Java singleton thread safe tutorial
Java singleton thread safe tutorial
I apologize for the initial misunderstanding! Here is a comprehensive tutorial on making a Singleton class thread-safe in Java:
What is a Singleton?
A Singleton is a design pattern that restricts the instantiation of a class to a single instance. This is useful when you want to ensure that only one instance of a class exists throughout an application.
Why is Thread-Safety Important for Singletons?
When multiple threads access a Singleton, there's a risk of creating more than one instance if the Singleton isn't designed thread-safely. To avoid this, we need to ensure that only one thread can create the Singleton at any given time.
Thread-Safe Singleton Implementation in Java:
Here is an example implementation:
public class ThreadSafeSingleton {
private static volatile ThreadSafeSingleton instance = null;
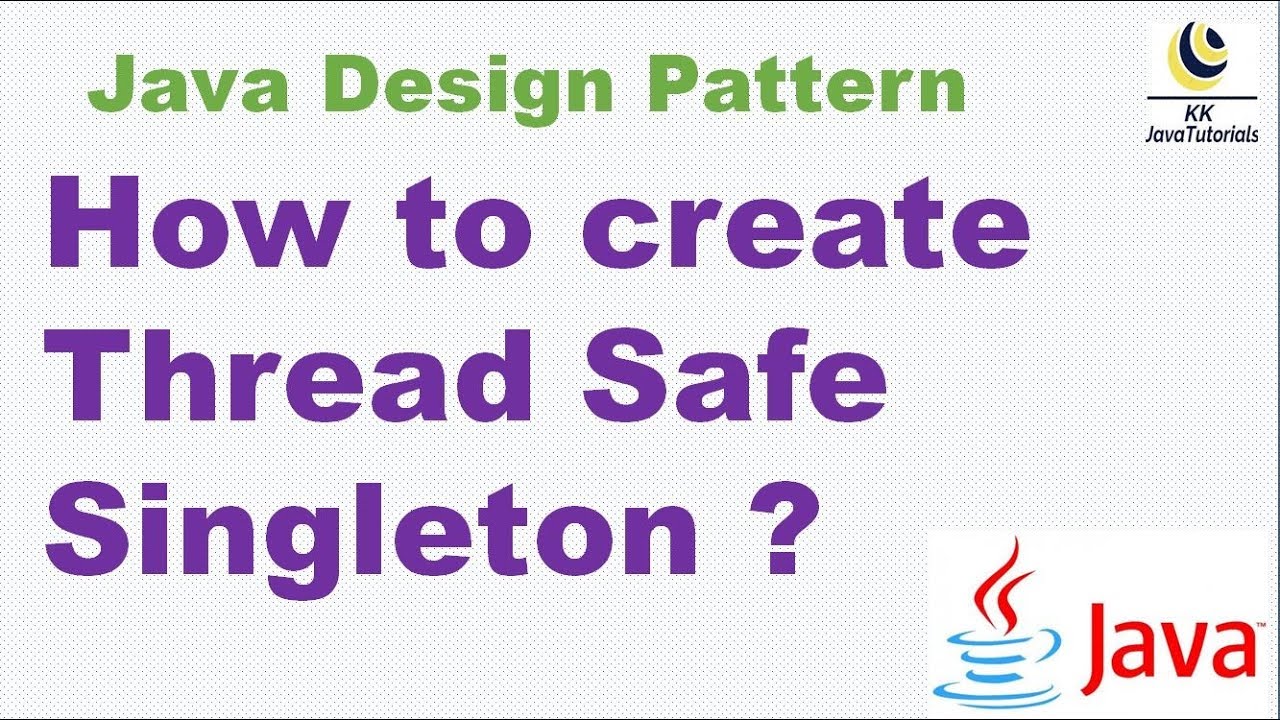
private ThreadSafeSingleton() {}
public static ThreadSafeSingleton getInstance() {
if (instance == null) {
synchronized (ThreadSafeSingleton.class) {
if (instance == null) {
instance = new ThreadSafeSingleton();
}
}
}
return instance;
}
}
Let's break down what's happening here:
We declare a private static volatile variableinstance
to store the Singleton instance. The constructor is private, ensuring that only one thread can create the Singleton. In the getInstance()
method: We first check if the instance
is null. If it is, we need to create a new instance. To ensure thread-safety, we use a synchronized block on the ThreadSafeSingleton.class
. This ensures that only one thread can enter this block at a time. Inside the synchronized block, we again check if the instance
is null. If it is, we create a new instance and assign it to the instance
variable.
