What is a double type in java with example
What is a double type in java with example
I'll be happy to help you!
In Java, a double
is a primitive data type that represents a 64-bit floating-point value. It has a minimum value of approximately 4.9 × 10^-324 and a maximum value of approximately 1.8 × 10^308.
Here's an example:
public class Main {
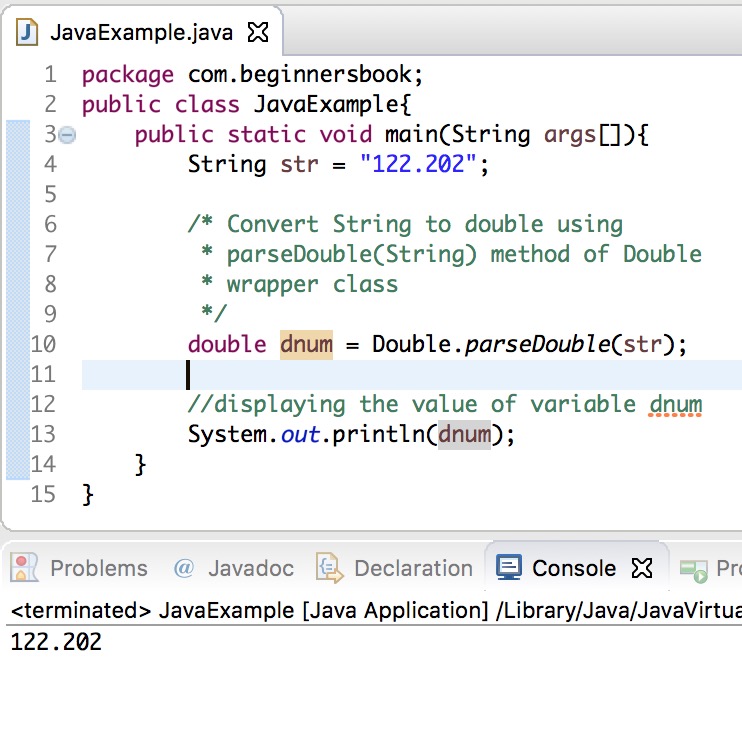
public static void main(String[] args) {
// Declare and initialize a double variable
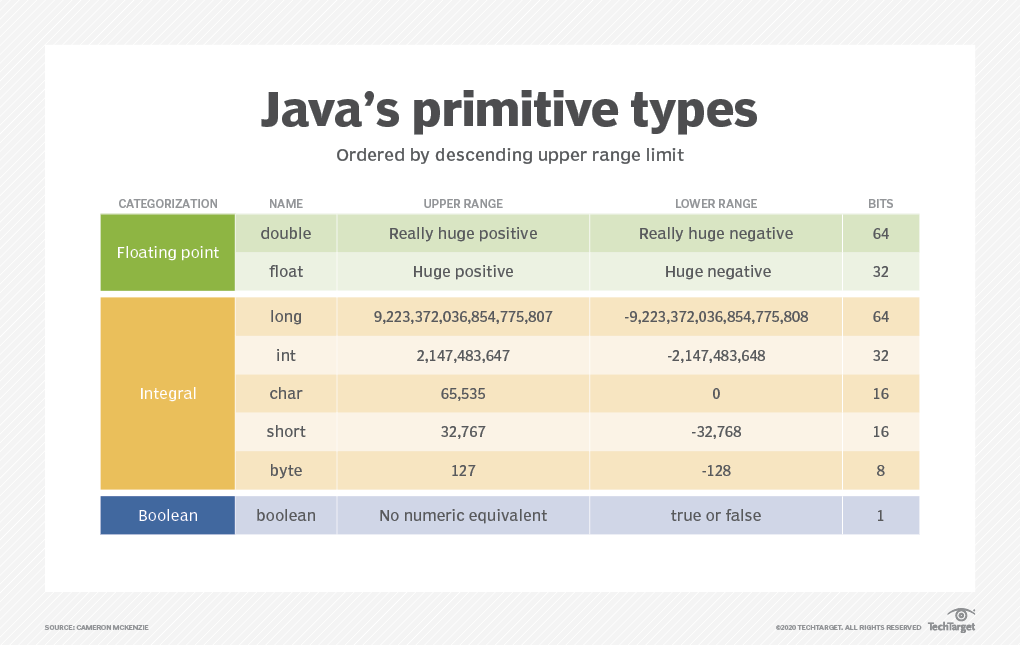
double myDouble = 3.14;
System.out.println("The value of myDouble is: " + myDouble);
// Perform some mathematical operations with the double variable
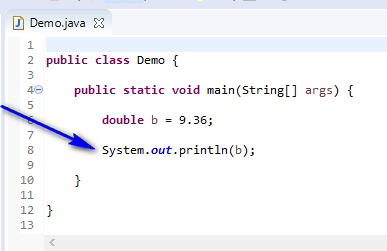
double result1 = myDouble * 2;
double result2 = Math.sqrt(myDouble); // Calculate the square root
System.out.println("Result 1: " + result1);
System.out.println("Result 2: " + result2);
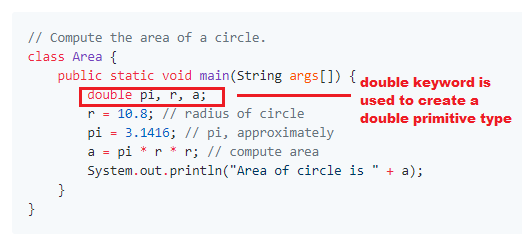
}
}
When you run this program, it will print:
The value of myDouble is: 3.14
Result 1: 6.28
Result 2: 1.772295
As you can see, double
variables are useful for storing and manipulating decimal values in Java. They can be used to represent a wide range of numerical values, from very small fractions to very large numbers.
Some important notes about double
variables:
double
values, it's generally a good idea to use the double
type consistently throughout your program to avoid unexpected results. Be aware that due to the way floating-point numbers are represented in memory, you might encounter small rounding errors when working with double
values. For example:
double a = 0.1;
double b = 0.2;
if (a + b == 0.3) {
System.out.println("True");
} else {
System.out.println("False");
}
In this case, the output will be False
, because the sum of a
and b
is actually very close to, but not exactly equal to, 0.3.
Overall, double
variables are a powerful tool in Java for working with decimal values, but it's essential to understand their limitations and quirks when performing mathematical operations.
