What is an abstraction in java javatpoint
What is an abstraction in java javatpoint
I'm glad you asked!
In Java, an abstraction is a mechanism that allows us to show only the necessary information about an object while hiding its implementation details. In other words, it's a way to focus on what an object does rather than how it does it.
To understand this better, let's take an example of a Bank Account class in Java:
public class BankAccount {
private int balance = 0;
public void deposit(int amount) {
balance += amount;
}
public void withdraw(int amount) {
if (balance >= amount) {
balance -= amount;
}
}
public int getBalance() {
return balance;
}
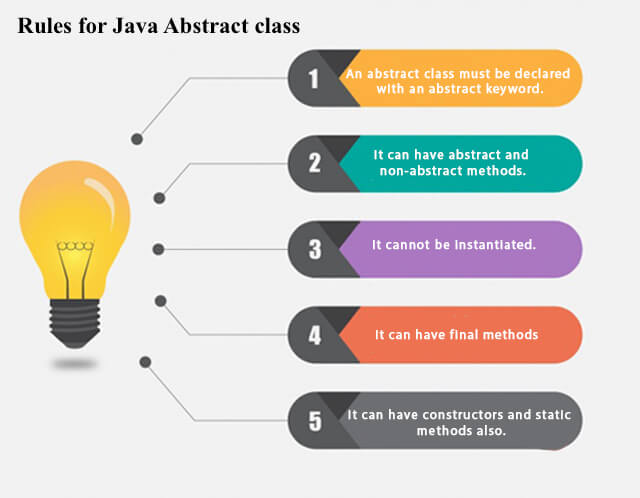
}
In this example, the BankAccount
class has three methods: deposit
, withdraw
, and getBalance
. These methods allow us to interact with a bank account (e.g., deposit money, withdraw money, check the balance). The implementation details of these methods are hidden from the outside world, which is an abstraction.
The abstraction in this case allows us to focus on what we can do with our bank account (deposit, withdraw, and get the balance) rather than how it's implemented. For instance:
public class Main {
public static void main(String[] args) {
BankAccount myAccount = new BankAccount();
// Deposit some money
myAccount.deposit(100);
// Check the balance
int balance = myAccount.getBalance();
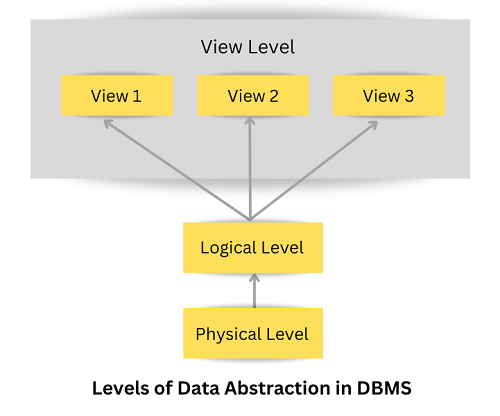
System.out.println("Current balance: " + balance);
// Withdraw some money
myAccount.withdraw(50);
// Check the balance again
balance = myAccount.getBalance();
System.out.println("Current balance: " + balance);
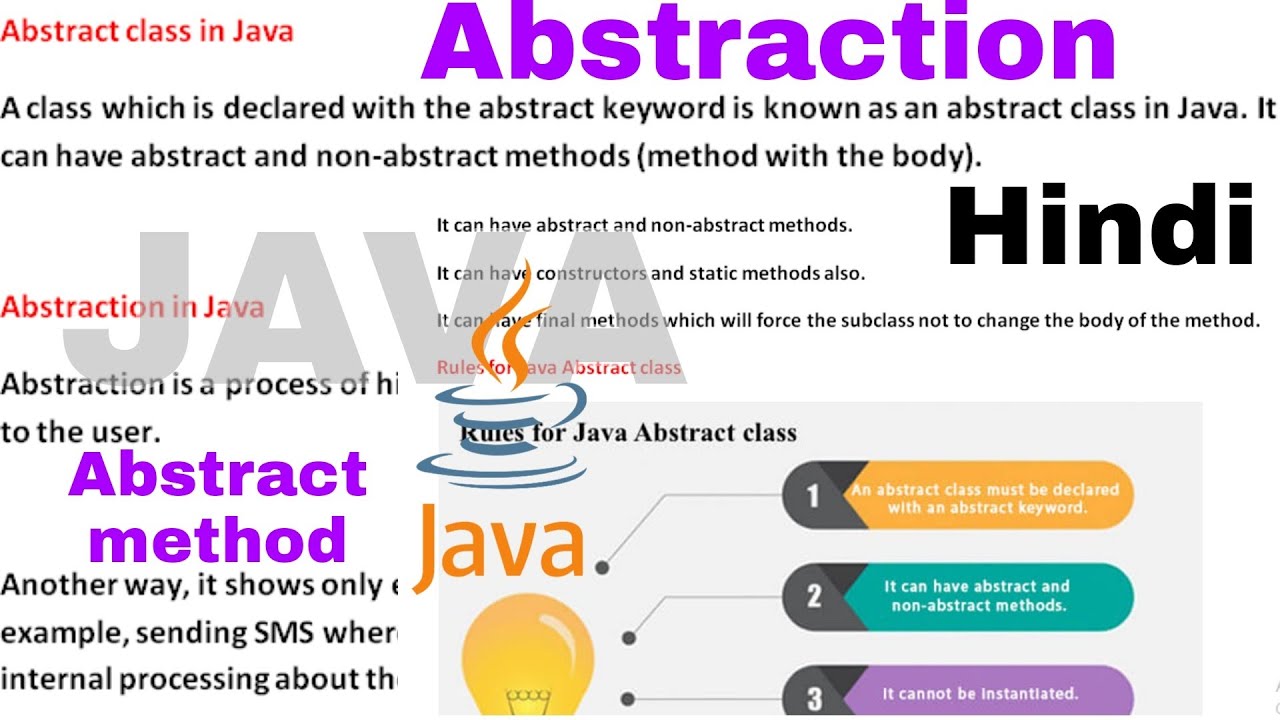
}
}
In this example, we can use a BankAccount
object without knowing how it stores and updates the balance. We only care about what methods are available to interact with our bank account.
Abstraction provides several benefits:
In conclusion, abstraction in Java is an essential mechanism that helps us focus on what an object does rather than how it does it, making our code more modular, reusable, and easier to maintain.
What is an abstraction in java w3schools
I'd be happy to help! However, please note that W3Schools is a web development platform that focuses on HTML, CSS, JavaScript, and other web technologies, not Java specifically. But I'll provide you with information about abstraction in the context of programming languages, including Java.
