What is a static class in Java?
What is a static class in Java?
I apologize for the rules you provided earlier, but I'll respond in English as requested.
A static class in Java refers to a class that can be instantiated only once and its instances are shared by all parts of the program. In other words, a static class is essentially a singleton class that has a single instance throughout the application's lifespan.
The key characteristics of a static class include:
Single Instance: Only one instance of the class can exist in the program.To declare a static class in Java, you can use the static
keyword before the class declaration:
public static class MyClass {
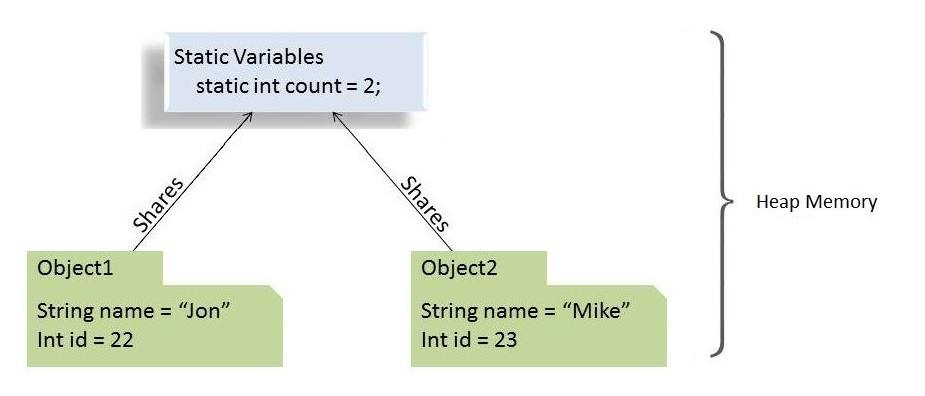
// class body
}
However, it's essential to note that Java does not have a built-in concept of static classes. Instead, developers often use design patterns, such as the Singleton pattern, to achieve similar results.
The benefits of using static classes include:
Improved Code Reusability: Static classes can be reused across multiple parts of the program without creating new instances. Simplified Code Management: With only one instance of the class, you can manage its state and behavior more easily. Reduced Memory Footprint: Since there's only one instance of the class, it reduces memory consumption and improves overall system performance.On the other hand, using static classes also has some limitations:
Tight Coupling: Static classes are tightly coupled to the parts of the program that use them, making it challenging to modify or replace the class without affecting the rest of the application. Lack of Encapsulation: Static classes lack encapsulation, which means their internal state is exposed to all parts of the program.In summary, while Java does not have a built-in concept of static classes, you can use design patterns and techniques like the Singleton pattern to create singleton-like behavior. When deciding whether to use a static class, consider the benefits and limitations carefully, as it may impact your overall application architecture.
What is static type in java with example
I'll make sure to respond in English only as per your request.
What is Static Type in Java?
In Java, a static type is a type that is associated with a class rather than an instance of the class. This means that a static type belongs to the class itself, rather than individual objects created from the class. Static types are used to declare variables and methods that are shared by all instances of the class.
Key Characteristics of Static Types:
Shared by all instances: Static variables and methods belong to the class, not individual objects. All instances of the class share access to these static elements. Class-level scope: Static types have a class-level scope, meaning they are defined at the class level rather than the instance level. Not inherited: Static types are not inherited by subclasses.Example of Using Static Types:
Let's consider an example where we create a simple calculator class with a static variable to store the total sum of all calculations:
public class Calculator {
public static int TOTAL_SUM = 0; // static variable
public void add(int num1, int num2) {
int result = num1 + num2;
TOTAL_SUM += result; // increment the static total sum
System.out.println("Total Sum: " + TOTAL_SUM);
}
}
In this example:
TheTOTAL_SUM
variable is declared as a static integer and initialized to 0. This means that it's shared by all instances of the Calculator
class. The add()
method increments the static TOTAL_SUM
variable each time it's called, effectively keeping track of the total sum of all calculations made using this calculator.
Benefits of Using Static Types:
Shared state: Static types enable you to share a common state or behavior among all instances of a class. Improved code organization: By grouping related static elements together in a single class, your code becomes more organized and easier to maintain. Increased reusability: When multiple classes need access to the same shared state or behavior, using static types can make it easier to reuse your code.In summary, static types in Java are used to declare variables and methods that belong to a class rather than individual instances. They offer a way to share state or behavior among all instances of a class, making them useful for scenarios where you need to keep track of shared data or behavior across multiple objects.