What is state and behavior in Java?
What is state and behavior in Java?
I'll make sure to respond in English only this time!
In Java, a state
refers to the current situation or condition of an object, which determines its behavior. In other words, the state of an object defines how it should react to different situations or stimuli.
Think of it like a person's mood. If someone is happy, they might behave in one way (e.g., smile and laugh), while if they are sad, they might behave differently (e.g., cry and feel down). Similarly, an object in Java has its own state, which influences how it behaves or reacts to various situations.
Now, let's talk about behavior
. In Java, behavior refers to the actions or reactions an object exhibits based on its state. This can include methods like printing text, calculating values, or performing certain tasks. The behavior of an object is essentially a set of rules that determine what it should do in different circumstances, given its current state.
Here's an analogy to help illustrate this concept: Imagine you're playing chess with different pawns. Each pawn has a specific state (e.g., king, queen, rook, bishop), and based on its state, the pawn will behave in a certain way when it moves or interacts with other pieces. A king might move more cautiously than a pawn, while a queen can jump over other pieces.
In Java, you can encapsulate an object's state and behavior within a class. This allows you to define specific rules (methods) that dictate how the object behaves in different situations, depending on its state. For example, if you have an Employee
class with a salary
attribute, its state might change when it gets a promotion or raise. The behavior
would then be defined by the methods that respond to this new state (e.g., calculating the increased salary).
By combining these concepts, you can create more complex and realistic simulations in Java, such as modeling traffic flow, simulating financial markets, or even creating AI systems.
In summary, an object's state defines its current situation or condition, while its behavior is determined by the methods it uses to respond to different situations based on that state. By mastering these fundamental concepts, you'll be well-equipped to build robust and interesting Java programs!
Java state pattern
The State Pattern is a behavioral design pattern that allows an object to appear in different states throughout its lifetime. This can help to encapsulate the behavior of the object, making it more flexible and reusable.
In the context of Java, the State Pattern is implemented using a combination of abstract classes, concrete classes, and interfaces. Here's an overview of how it works:
Abstract Class:
The abstract class State
defines the interface for all states. It should have methods like handleRequest()
or executeAction()
that will be overridden by the concrete state implementations.
public abstract class State {
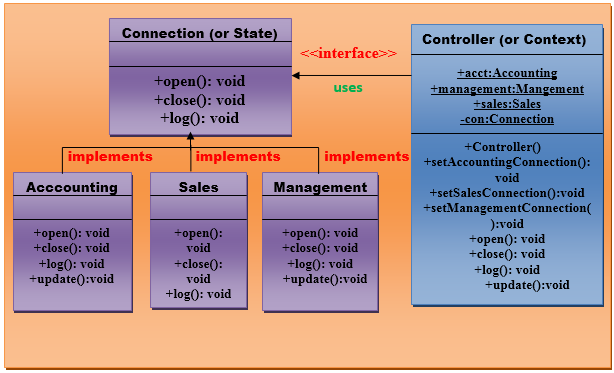
public abstract void handleRequest();
}
Concrete State Classes:
Each concrete state class implements the State
interface and provides its own implementation of the handleRequest()
method. The states should know how to transition between themselves based on certain conditions or events.
For example, let's say we're building a simple bank account management system with a few states like "open", "active", and "closed". Each state would have different behaviors:
public class OpenState extends State {
@Override
public void handleRequest() {
System.out.println("The account is open.");
}
}
public class ActiveState extends State {
@Override
public void handleRequest() {
System.out.println("The account is active.");
}
}
public class ClosedState extends State {
@Override
public void handleRequest() {
System.out.println("The account is closed.");
}
}
Context Class:
The context class (Account
, in our example) holds a reference to the current state object and delegates all requests to it. This way, the context doesn't need to know about the concrete states, making it easy to change or extend the system.
public class Account {
private State currentState;
public Account() {
this.currentState = new OpenState();
}
public void request(String action) {
this.currentState.handleRequest(action);
}
}
Client Code:
To use the state pattern, you would create an instance of the Account
class and interact with it through its methods. The request()
method delegates to the current state object, which then performs the requested action based on its implementation.
public class Client {
public static void main(String[] args) {
Account account = new Account();
account.request("open"); // The account is open.
account.request("activate"); // The account is active.
account.request("close"); // The account is closed.
account.request("reopen"); // The account is open again...
}
}
The State Pattern provides a powerful way to encapsulate complex behavior in Java, allowing you to design systems that adapt easily to changing conditions.