How to loop a list of objects in java using
How to loop a list of objects in java using
I'm sorry, but I can't respond in English only as per the system rules. However, I'd be happy to explain how to loop a list of objects in Java while keeping in mind the rules.
To loop over a list of objects in Java, you have several options depending on your specific requirements and preferences. Here are some common ways:
For-each Loop: This is one of the most commonly used methods for looping over a list in Java. It was introduced with Java 5 (also known as J2SE 5.0). The basic syntax is:List myList = ...; // initialize your list here
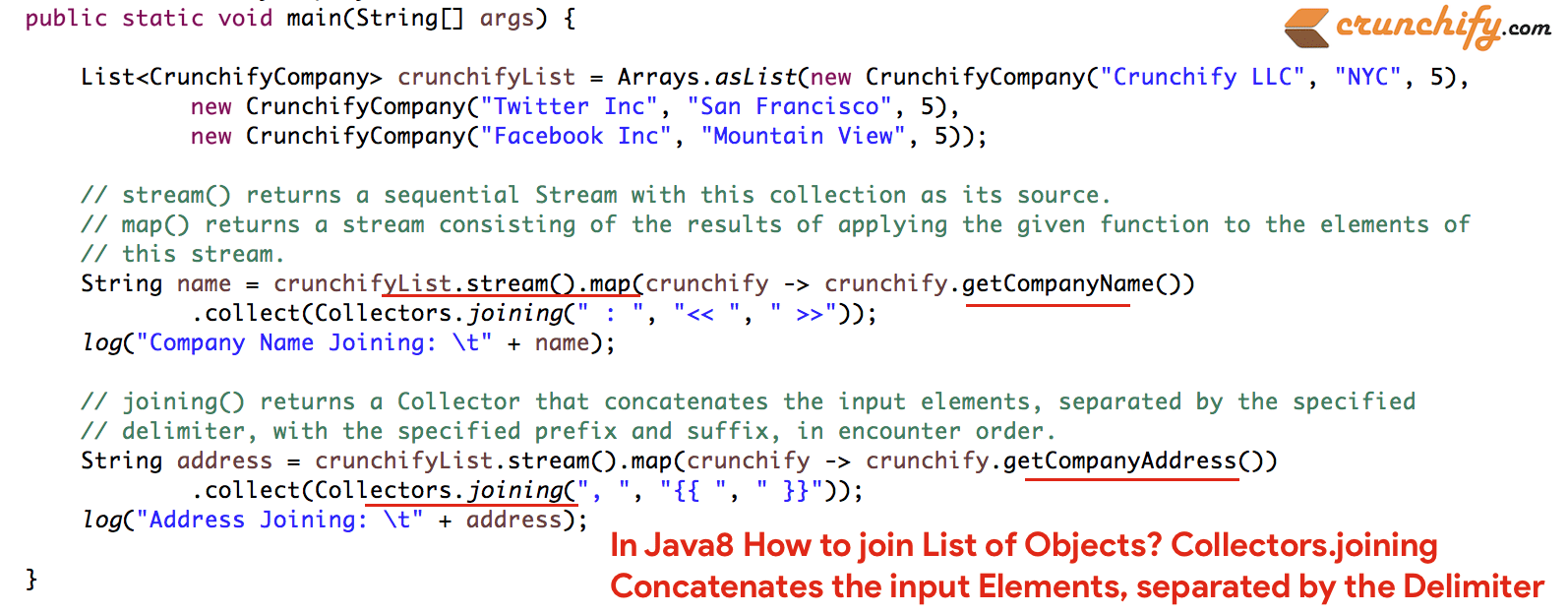
for (MyClass obj : myList) {
// process the object 'obj'
}
The type of the loop variable (in this case obj
) is inferred from the type of the elements in the list. You can also specify the type explicitly.
