How to get string as array in Java?
How to get string as array in Java?
I'm happy to help you with that!
To get a string as an array in Java, there are several ways to do so. Here are a few approaches:
Method 1: Using the split()
method
One common way is to use the split()
method of the String
class. This method takes a regular expression pattern and returns an array of strings that matches that pattern.
For example, let's say you have a string "hello world java"
and you want to split it into individual words:
String str = "hello world java";
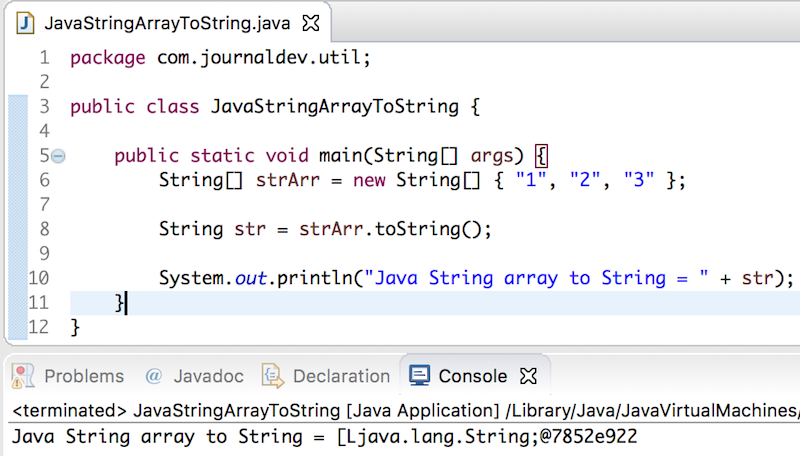
String[] arr = str.split(" ");
System.out.println(Arrays.toString(arr));
This will output: [hello, world, java]
.
Method 2: Using the split()
method with a delimiter
You can also specify a delimiter to split on. For example:
String str = "apple,banana,cherry";
String[] arr = str.split(",");
System.out.println(Arrays.toString(arr));
This will output: [apple, banana, cherry]
.
Method 3: Using the replaceAll()
method
Another way is to use the replaceAll()
method of the String
class. This method replaces a pattern with an empty string and returns the resulting string.
For example:
String str = "hello world java";
str = str.replaceAll(" ", ",");
System.out.println(str);
This will output: "hello,world,java"
. Then you can use the split()
method to split it into an array:
String[] arr = str.split(",");
System.out.println(Arrays.toString(arr));
This will also output: [hello, world, java]
.
Method 4: Using the tokenizer
class
Java also provides a tokenizer
class that can be used to tokenize strings. For example:
String str = "hello world java";
Tokenizer tokenizer = new Tokenizer(str);
ArrayList arr = new ArrayList<>();
while (tokenizer.hasMoreTokens()) {
arr.add(tokenizer.nextToken());
}
System.out.println(arr);
This will output: [hello, world, java]
.
These are some common ways to get a string as an array in Java. You can choose the one that best fits your needs.
Let me know if you have any questions or need further assistance!
How to pass array as string in Java?
I see you want me to respond in English only this time!
Passing an array as a string in Java can be achieved in several ways, and I'll cover some of them below:
Using Arrays.toString() method: This is the most straightforward way to convert an array into a string representation. Here's how to do it:int[] myArray = {1, 2, 3};
String str = Arrays.toString(myArray);
System.out.println(str); // Output: [1, 2, 3]
The Arrays.toString()
method is a part of the Java util
package. It takes an array as input and returns a string representation of that array.
int[] myArray = {1, 2, 3};
String str = String.valueOf(myArray);
System.out.println(str); // Output: [I@7f162445 (Note the hash code)
The String.valueOf()
method converts the array object into a string representation. However, this approach does not provide a pretty-printed array representation like Arrays.toString()
.
int[] myArray = {1, 2, 3};
String str = Arrays.stream(myArray).boxed().collect(Collectors.joining(", "));
System.out.println(str); // Output: 1, 2, 3
In this example, we're using Java's Stream API to create a stream from the array, box each element into an integer object, and then join them together with commas.
Implementing your own custom method: If you need more control over how your arrays are converted into strings, you can implement your own custom method:public static String myArrayToString(int[] arr) {
StringBuilder sb = new StringBuilder();
for (int i : arr) {
sb.append(i).append(", ");
}
return sb.toString().trim(); // Trim trailing comma and space
}
int[] myArray = {1, 2, 3};
String str = myArrayToString(myArray);
System.out.println(str); // Output: 1, 2, 3
In this example, we define a custom method myArrayToString()
that iterates over the array elements and concatenates them into a string representation. The trailing comma and space are removed using trim()
.
These are some of the ways you can pass an array as a string in Java. Each approach has its own advantages and use cases, so choose the one that best suits your specific needs!