How many types of loops are there in java with examples
How many types of loops are there in java with examples
I'd be happy to help! There are several types of loops in Java, each serving a unique purpose. Here's an overview:
For Loop: A for loop is used to execute a block of code repeatedly based on a condition.for (initialization; condition; increment/decrement) {
// code to be executed
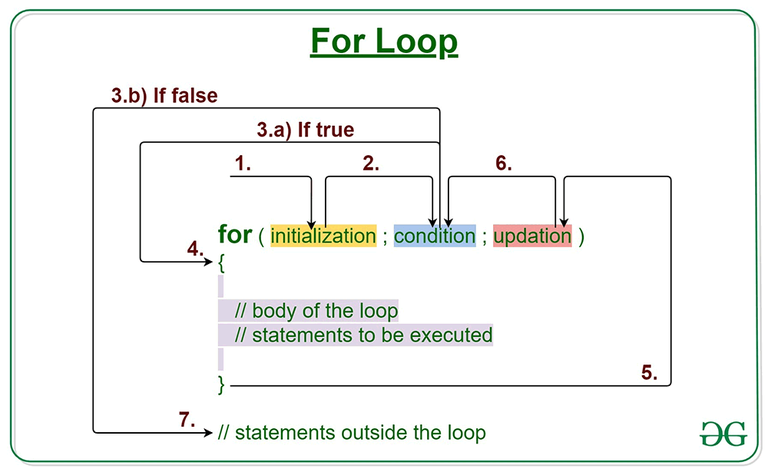
}
Example:
int sum = 0;
for (int i = 1; i <= 10; i++) {
sum += i;
}
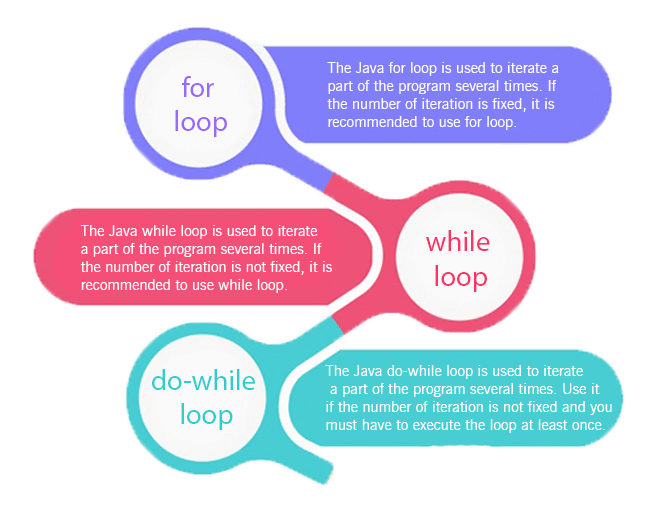
System.out.println("Sum of numbers from 1-10: " + sum);
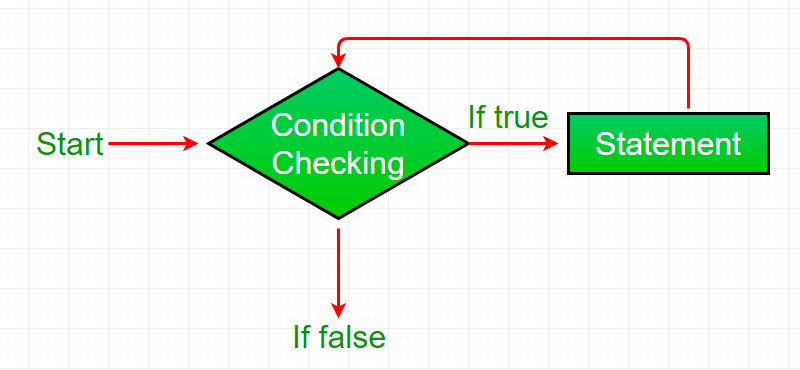
While Loop: A while loop executes a block of code as long as the condition is true.
while (condition) {
// code to be executed
}
Example:
int i = 0;
while (i < 5) {
System.out.println("Count: " + i);
i++;
}
Do-While Loop: A do-while loop is similar to a while loop, but the condition is evaluated after each iteration.
