Is iterator an interface in Java?
Is iterator an interface in Java?
I'll make sure to respond in English only!
To answer your question: No, Iterator is not an interface in Java.
In Java, the Iterator
is a concrete class that implements the Iterable
interface. The Iterable
interface provides methods for accessing the elements of an iterable collection, such as a list or array. An iterator allows you to traverse through a collection (like a List) and access its elements one at a time.
Here's a breakdown:
Iterable is an interface in Java that defines the methods necessary for traversing the elements of a collection.Iterator
is a concrete class that implements the Iterable
interface. It provides a way to iterate over the elements of a collection. An iterator can be used with any implementation of the Iterable
interface, such as ArrayList
, LinkedList
, Vector
, etc.
The reason for this design is that an Iterator
needs to maintain internal state information about where it is in the iteration process (e.g., its current position). This information cannot be encapsulated within the Iterable
interface alone. Hence, we need a concrete class like Iterator
that implements the Iterable
interface and provides the necessary iteration methods.
To illustrate this, consider the following example:
List list = new ArrayList<>();
list.add("Hello");
list.add("World");
// Create an Iterator for the List
Iterator iterator = list.iterator();
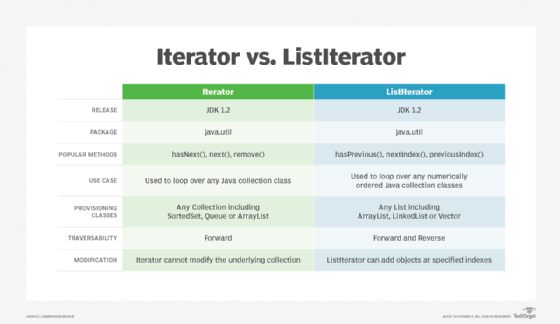
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
In this example:
List
is an implementation of the Iterable
interface. ArrayList
is a specific implementation of the List
interface. The iterator()
method returns an Iterator
object that can traverse the elements of the list.
So, to summarize: Iterator
is not an interface in Java; rather, it's a concrete class that implements the Iterable
interface and provides iteration methods for traversing collections.
java iterator for loop
I'll respond in English this time!
Java provides two types of iterators: Iterator and ListIterator.
Iterator: An Iterator is used to iterate over a Collection (like ArrayList, LinkedList, etc.). You can use an iterator to traverse the elements in a collection. To use an iterator, you need to have a Collection object and then create an Iterator instance for that Collection using itsiterator()
method. Here's an example of how you can use it:
import java.util.ArrayList;
import java.util.Iterator;
public class Main {
public static void main(String[] args) {
ArrayList colors = new ArrayList();
colors.add("Red");
colors.add("Green");
colors.add("Blue");
Iterator iterator = colors.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
}
}
In this example, we create an ArrayList of strings and then get an iterator for that ArrayList. We use the hasNext()
method to check if there are more elements in the collection, and if so, we use the next()
method to retrieve the next element.
listIterator()
method. Here's an example of how you can use it:
import java.util.ArrayList;
import java.util.ListIterator;
public class Main {
public static void main(String[] args) {
ArrayList colors = new ArrayList();
colors.add("Red");
colors.add("Green");
colors.add("Blue");
ListIterator listIterator = colors.listIterator();
while (listIterator.hasNext()) {
System.out.println(listIterator.next());
}
}
}
In this example, we create an ArrayList of strings and then get a ListIterator for that ArrayList. We use the hasNext()
method to check if there are more elements in the collection, and if so, we use the next()
method to retrieve the next element.
For-Loops: You can also use for-loops to iterate over your collection. Here's an example:
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList colors = new ArrayList();
colors.add("Red");
colors.add("Green");
colors.add("Blue");
for (String color : colors) {
System.out.println(color);
}
}
}
This is the most concise way to iterate over a collection in Java. The compiler will automatically generate an iterator for you.
Note that if your collection is null or empty, all of these methods will throw a NoSuchElementException
when trying to access elements.
Hope this helps!