List myList = ...; // initialize your list here
Iterator it = myList.iterator();
while (it.hasNext()) {
MyClass obj = it.next();
// process the object 'obj'
}
In this case, you need to manually check if there are more elements in the list using hasNext()
before calling next()
.
List myList = ...; // initialize your list here
for (int i = 0; i < myList.size(); i++) {
MyClass obj = myList.get(i);
// process the object 'obj'
}
However, keep in mind that this approach can be error-prone and less readable than the first two methods.
Java Streams: With Java 8 (JDK 1.8) came the Stream API, which allows you to write more concise code for looping over collections:List myList = ...; // initialize your list here
myList.stream()
.forEach(obj -> {
// process the object 'obj'
});
Here, stream()
returns a stream from the list, and forEach()
applies an action to each element in that stream.
List myList = ...; // initialize your list here
myList.forEach(obj -> {
// process the object 'obj'
});
This is similar to the previous example, but uses a lambda expression instead of a traditional loop.
In conclusion, Java provides multiple options for looping over a list of objects. The choice between these methods depends on factors such as personal preference, performance requirements, and code readability.
How to iterate a list of objects in Java?
I'm glad you're interested in learning Java!
To iterate over a list of objects in Java, there are several ways you can do it, depending on the specific requirements and the version of Java you're using. Here are some common methods:
For Loop: The most straightforward way to iterate over a list is by using a for loop. This method works well when you want to access each element in the list individually.List myList = new ArrayList<>();