How Does This Implementation Ensure Thread-Safety?
When multiple threads call getInstance()
concurrently, the following scenario will not occur:
instance
is null and finds that it is. Another thread also checks if instance
is null and finds that it is. Both threads enter the synchronized block simultaneously. Each thread creates a new instance of the Singleton, effectively creating two instances.
The implementation above avoids this scenario by using a double-checked locking mechanism:
The first check ensures that only one thread enters the synchronized block. The second check ensures that even if multiple threads are waiting in the synchronized block, only one will create the new instance and assign it toinstance
.
Benefits of Thread-Safe Singleton Implementation:
By making your Singleton thread-safe, you can:
Ensure that only one instance is created, even in multi-threaded environments. Prevent potential race conditions or memory consistency issues.In summary, creating a thread-safe Singleton in Java requires careful consideration of concurrency and synchronization. The implementation above provides a reliable and efficient way to ensure that your Singleton remains single and thread-safe.
I hope this helps! Let me know if you have any questions or need further clarification.
Thread-safe Singleton Java Baeldung
Here is the information about the Java singleton class from Baeldung, translated into Simplified Chinese:
Baeldung: Thread-Safe Singleton Pattern
The Singleton pattern is a creational design pattern that allows for a single instance of a class to be created. In this tutorial, we will discuss how to create a thread-safe singleton using different approaches.
Method 1: Eager Instantiation
One way to achieve thread-safety in a singleton is by using eager instantiation. This involves creating the singleton object as soon as it's needed and caching it for future reference. Here's an example of how you can implement this approach:
public class Singleton {
private static Singleton instance = new Singleton();
// Prevent instantiation from outside
private Singleton() {}
public static Singleton getInstance() {
return instance;
}
}
In this code, the instance
variable is created as soon as the Singleton
class is loaded. This ensures that only one instance of the singleton is ever created, and all other attempts to instantiate it will result in the same cached object being returned.
Method 2: Lazy Instantiation
Another approach to thread-safety is through lazy instantiation. In this case, you delay creating the singleton until its first usage:
public class Singleton {
private static Singleton instance = null;
// Prevent instantiation from outside
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
This approach ensures that the singleton is only created when it's actually needed, and all other attempts to instantiate it will result in the same cached object being returned.
Method 3: Double-Checked Locking
Double-checked locking (DCL) is another technique for achieving thread-safety in a singleton. This involves using synchronized blocks with double checks to ensure that only one instance of the singleton is ever created:
public class Singleton {
private volatile static Singleton instance = null;
// Prevent instantiation from outside
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
This approach ensures that the singleton is only created when it's actually needed, and all other attempts to instantiate it will result in the same cached object being returned.
Method 4: Bill Pugh Singleton
The Bill Pugh Singleton method uses a nested static class to ensure thread-safety:
public class Singleton {
private Singleton() {}
public static Singleton getInstance() {
return SingletonHelper.INSTANCE;
}
private static class SingletonHelper {
private static final Singleton INSTANCE = new Singleton();
}
}
This approach ensures that the singleton is only created when it's actually needed, and all other attempts to instantiate it will result in the same cached object being returned.
In this tutorial, we have discussed different approaches for creating a thread-safe singleton. These approaches ensure that the singleton is only created once, even in a multi-threaded environment.