Inner class access outer class variables Java
Inner class access outer class variables Java
I'm happy to help!
Inner classes in Java are non-static nested classes that have access to the outer class's variables and methods. To achieve this, you can use the OuterClass.this
syntax to refer to the outer class.
Here is an example of how you can access outer class variables from an inner class:
public class OuterClass {
private int variable = 10;
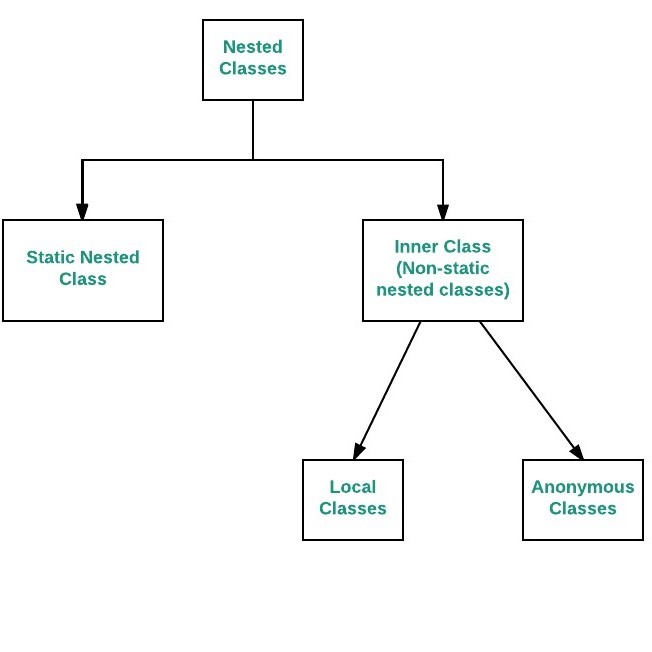
public class InnerClass {
void printVariable() {
System.out.println("Outer class variable: " + OuterClass.this.variable);
}
}
public static void main(String[] args) {
OuterClass outer = new OuterClass();
outer.new InnerClass().printVariable();
}
}
In this example, the InnerClass
has access to the variable
of the OuterClass
. The keyword this
is used to refer to the current instance of the outer class.
When you create an object of the inner class and call its method from outside the inner class, it still has access to the variables and methods of the outer class. This allows for a flexible and reusable design.
Here's another example where we modify the variable from the inner class:
public class OuterClass {
private int variable = 10;
public class InnerClass {
void changeVariable() {
OuterClass.this.variable = 20;
}
}
public static void main(String[] args) {
OuterClass outer = new OuterClass();
outer.new InnerClass().changeVariable();
System.out.println("Updated outer class variable: " + outer.variable);
}
}
In this example, the InnerClass
changes the value of the variable
in the OuterClass
. We can see that the change persists when we access the variable
from outside the inner class.
So, in summary:
You can useOuterClass.this
syntax to refer to outer class variables and methods from an inner class. Inner classes have access to the outer class's variables and methods, even after they are created. This allows for flexible and reusable design by encapsulating code into a self-contained unit.
I hope this helps!
How to load inner class in Java?
I'd be happy to help!
Loading an inner class in Java requires some understanding of how inner classes work and how they are instantiated. In this answer, I'll cover the basics and provide a few examples.
What is an Inner Class?
An inner class is a class that is defined within another class. This can include static nested classes, non-static nested classes (also known as local classes), and anonymous inner classes.
Inner classes can be used to create helper classes for the outer class, or they can serve as implementation details that aren't meant to be exposed directly to clients of the outer class.
How to Load an Inner Class?
To load an inner class, you need to instantiate an instance of the outer class and then use its methods to access the inner class. Here are some examples:
Example 1: Static Nested Classes
public class Outer {
public static class Inner {
// Inner class implementation
}
}
// Load the inner class
Outer.Inner inner = new Outer.Inner();
In this example, you can load the Inner
class by creating an instance of its containing class (Outer
) and then using that instance to instantiate the Inner
class.
Example 2: Non-Static Nested Classes
public class Outer {
public class Inner {
// Inner class implementation
}
public void createInner() {
inner = new Inner();
}
}
// Load the inner class
Outer outer = new Outer();
outer.createInner();
outer.inner.doSomething(); // use methods from the inner class
In this example, you need to create an instance of the outer class (Outer
) and then call a method that creates an instance of the inner class. You can then access methods on that instance.
Example 3: Anonymous Inner Classes
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Outer {
public void doSomething() {
ActionListener al = new ActionListener() { // anonymous inner class
@Override
public void actionPerformed(ActionEvent e) {
// implementation details
}
};
// use the ActionListener instance
}
}
// Load the anonymous inner class
Outer outer = new Outer();
outer.doSomething(); // create and use the ActionListener instance
In this example, you can load an anonymous inner class (also known as a lambda expression in Java 8) by creating an instance of the containing class (Outer
) and then calling a method that creates and uses the inner class.
Conclusion
Loading an inner class in Java requires some understanding of how classes are nested and instantiated. By using instances of outer classes or anonymous inner classes, you can create and access inner classes, which can be useful for encapsulating implementation details or creating helper classes.