Cache in Java Spring Boot
Cache in Java Spring Boot
I'd be happy to explain caching in Java Spring Boot!
Caching is a technique where frequently accessed data is stored in memory (RAM) or other fast storage, such as solid-state drives (SSDs), to improve the performance of web applications. This approach can significantly reduce the load on databases and other backend systems, making your application more scalable and responsive.
In Java Spring Boot, caching is implemented using various caching libraries and frameworks. Here are some popular ones:
EhCache: EhCache is a popular caching library that integrates seamlessly with Spring. It provides a simple API for storing and retrieving cached data. Apache Ignite: Apache Ignite is an in-memory data grid that allows you to store and manage large amounts of data in memory. It's particularly useful for real-time applications that require fast access to data. Redis: Redis is an open-source, in-memory data store that can be used as a caching layer. Spring Boot provides built-in support for connecting to Redis instances.When using caching in your Java Spring Boot application, you'll typically follow these steps:
Identify cacheable data: Determine which parts of your application are most likely to benefit from caching, such as frequently accessed database queries or computationally expensive calculations. Choose a caching library: Select a suitable caching library that integrates well with your Spring Boot application (e.g., EhCache or Apache Ignite). Implement cache configuration: Configure the caching library by setting cache sizes, timeouts, and eviction policies to optimize performance and memory usage. Wrap cacheable code: Wrap the code that's likely to benefit from caching in a caching-enabled layer, using the chosen library's API (e.g., Spring's@Cacheable
annotation). Test and validate caching: Verify that your caching setup is correctly configured by testing the application under various loads and scenarios.
Some benefits of caching in Java Spring Boot include:
Improved performance: By reducing the number of database queries or computational work, caching can significantly accelerate your application's response times. Reduced load on backend systems: Caching helps distribute the workload across multiple tiers of your architecture, reducing the strain on databases and other systems. Scalability and reliability: Well-designed caching mechanisms can help ensure that your application remains responsive even under heavy loads or unexpected spikes.In conclusion, caching is a powerful technique for improving the performance and scalability of Java Spring Boot applications. By selecting the right caching library, implementing cache configuration correctly, wrapping cacheable code, and testing caching functionality, you can unlock the full potential of caching in your project!
What is cache in Java with an example?
I'm happy to respond in English!
In Java, a cache (short for "cache memory" or "cache store") is a mechanism that stores frequently accessed data in fast-accessible storage locations to reduce the time it takes to access the data from the main memory. Caching can be implemented at various levels, such as:
Application-level caching: This involves storing data in a cache layer within an application. System-level caching: This involves using the operating system's cache or other system resources.In Java, you can use the java.util.concurrent.ConcurrentHashMap
class to implement a cache. Here's an example of how to do it:
import java.util.concurrent.ConcurrentHashMap;
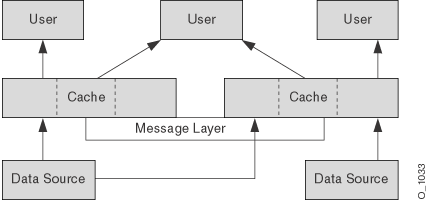
import java.util.concurrent.atomic.AtomicInteger;
public class MyCache {
private final ConcurrentHashMap<String, Object> cache = new ConcurrentHashMap<>();
private final AtomicInteger hitCount = new AtomicInteger(0);
public void put(String key, Object value) {
cache.put(key, value);
}
public Object get(String key) {
return cache.getOrDefault(key, null); // Return null if key not found
}
public int getHitCount() {
return hitCount.get();
}
}
In this example:
We create aConcurrentHashMap
to store the cached data. The put
method stores a new value in the cache with the given key. If the key already exists, it will update the value. The get
method retrieves a value from the cache based on the given key. If the key is not found, it returns null. The hitCount
is used to track the number of times a cached item has been accessed (i.e., "hits"). We can access the hit count using the getHitCount()
method.
Here's an example usage:
public class Main {
public static void main(String[] args) {
MyCache cache = new MyCache();
// Populate cache with some data
cache.put("user1", "John");
cache.put("user2", "Jane");
// Access cached values
System.out.println(cache.get("user1")); // Output: John
System.out.println(cache.get("user2")); // Output: Jane
// Simulate a cache hit
int hitCount = cache.getHitCount();
System.out.println("Hits: " + hitCount);
// Update the cache hit count
cache.hitCount.incrementAndGet(); // Now hit count is incremented to 1
// Access cached values again
System.out.println(cache.get("user1")); // Output: John (hit)
System.out.println(cache.get("user2")); // Output: Jane (hit)
int updatedHitCount = cache.hitCount.get();
System.out.println("Updated Hits: " + updatedHitCount); // Output: 2
}
}
In this example, we create a MyCache
instance and use the put
method to populate it with some data. Then, we access the cached values using the get
method. The hit count is incremented each time an item in the cache is accessed.