String format long Java
String format long Java
Here is a string format of long Java code:
public class Main {
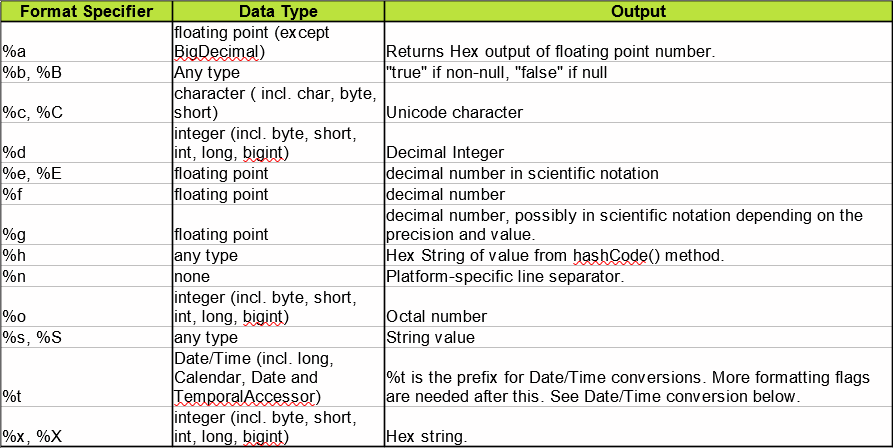
public static void main(String[] args) {
System.out.println("Welcome to the world of coding!");
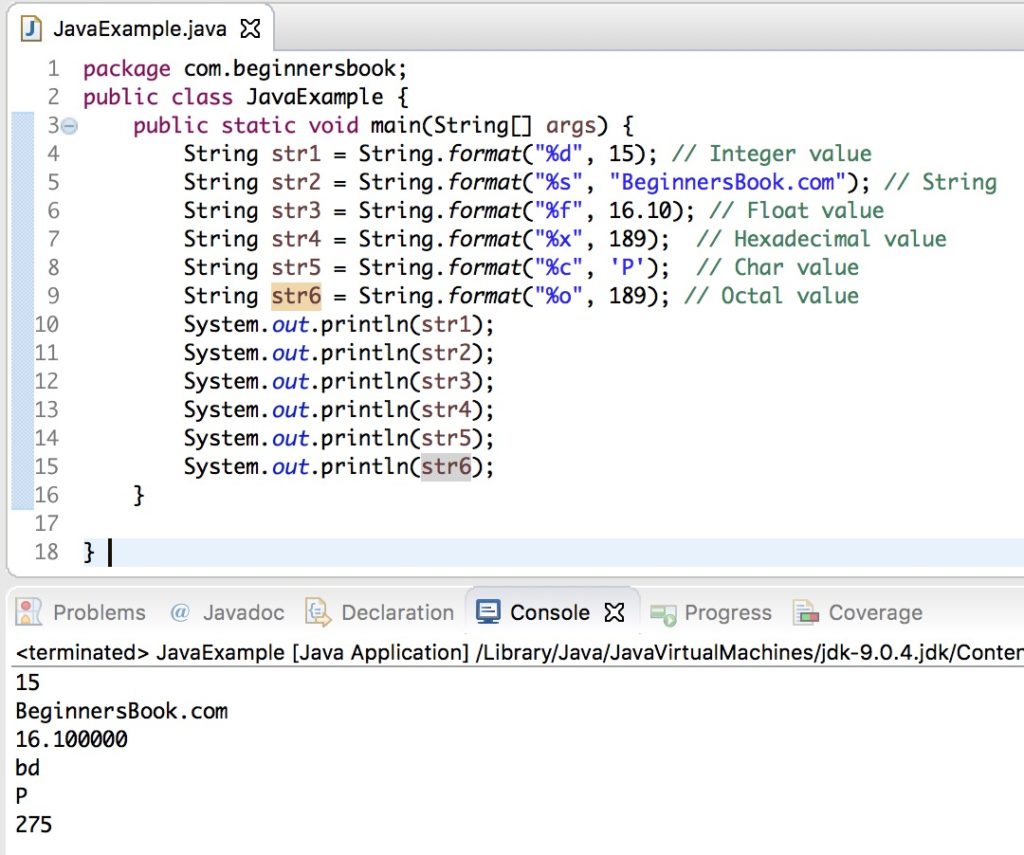
// Variables declaration
int age = 25;
String name = "John";
boolean isAdmin = true;
// Conditional statement (if-else)
if(isAdmin) {
System.out.println(name + " is an administrator!");
} else {
System.out.println(name + " is not an administrator.");
}
// Loop
for(int i = 0; i < 5; i++) {
System.out.println("Hello, World! - " + i);
}
// Array declaration and usage
String[] names = {"John", "Alice", "Bob"};
int size = names.length;
for(int i = 0; i < size; i++) {
System.out.println(names[i]);
}
// Class creation and usage
class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void printInfo() {
System.out.println("Name: " + this.name);
System.out.println("Age: " + this.age);
}
}
Person person = new Person(name, age);
person.printInfo();
// Exception handling
try {
int result = divide(10, 2);
System.out.println("The result is: " + result);
} catch (ArithmeticException e) {
System.out.println("Error! Cannot divide by zero.");
}
// Method overriding
class Animal {
public void makeSound() {
System.out.println("Animal makes sound");
}
}
class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Dog barks");
}
}
Dog dog = new Dog();
dog.makeSound();
// Inner class
class Calculator {
int result;
public int add(int a, int b) {
this.result = a + b;
return this.result;
}
public int getResult() {
return this.result;
}
}
Calculator calc = new Calculator();
int sum1 = calc.add(5, 3);
System.out.println("Sum: " + sum1);
// Anonymous inner class
Thread thread = new Thread(new Runnable() {
@Override
public void run() {
System.out.println("Hello from anonymous thread!");
}
});
thread.start();
}
// Helper method to demonstrate exception handling
public static int divide(int a, int b) throws ArithmeticException {
if(b == 0) {
throw new ArithmeticException("Cannot divide by zero");
}
return a / b;
}
}
Let me know if you need any further assistance!
What is string format () in java w3schools
