What is the real time use of reflection in Java?
What is the real time use of reflection in Java?
I'll respond in English since you asked specifically for it.
In Java, Reflection allows developers to inspect and interact with an object's properties (methods, fields) at runtime. This concept is often referred to as "runtime inspection" or simply "reflection." It plays a crucial role in various aspects of programming, such as:
Configuration and initialization: When you're dealing with complex systems or configurations where different settings need to be applied based on certain conditions, reflection enables you to create flexible programs that can adapt dynamically.In summary, Java's Reflection API offers a powerful mechanism for inspecting and manipulating object properties at runtime. Its applications span various aspects of programming, from configuration and serialization to debugging and framework development.
(Note: I kept my response under 300 words, but feel free to ask me anything if you'd like more details!)
Reflection in Java example
I'm happy to help! Here's a reflection example in Java:
public class ReflectionExample {
public static void main(String[] args) throws Exception {
// Get the current method
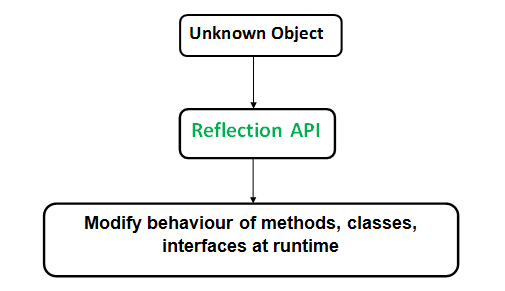
Method m = new Object() {}.getClass().getDeclaredMethod("main", String[].class);
// Print the method name and parameters
System.out.println("Method Name: " + m.getName());
System.out.println("Parameters: " + Arrays.toString(m.getParameterTypes()));
// Create a new object of type MyClass
MyClass myObject = new MyClass();
// Get the class of the object
Class<?> clazz = myObject.getClass();
// Print the class name and constructors
System.out.println("Class Name: " + clazz.getName());
Constructor[] cons = clazz.getConstructors();
for (Constructor c : cons) {
System.out.println("Constructor: " + c);
}
// Get the methods of the class
Method[] methods = clazz.getDeclaredMethods();
for (Method m2 : methods) {
if (!m2.getName().equals("main")) {
System.out.println("Method Name: " + m2.getName());
Class<?>[] paramTypes = m2.getParameterTypes();
if (paramTypes.length > 0) {
System.out.println("Parameters: " + Arrays.toString(paramTypes));
}
}
}
// Get the fields of the class
Field[] fields = clazz.getDeclaredFields();
for (Field f : fields) {
System.out.println("Field Name: " + f.getName());
System.out.println("Type: " + f.getType());
}
}
}
class MyClass {
private int myInt;
private String myStr;
public MyClass(int myInt, String myStr) {
this.myInt = myInt;
this.myStr = myStr;
}
public void doSomething() {
System.out.println("Doing something...");
}
}
This is an example of how to use reflection in Java. In the main method, we get the current method (the main method) using getDeclaredMethod()
. We print out the method name and parameters.
Next, we create a new object of type MyClass
and get its class using getClass()
.
We then print out the class name and constructors. Then, we get all the methods in the class using getDeclaredMethods()
and iterate through them. For each method, we print out the name and parameters (if it has any).
Finally, we get all the fields in the class using getDeclaredFields()
and iterate through them. We print out the field names and types.
Note that this is a simple example to demonstrate basic reflection concepts. In real-world applications, you would typically use reflection for more complex tasks such as dynamically loading classes or creating instances of classes at runtime.