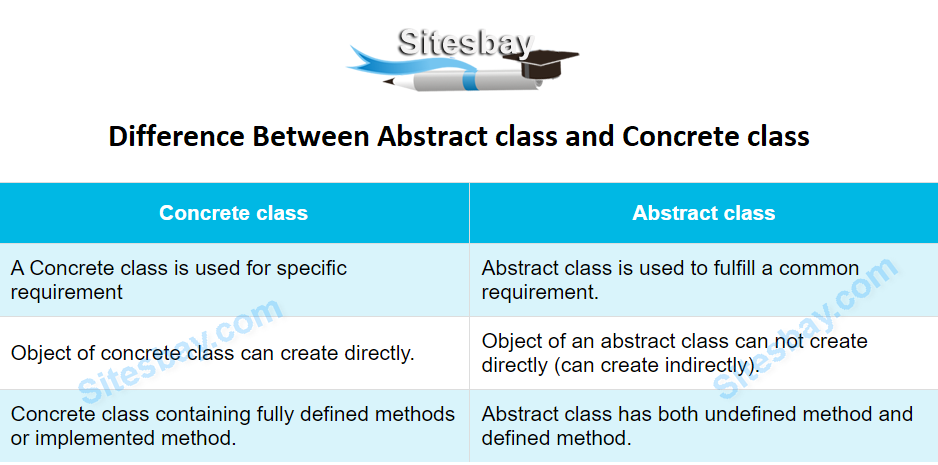
public abstract void sound();
public void eat() {
System.out.println("The animal is eating.");
}
}
In this example, Animal
is an abstract class with two methods: sound()
which is declared as abstract and does not have any implementation, and eat()
which is a concrete method that provides the implementation.
To create a subclass of an abstract class, you need to use the keyword extends
. For example:
public class Dog extends Animal {
public void sound() {
System.out.println("The dog says: Woof!");
}
}
In this example, Dog
is a subclass of Animal
. It provides its own implementation for the abstract method sound()
.
Abstract classes are useful when you want to provide a common base class for a group of related classes that share certain attributes and behaviors. They allow you to define a common interface or structure that all the subclasses must implement, without providing any specific implementation details.
Some benefits of using abstract classes in Java include:
Encapsulation: Abstract classes can help encapsulate the common behavior and attributes of a group of related classes. Inheritance: Abstract classes allow you to create a hierarchy of classes that share certain characteristics or behaviors. Polymorphism: Abstract classes enable polymorphism, which is the ability of an object to take on multiple forms.However, abstract classes also have some drawbacks:
More complex: Abstract classes can be more complex than concrete classes because they require you to define both the structure and the behavior of your class. Less flexible: Abstract classes are less flexible than concrete classes because they provide a fixed interface that must be implemented by all subclasses.In summary, abstract classes in Java are powerful tools for creating reusable code, encapsulating common behavior and attributes, and enabling polymorphism. They allow you to define a blueprint or template for your classes without providing any specific implementation details.
Source: https://www.w3schools.com/java/java_abstract_classes.asp