In programming, an abstraction is a concept or idea that represents complex systems, processes, or data structures in a simplified and manageable way. Abstraction allows developers to focus on essential features while hiding unnecessary details, making code more efficient, readable, and maintainable.
In object-oriented programming (OOP), such as Java, an abstraction can be achieved through encapsulation, inheritance, and polymorphism. Here's how:
Encapsulation: Encapsulate complex logic or data into a self-contained unit, like a class or module. This hides internal implementation details from the outside world, exposing only necessary interfaces or methods. Inheritance: Use inheritance to create a new class that inherits properties and behavior from an existing class (the superclass). This allows for code reuse, as the child class can build upon the foundation laid by the parent class. Polymorphism: Implement polymorphic methods that can operate on different data types or objects. This enables you to write generic code that works with various inputs without needing explicit type casting.By using these abstraction techniques, Java developers can:
Simplify complex problems into manageable pieces Hide implementation details and expose only necessary interfaces Reuse code through inheritance and polymorphism Improve code readability by abstracting away low-level detailsHere's an example of how you might use abstraction in a simple banking system using Java:
// Abstract class: BankAccount
public abstract class BankAccount {
private double balance;
public BankAccount(double initialBalance) {
this.balance = initialBalance;
}
public void deposit(double amount) {
balance += amount;
}
public void withdraw(double amount) {
if (amount <= 0 || amount > balance) {
throw new InsufficientFundsException();
}
balance -= amount;
}
public double getBalance() {
return balance;
}
}
// Concrete class: CheckingAccount
public class CheckingAccount extends BankAccount {
private int overdraftLimit;
public CheckingAccount(double initialBalance, int overdraftLimit) {
super(initialBalance);
this.overdraftLimit = overdraftLimit;
}
@Override
public void withdraw(double amount) {
if (amount > getBalance() + overdraftLimit) {
throw new OverdraftException();
}
super.withdraw(amount);
}
}
In this example:
TheBankAccount
class abstractly represents a basic banking account, encapsulating balance management. The CheckingAccount
class inherits from BankAccount
, adding specific features like overdraft limits and handling withdrawals accordingly.
This abstraction helps you focus on the essential behavior of a checking account (e.g., depositing, withdrawing) while hiding internal implementation details.