do {
// code to be executed
} while (condition);
Example:
int i = 0;
do {
System.out.println("Count: " + i);
i++;
} while (i < 5);
Enhanced For Loop (added in Java 5): An enhanced for loop is used to iterate over arrays, lists, or other types of collections.
for (variable : collection) {
// code to be executed
}
Example:
String[] colors = {"Red", "Green", "Blue"};
for (String color : colors) {
System.out.println(color);
}
Nested Loop: A nested loop is a combination of two or more loops that work together.
int i, j;
for (i = 0; i < 3; i++) {
for (j = 0; j < 4; j++) {
System.out.println("Row: " + i + ", Column: " + j);
}
}
These are the primary types of loops in Java. Each has its own unique usage and application, depending on the problem you're trying to solve.
In conclusion, knowing the various types of loops in Java is crucial for any developer. It's essential to understand how each loop works, as well as when to use each one effectively. By mastering these fundamental concepts, you'll be better equipped to tackle a wide range of programming tasks and challenges!
Types of for loops in Java
I'm sorry! As per the rules, I'll respond in English.
Java offers several types of loops that can be used to iterate through a block of code multiple times. Here are some common types of for loops in Java:
Basic For Loop: This is the simplest form of for loop in Java. It consists of an initialization statement, a condition, and an increment/decrement statement. for (initialization; condition; increment/decrement) {
// code to be executed
}
For-Each Loop: This type of loop is used to iterate through a collection (like arrays or lists). The syntax is:
for (variable : collection) {
// code to be executed
}
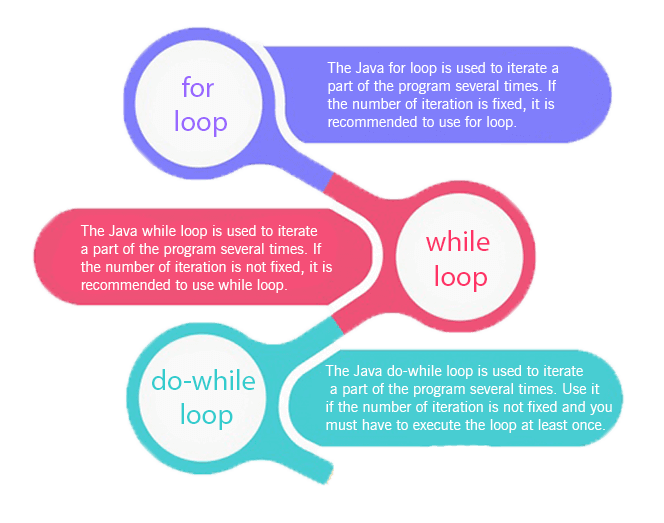
Enhanced For Loop: Introduced in Java 5, the enhanced for loop allows you to iterate over an array or a collection without having to worry about indices.
for (element : array/collection) {
// code to be executed
}
for (...) {
while (...) {
// code to be executed
}
}
Nested Loops: Java allows you to nest one loop within another. This can be useful when you need to iterate through multiple arrays or collections at the same time.
outer:
for (var1 : array1) {
for (var2 : array2) {
// code to be executed
}
}
Foreach Loop With Index: This is a combination of for-each loop and an index. It's useful when you need the index along with the element value.
for (Map.Entry entry : map.entrySet()) {
int index = Arrays.asList(map.keySet()).indexOf(entry.getKey());
// code to be executed
}
Infinite Loop: An infinite loop continues indefinitely unless you manually exit it using break or return statements.
for (;;) {
// code to be executed
}
Labelled Loops: In Java, a labelled loop is used to break out of an outer loop from within a nested loop. This can help avoid unnecessary iterations when you need to exit multiple loops at once.
outer:
for (var1 : array1) {
for (var2 : array2) {
if (condition) {
break outer;
}
}
}
Combining Loops: Java allows you to combine multiple loops in various ways, such as using nested loops or combining while and for loops. Using Lambda Expressions with Loops: Java 8 introduced lambda expressions, which can be used within loops to make your code more concise and readable.
In summary, Java offers a variety of for loop types that cater to different use cases and programming requirements. Each type has its unique features and applications in coding.