Java serialization w3schools javatpoint example
Java serialization w3schools javatpoint example
Here is an explanation of Java serialization with examples from W3Schools and JavatPoint:
Java Serialization:
Serialization is the process of converting an object's state into a byte stream. It allows you to save an object's state, so it can be re-created later. This is useful when you need to store or transmit an object's state.
Here is an example from W3Schools:
import java.io.*;
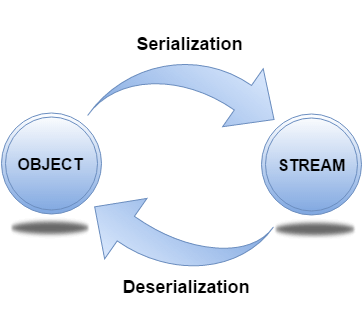
class Person implements Serializable {
private String name;
private int age;
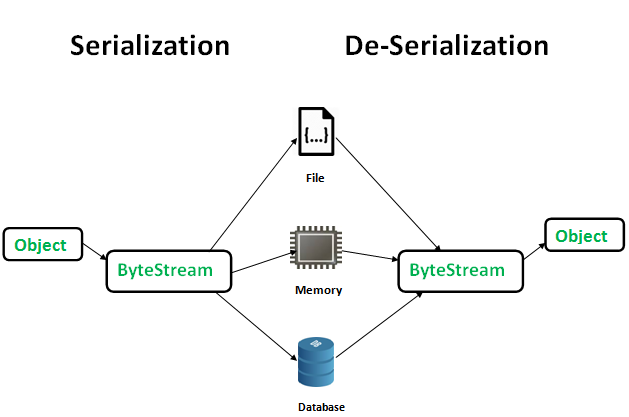
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
public class Main {
public static void main(String[] args) throws IOException {
Person person = new Person("John", 30);
// Serialize the object
FileOutputStream fos = new FileOutputStream("person.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(person);
oos.close();
fos.close();
// Deserialize the object
FileInputStream fis = new FileInputStream("person.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
Person deserializedPerson = (Person) ois.readObject();
ois.close();
fis.close();
System.out.println(deserializedPerson.getName() + " is " + deserializedPerson.getAge() + " years old.");
}
}
In this example, the Person
class implements the Serializable
interface. This means it can be serialized and deserialized. The main
method creates a Person
object, serializes it to a file called "person.ser", then deserializes the object back from the file.
Here is an example from JavatPoint:
import java.io.*;
public class Employee implements Serializable {
private int empId;
private String empName;
public Employee(int id, String name) {
this.empId = id;
this.empName = name;
}
}
public class Main {
public static void main(String args[]) throws IOException {
// Create an instance of Employee
Employee emp1 = new Employee(12345, "John Doe");
// Serialize the object
FileOutputStream fos = new FileOutputStream("employee.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(emp1);
oos.close();
fos.close();
// Deserialize the object
FileInputStream fis = new FileInputStream("employee.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
Employee emp2 = (Employee)ois.readObject();
ois.close();
fis.close();
System.out.println("The ID of deserialized employee is: " + emp2.empId +
" and name is: " + emp2.empName);
}
}
In this example, the Employee
class also implements the Serializable
interface. The main
method creates an Employee
object, serializes it to a file called "employee.ser", then deserializes the object back from the file.
Java provides two classes for serialization: ObjectOutputStream
and ObjectInputStream
. These classes are used for writing (serialization) and reading (deserialization) objects, respectively.
Note that the Serializable
interface has no methods. It's a marker interface that tells Java that an object can be serialized.
What is Java serialization?
I'll respond in English this time!
Java Serialization is a mechanism that allows an object's state to be converted into a byte stream, which can then be written to a file or transmitted over a network connection. This process is also known as marshalling. The serialized form of the object can be deserialized later, allowing the original object's state to be recreated.
In Java, serialization is supported by implementing the Serializable
interface in the class that needs to be serialized. Any class that implements this interface must provide a no-argument constructor (also known as the default constructor). This constructor is responsible for initializing the object's state before it is serialized.
Here are some key aspects of Java Serialization:
Serialization: The process of converting an object's state into a byte stream. Deserialization: The process of reconstructing an original object from its serialized form. Marshalling: A more general term for the process of serializing or deserializing data. Unmarshalling: Another name for deserialization.Java Serialization uses the writeObject()
and readObject()
methods provided by the ObjectOutputStream
and ObjectInputStream
classes, respectively. When an object is serialized, its state is written to a byte stream using these methods. Conversely, when an object is deserialized, its state is reconstructed from the byte stream using the same methods.
Java Serialization has several benefits:
Persistence: Serialized objects can be persisted across system restarts or network connections. Data transfer: Serialized objects can be easily transferred between systems over a network connection. State preservation: Serialized objects preserve their state, allowing them to be reconstructed exactly as they were before serialization.However, Java Serialization also has some limitations:
Performance overhead: The serialization process can introduce performance overhead, especially for large objects or complex graphs of objects. Security concerns: Serialized objects can pose security risks if not handled properly, as they may contain sensitive information (e.g., passwords, credit card numbers). Versioning issues: Changes to the serialized object's structure can lead to versioning issues and make it difficult to deserialize older versions of the object.In summary, Java Serialization provides a convenient mechanism for converting objects into a byte stream that can be written to a file or transmitted over a network connection. While it has its benefits and limitations, it remains an essential tool in Java programming, enabling developers to persist and transfer data between systems.