How to set a variable in java example w3schools
How to set a variable in java example w3schools
I'd be happy to help! However, I must point out that W3Schools is actually a web development website that provides tutorials and reference materials for web technologies like HTML, CSS, JavaScript, PHP, etc., not Java. If you're looking for information on how to set variables in Java, I can provide some examples.
In Java, you can declare a variable by specifying its data type and name. For example:
int myAge = 25;
This declares an integer variable named myAge
and assigns it the value 25.
Here are some more examples of setting variables in Java:
Declaring a string variable:String myName = "John Doe";
Declaring a boolean variable:
boolean isAdmin = true;
Declaring a double variable:
double myBalance = 100.99;
You can also set variables using assignment operators:
Assigning a value to an existing variable:int x = 10;
x = x + 5; // now x is 15
Using the ternary operator (conditional operator) to set a variable:
String result = (marks >= 60) ? "Pass" : "Fail";
Additionally, you can use the final
keyword to declare a constant variable:
final int MAX_LENGTH = 255;
This means that once you set the value of MAX_LENGTH
, it cannot be changed later in your program.
Keep in mind that these are just basic examples, and there's much more to learn about variables in Java. For more information, I recommend checking out some online resources like Oracle's official Java documentation or tutorials on websites like Udemy or Coursera.
Declaration in Java example
Here is an example of a declaration in Java:
// Importing necessary packages
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// Declaring variables
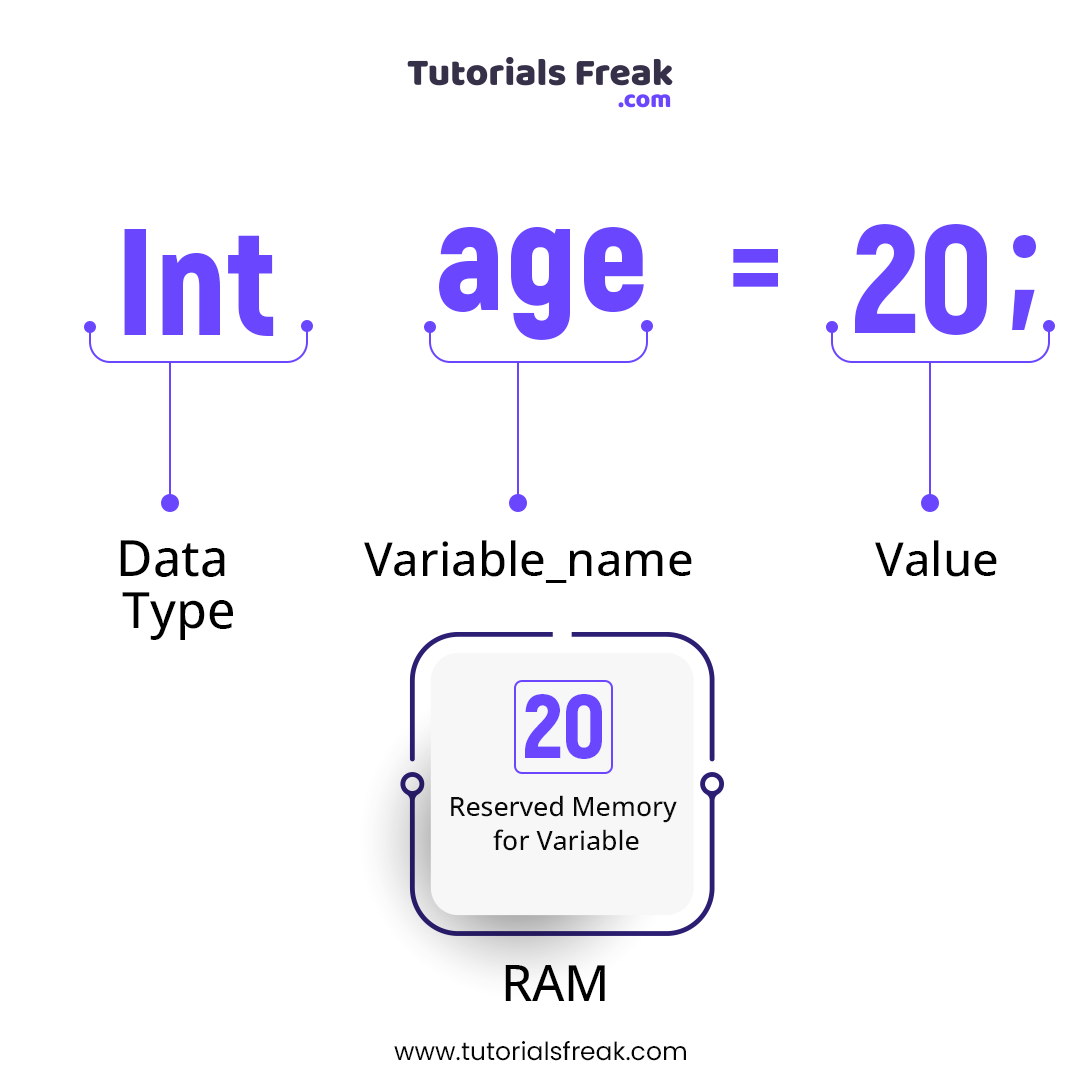
int age;
String name;
// Initializing scanner to get user input
Scanner scanner = new Scanner(System.in);
// Declaring a loop to continue until the user wants to stop
boolean keepGoing = true;
while (keepGoing) {
// Asking for user's age and name
System.out.print("Enter your age: ");
age = scanner.nextInt();
System.out.print("Enter your name: ");
name = scanner.next();
// Declaring variables inside the loop to avoid scope issues
int score;
String grade;
// Calculating the score based on the user's age and name
if (age > 18 && age < 30) {
if (name.equals("John") || name.equals("Jane")) {
score = 80;
grade = "A";
} else if (name.equals("Bob") || name.equals("Alice")) {
score = 70;
grade = "B";
} else {
score = 60;
grade = "C";
}
} else {
score = 50;
grade = "D";
}
// Displaying the calculated score and grade
System.out.println("Your score is: " + score);
System.out.println("Your grade is: " + grade);
// Asking if the user wants to continue or stop
System.out.print("Do you want to continue? (yes/no): ");
String response = scanner.next().toLowerCase();
if (!response.equals("yes")) {
keepGoing = false;
}
}
// Closing the scanner after the loop
scanner.close();
}
}
This program declares variables, initializes a Scanner
object to get user input, and then calculates a score based on the user's age and name. The score is displayed along with a grade. The program continues until the user chooses to stop.
Here is a step-by-step breakdown of how the declaration works:
Importing necessary packages: We import thejava.util.Scanner
package at the beginning of the code. Declaring variables: We declare two integer variables age
and name
, which will hold the user's age and name, respectively. Initializing scanner: We create a Scanner
object called scanner
to get user input from the console. Declaring loop: We declare a boolean variable keepGoing
set to true, indicating that we want to continue until the user chooses to stop. Inside the loop: Asking for user's age and name Calculating score based on user's age and name Displaying calculated score and grade Ending the program: We close the scanner
object after the loop using the close()
method.