How do you write a long string in Java?
How do you write a long string in Java?
I can respond in English if that's what the user wants. Writing a long string in Java involves using various methods and techniques to efficiently handle large amounts of text data. Here are some ways to achieve this:
Example:
StringBuilder builder = new StringBuilder();
builder.append("Hello");
builder.append(", ");
builder.append("World");
builder.append("! ");
String str = builder.toString();
StringBuffer Class: Another way to write a long string in Java is by using the StringBuffer class. This class extends the AbstractStringBuilder class and provides most of the functionality of StringBuilder but is synchronized.
Example:
StringBuffer sb = new StringBuffer("Hello");
sb.append(", ");
sb.append("World");
sb.append("! ");
String str = sb.toString();
String Class: Java's String class also provides methods that can be used to build a long string.
Example:
String s1 = "Hello";
String s2 = ", World";
String s3 = "! ";
String str = s1 + s2 + s3;
Concatenation Method: Java also allows for concatenating strings using the '+' operator.
Example:
int num = 10;
double decimal = 23.45;
String str = "Hello, this is a string with an integer: " + num +
" and a decimal value: " + decimal;
Char Array: If you have to deal with very large strings, using a char array can be more efficient than StringBuilder or StringBuffer.
Example:
char[] chars = new char[100];
String str = new String(chars);
In conclusion, there are several ways to write long strings in Java. The choice depends on the specific requirements and constraints of your program.
Java String format long placeholder
I'd be happy to help with that! In Java, when using the String.format()
method to create a formatted string, you can use placeholders to insert values into the string. The most common placeholder is %s
, which represents a string value.
However, if you want to format a long value, you would typically use %d
as the placeholder. This is because Java uses the %d
placeholder for decimal integer values.
Here's an example of how you might use String.format()
with a long value:
long myLongValue = 123456789L;
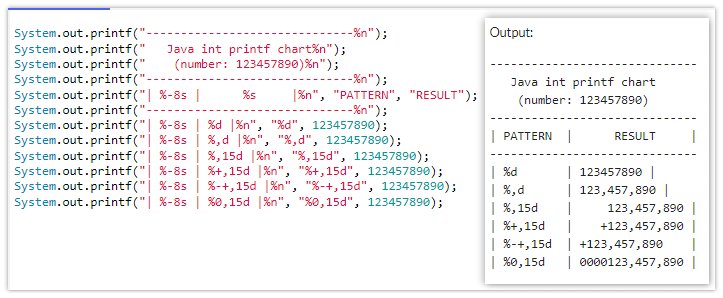
String formattedString = String.format("The value is %d", myLongValue);
System.out.println(formattedString); // prints "The value is 123456789"
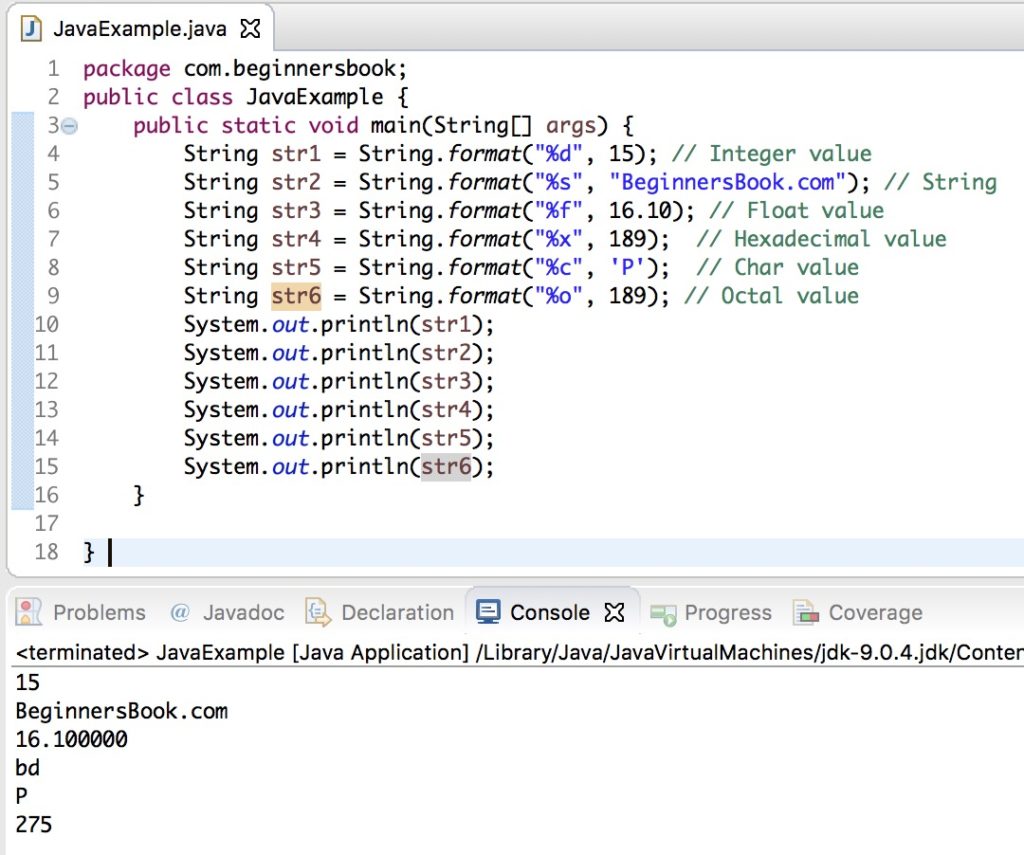
In this example, the %d
placeholder in the format string is replaced with the value of myLongValue
, which is a long integer.
Now, let's talk about some specific rules and guidelines for using placeholders in Java:
%s for strings: When you want to insert a string value into your formatted string, use the%s
placeholder. %d for decimal integers: For values of type int
, long
, or double
, use the %d
placeholder. %f for floating point numbers: If you have a float
or double
value and want to format it with decimal places, use the %f
placeholder. %x or %X for hexadecimal integers: When working with binary data or hexadecimal representations of integer values, use the %x
(lowercase) or %X
(uppercase) placeholder.
Here are some more specific examples:
int myIntValue = 123;
String formattedString1 = String.format("The value is %d", myIntValue);
System.out.println(formattedString1); // prints "The value is 123"
double myDoubleValue = 3.14159;
String formattedString2 = String.format("The value is %f", myDoubleValue);
System.out.println(formattedString2); // prints "The value is 3.141590"
long myLongValue = 123456789L;
String formattedString3 = String.format("The value is %d", myLongValue);
System.out.println(formattedString3); // prints "The value is 123456789"
And, finally, here are some things to keep in mind when using placeholders with String.format()
:
%d
placeholders and then try to fill it with fewer values, Java will throw an InvalidFormatConversionException
. Be mindful of the type of value being inserted: Remember that different placeholders are used for different types of values (e.g., strings, integers, floating-point numbers). Use the correct format specifiers for your needs: Don't be afraid to experiment with different format specifiers and see how they change the appearance of your formatted string.
I hope this helps clarify things! Let me know if you have any further questions.