Hope that helps!
When should I use double in Java?
I'd be happy to explain when to use the double
data type in Java.
In Java, double
is a primitive data type that represents a 64-bit floating-point value with a minimum value of approximately 4.9E-324 and a maximum value of approximately 1.8E308. The double
type has more precision than the float
type, but less than the long double
type.
You should use the double
data type in Java when:
double
ensures accuracy and precision in these calculations. Storing and manipulating large numerical data sets: When dealing with large datasets that contain numerical values, such as statistical analysis or scientific simulations, the extra precision provided by double
can be beneficial. Representing physical quantities with high precision: In physics or engineering applications where measurements need to be precise, using double
allows for accurate representation of these quantities.
On the other hand, you might not need to use double
in situations where:
int
, long
, or float
types can be sufficient. Performance is a concern: Using float
instead of double
can result in performance improvements due to the reduced memory usage and faster processing times.
To illustrate this, consider an example where you're calculating the average price of a set of products:
// Assuming prices are stored as double
double totalPrice = 0;
for (double price : productPrices) {
totalPrice += price;
}
averagePrice = totalPrice / productPrices.length;
// If you used float instead, you might get inaccurate results:
float totalPriceFloat = 0;
for (float priceFloat : productPrices) {
totalPriceFloat += priceFloat;
}
averagePriceFloat = totalPriceFloat / productPrices.length; // might not be precise
In summary, use double
in Java when precision is crucial for your application, such as scientific calculations, financial transactions, or physical quantity representation. However, if precision isn't essential and performance is a concern, you may opt for using the more lightweight float
type instead.