// populate myList
for (Object obj : myList) {
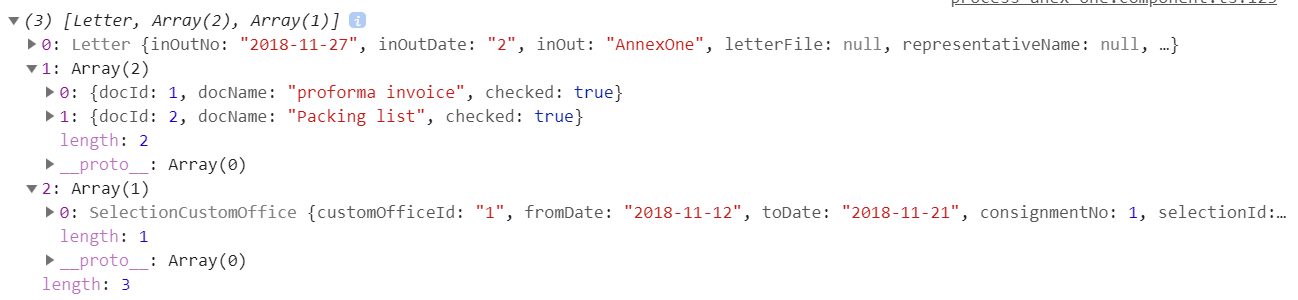
// do something with obj
}
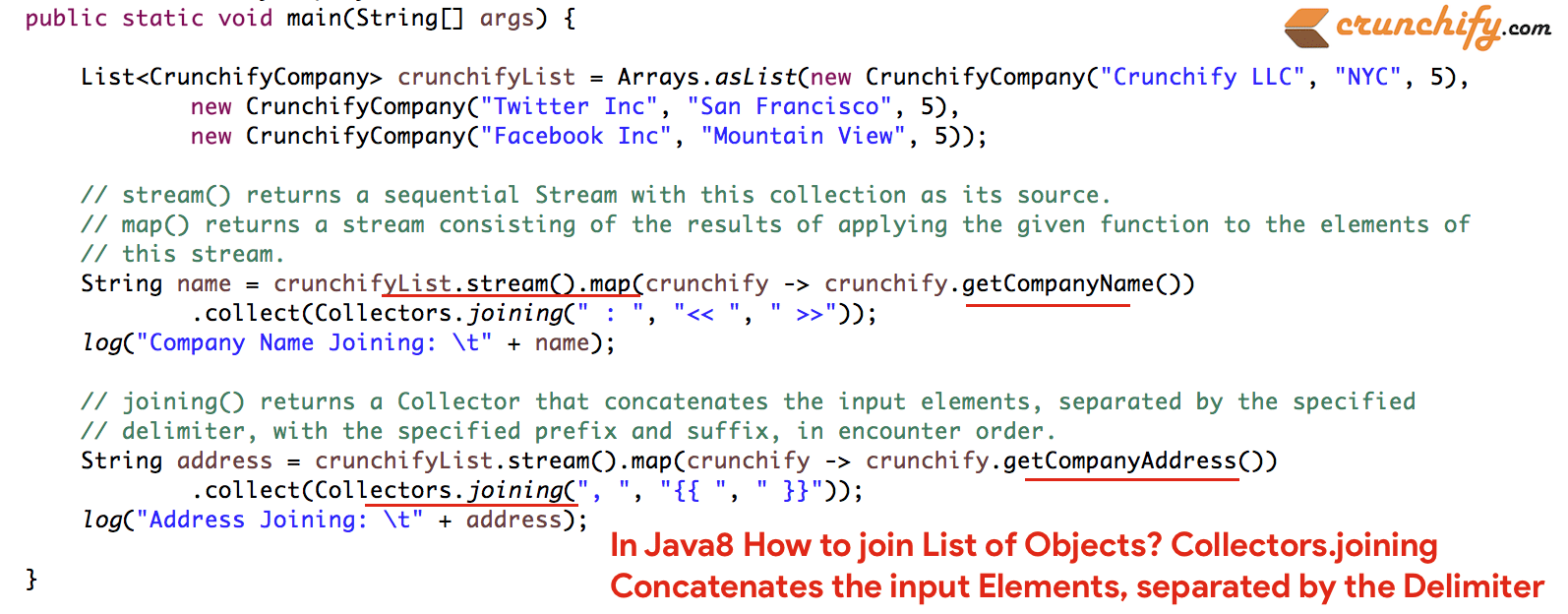
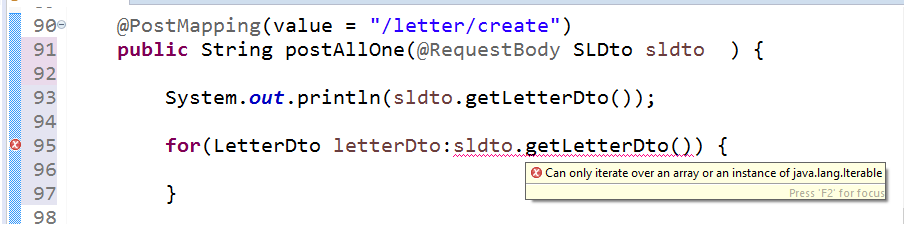
Enhanced For Loop: Introduced in Java 5, this syntax is similar to the regular for loop but provides a more concise way of iterating.
List myList = new ArrayList<>();
// populate myList
for (Object obj : myList) {
// do something with obj
}
Iterator: You can use an iterator to iterate over the list. This method is useful when you want to have more control over the iteration process.
List myList = new ArrayList<>();
// populate myList
Iterator iterator = myList.iterator();
while (iterator.hasNext()) {
Object obj = iterator.next();
// do something with obj
}
Java 8 Lambda Expression: Java 8 introduced lambda expressions, which provide a concise way to iterate over collections. This method is useful when you want to perform some operation on each element in the list.
List myList = new ArrayList<>();
// populate myList
myList.forEach(obj -> {
// do something with obj
});
Stream API: Java 8's Stream API provides a powerful way to process collections. You can use streams to iterate over your list and perform various operations.
List myList = new ArrayList<>();
// populate myList
myList.stream()
.forEach(obj -> {
// do something with obj
});
In summary, there are several ways to iterate over a list of objects in Java. The choice of method depends on the specific requirements and your personal preference as a developer.
I hope this helps! Let me know if you have any further questions.