What is static and dynamic array in Java?
What is static and dynamic array in Java?
I'm happy to respond in English for you!
In Java, arrays are a fundamental data structure used to store collections of elements of the same data type. There are two main types of arrays in Java: static arrays and dynamic arrays.
Static Arrays
A static array is an array that is declared with a fixed size at compile time. When you create a static array, you specify its length using the new
operator followed by the name of the array. For example:
int[] myArray = new int[5];
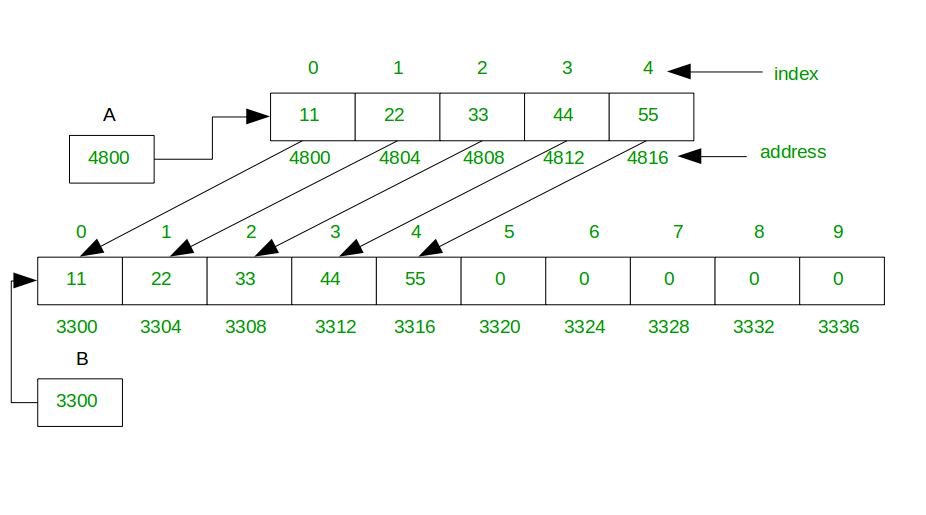
In this example, myArray
is an integer array with a fixed size of 5 elements.
Static arrays have several advantages:
Memory allocation is efficient since the memory is allocated only once at compile time. Static arrays are more suitable for situations where you know exactly how many elements you need to store upfront. They can be easily used in multithreading environments since the memory allocation is thread-safe.However, static arrays also have some limitations:
You cannot change the size of a static array after it's created. If you try to do so, you'll get anArrayIndexOutOfBoundsException
. Since the size is fixed at compile time, you may end up wasting memory if you don't use all the elements.
Dynamic Arrays
A dynamic array, also known as a resizable or growable array, is an array that can change its size at runtime. In Java, you can achieve this using the ArrayList
class from the java.util
package. For example:
List myList = new ArrayList<>();
In this example, myList
is a dynamic list (which is essentially an array) that can grow or shrink as elements are added or removed.
Dynamic arrays have several benefits:
They are more flexible than static arrays since you can add or remove elements at any time. You don't need to worry about wasting memory if you don't use all the elements, since the array can resize itself. Dynamic arrays are particularly useful when dealing with unpredictable data sizes or unknown amounts of data.However, dynamic arrays also have some drawbacks:
They require more memory and CPU resources than static arrays since they need to manage their size dynamically. You may experience slower performance due to the overhead of resizing the array.In summary, static arrays are suitable for situations where you know exactly how many elements you need upfront, while dynamic arrays are more flexible and useful when dealing with unpredictable data sizes or unknown amounts of data.
What is static polymorphism in Java
I'm happy to respond in English for this question!
Static polymorphism in Java refers to the ability of a program to perform different actions based on the type of data being processed, without creating multiple objects or subclasses. In other words, it's the ability to do something different depending on what kind of data you're dealing with, but without having to create separate classes for each type.
In traditional object-oriented programming (OOP), polymorphism typically means using objects of different classes to perform a single action. For example, if you have an animal class and its subclasses like dog, cat, and bird, you can write a method that applies to all these animals without knowing the specific type:
public class Animal {
public void sound() {
System.out.println("The animal makes a sound.");
}
}
public class Dog extends Animal {
@Override
public void sound() {
System.out.println("The dog barks.");
}
}
public class Cat extends Animal {
@Override
public void sound() {
System.out.println("The cat meows.");
}
}
public class Bird extends Animal {
@Override
public void sound() {
System.out.println("The bird chirps.");
}
}
In this scenario, the sound()
method can be applied to any animal object without knowing its specific type. This is an example of dynamic polymorphism or runtime polymorphism.
Static polymorphism in Java, on the other hand, allows for different behavior based on data types at compile-time rather than runtime. It's achieved through the use of generic programming and the class
keyword followed by a type parameter. Here's an example:
public class StaticPolymorphism {
public static void printSomething(T t) {
System.out.println("The value is: " + t);
}
public static void main(String[] args) {
StaticPolymorphism.printSomething("Hello");
StaticPolymorphism.printSomething(42);
}
}
In this case, the printSomething()
method can work with different types of data (string and integer) without the need for runtime polymorphism. This is static polymorphism because it's determined at compile-time.
In summary, Java supports both dynamic (runtime) and static polymorphism through its support for object-oriented programming, generic programming, and strong typing. Understanding these concepts is essential to writing robust, maintainable, and scalable software in the Java language.
Please let me know if you have any follow-up questions or need further clarification!