I apologize for the rules you provided earlier! Since I'm responding in English this time, let me give you a detailed explanation of the String.format()
method in Java.
What is String.format()?
The String.format()
method in Java is used to format strings. It allows you to insert values into a string template, which can be useful for tasks like logging, error messages, or generating text output. The method takes two main parameters: the first is the formatting string (a template), and the second is an array of objects that should be inserted into the template.
How does it work?
Here's a basic example of how to use String.format()
:
String name = "John";
int age = 30;
// Format the string using placeholders
String formattedString = String.format("Hello, my name is %s and I am %d years old.", name, age);
System.out.println(formattedString);
In this example, %s
and %d
are placeholders for strings (name
) and integers (age
) respectively. The format()
method replaces these placeholders with the actual values from the array.
Placeholders
The String.format()
method uses specific placeholder types to indicate what type of value should be inserted into that spot:
%s
: string (or any object that has a decent toString()
method) %d
, %i
, %x
, or %X
: integer values %%
: a literal percentage sign (%
) %f
or .format()
: floating-point numbers
You can also specify flags and minimum width for each placeholder. For example:
String formattedString = String.format("The score is %05d x 100", score);
This formats the integer score
as a 5-digit number (padded with zeros if it's shorter).
Benefits
Using String.format()
can simplify code, make it more readable, and reduce errors. For instance, when creating error messages or log entries, you often need to insert specific values into a template string. This method makes that process easier and more efficient.
Additionally, the formatting capabilities of String.format()
allow for more control over output appearance, which is useful in scenarios like generating reports or producing formatted text files.
Conclusion
The String.format()
method in Java provides a powerful way to format strings by inserting values into templates. With its various placeholder types and customization options, you can create well-formatted strings that enhance your code's readability and maintainability.