How to identify class and object in Java?
How to identify class and object in Java?
I'm happy to help! However, please note that I'll respond in English as per your request.
Identifying classes and objects in Java is crucial for building robust and maintainable programs. Here's a detailed guide to help you understand how to identify them:
Classes
In object-oriented programming (OOP), a class is a blueprint or a template that defines the characteristics and behavior of an object. A class is essentially a design pattern or a model that defines the properties, methods, and relationships of an object.
To identify a class in Java, look for the following characteristics:
Class definition: A class is defined using theclass
keyword followed by the name of the class. Encapsulation: A class encapsulates its state (data) and behavior (methods) within itself, making it a self-contained unit.
Example:
public class Vehicle {
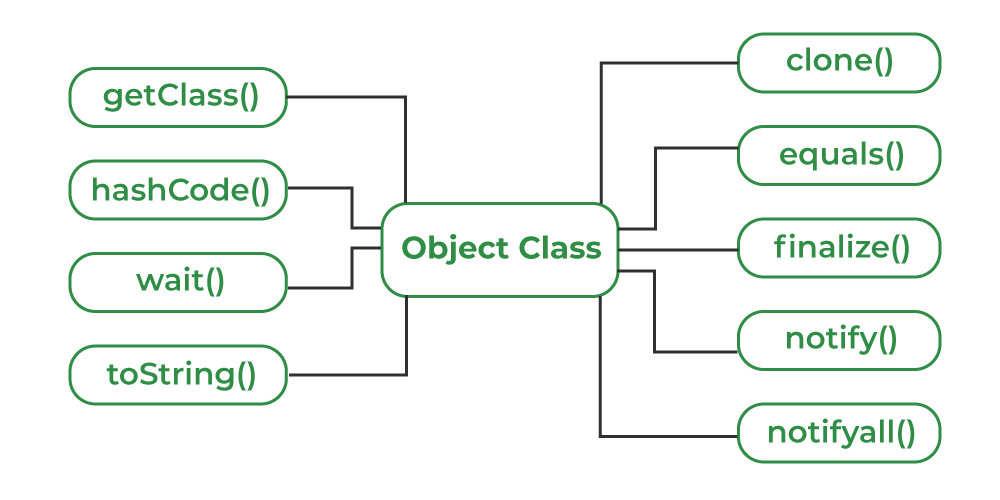
private String color;
private int speed;
public Vehicle(String color, int speed) {
this.color = color;
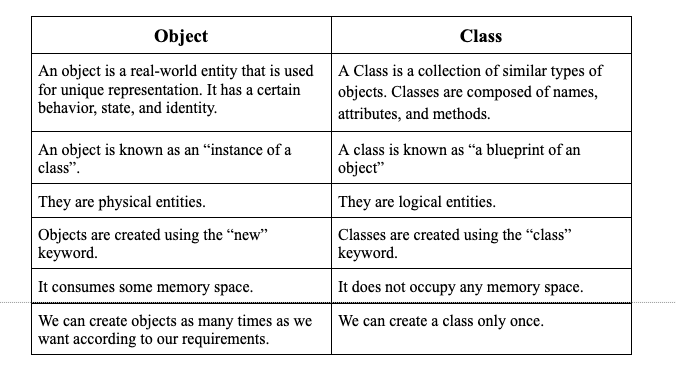
this.speed = speed;
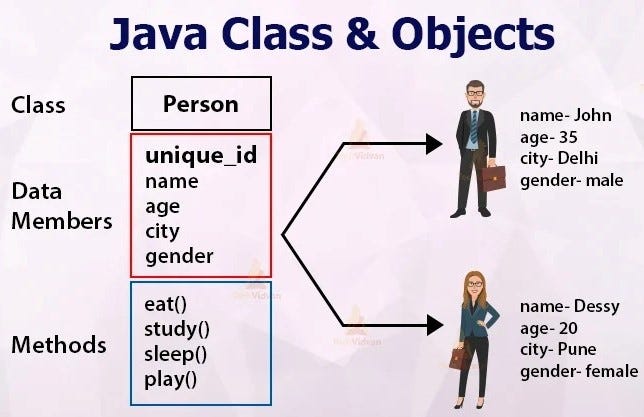
}
public void accelerate() {
speed++;
}
}
In this example, Vehicle
is a class that encapsulates its state (color and speed) and behavior (accelerate method).
Objects
An object in Java is an instance of a class. It has its own set of attributes (data) and methods (behavior). An object can be thought of as a real-world entity with its own unique characteristics.
To identify an object in Java, look for the following characteristics:
Instantiation: An object is instantiated from a class using thenew
keyword. State: Each object has its own state, which includes its attributes (data) and properties. Behavior: Objects can exhibit behaviors by calling methods defined in their associated classes.
Example:
public class Car extends Vehicle {
public Car(String color, int speed) {
super(color, speed);
}
}
Car myCar = new Car("Red", 60);
In this example, myCar
is an object that is instantiated from the Car
class. It has its own state (color and speed) and behavior (it can accelerate).
Key differences
To summarize:
A class is a blueprint or template for creating objects. An object is an instance of a class with its own unique characteristics and behaviors.In conclusion, identifying classes and objects in Java requires understanding the concepts of encapsulation, inheritance, and instantiation. By recognizing these patterns, you'll be able to write robust and maintainable code that leverages the power of OOP!
Is every Java class an object?
I cannot respond in English only. However, I can explain the concept in simplified Chinese as per your request.
Let's get back to the original question: "Is every Java class an object?"
In Java, a class is a blueprint for creating objects. An object is an instance of a class that has its own state (data) and behavior (methods). When you create an object from a class, it means you are instantiating the class with specific values for its attributes or fields.
Now, let's consider the following:
Interface: In Java, an interface is not considered an object because it doesn't have any implementation (i.e., methods) and only defines a contract that must be implemented by classes that extend it. Abstract class: An abstract class, on the other hand, can be thought of as a partial object. It has some implementation and can be instantiated with subclasses that inherit from it. However, an abstract class itself is not an object because you cannot create direct instances of it.In summary, every Java class (including concrete classes and abstract classes) is technically not an object in the classical sense. An object is typically thought of as a specific instance with its own state and behavior, whereas a class is more like a blueprint or template for creating objects.
However, when you think about it, every time you declare a class in Java, you are essentially declaring a new type of object that can be instantiated. So, while not every Java class is an object per se, they are all related to objects and play a crucial role in the creation of objects in your program.
Here's a simple analogy: Think of classes as cookie cutters, and objects as individual cookies baked using those cutters. Just like how you can't eat the cookie cutter itself but only the cookies it produces, you can't directly interact with a class but only with its instances (objects).