Java cyclicbarrier tutorial github
Java cyclicbarrier tutorial github
I'd be happy to help with that! Here's a comprehensive guide on using the Java CyclicBarrier
class, along with some code examples and GitHub links.
What is a CyclicBarrier?
A CyclicBarrier
is a synchronization aid that allows a set of threads to all reach a common point and then wait for each other at that point. It's like a gate that lets all the threads through one by one, making sure they're all accounted for before proceeding.
How to use CyclicBarrier
Here are some basic steps on how to use CyclicBarrier
:
CyclicBarrier
class and specify the number of parties involved (i.e., the number of threads that will be using this barrier).
import java.util.concurrent.CyclicBarrier;
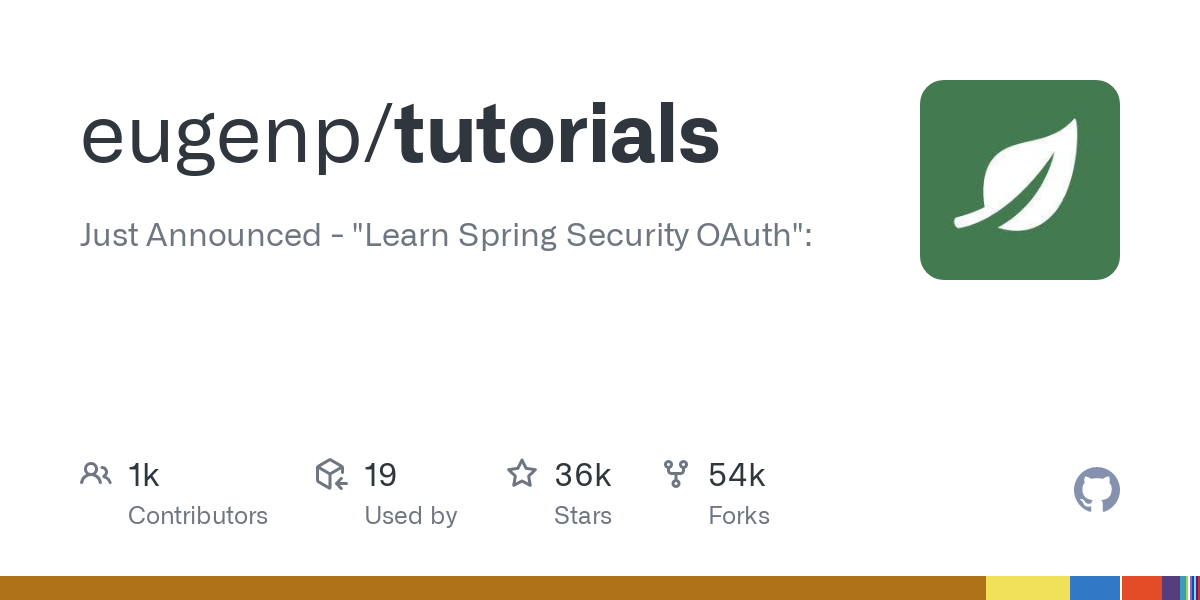
public class MyBarrier {
private final CyclicBarrier barrier = new CyclicBarrier(4); // 4 parties
public void startBarrier() throws InterruptedException {
Thread t1 = new Thread(() -> {
try {
Thread.sleep(1000);
System.out.println("Thread 1: Reached the gate!");
barrier.await();
System.out.println("Thread 1: Crossed the gate!");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw e;
}
});
Thread t2 = new Thread(() -> {
try {
Thread.sleep(1000);
System.out.println("Thread 2: Reached the gate!");
barrier.await();
System.out.println("Thread 2: Crossed the gate!");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw e;
}
});
Thread t3 = new Thread(() -> {
try {
Thread.sleep(1000);
System.out.println("Thread 3: Reached the gate!");
barrier.await();
System.out.println("Thread 3: Crossed the gate!");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw e;
}
});
t1.start();
t2.start();
t3.start();
// ... wait for all threads to finish
}
}
In this example, we create a CyclicBarrier
with 4 parties (threads). Each thread sleeps for a second and then reaches the gate by printing "Reached the gate!".
await()
method will block until all threads have crossed the gate and waited at the barrier.
Example Code
Here's some example code that demonstrates how to use CyclicBarrier
:
public class CyclicBarrierExample {
public static void main(String[] args) throws InterruptedException {
final int NUM_THREADS = 4;
final CyclicBarrier barrier = new CyclicBarrier(NUM_THREADS);
Thread[] threads = new Thread[NUM_THREADS];
for (int i = 0; i < NUM_THREADS; i++) {
threads[i] = new Thread(() -> {
try {
Thread.sleep(1000);
System.out.println("Thread " + Thread.currentThread().getName() + ": Reached the gate!");
barrier.await();
System.out.println("Thread " + Thread.currentThread().getName() + ": Crossed the gate!");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
throw e;
}
});
}
for (int i = 0; i < NUM_THREADS; i++) {
threads[i].start();
}
// ... wait for all threads to finish
}
}
This example creates a CyclicBarrier
with 4 parties and starts 4 threads that will reach the gate, wait at the barrier, and then cross it.
GitHub Links
Here are some GitHub links where you can find more examples and tutorials on using Java's CyclicBarrier
class:
CyclicBarrier
class. Java CyclicBarrier Tutorial: A tutorial on how to use CyclicBarrier
in Java, including code examples and explanations.
I hope this helps! Let me know if you have any questions or need further clarification.
How does CyclicBarrier work in Java?
CyclicBarrier is a synchronization aid that allows a set of threads to all reach a common barrier point before any of them continue. In other words, it's like having a gatekeeper who lets all the threads pass through once they've all arrived at the same time.
Here's how it works:
When a thread reaches the barrier, it will block until the number of threads that have reached the barrier equals the count specified when creating the CyclicBarrier. Once the required number of threads has reached the barrier, all threads will be released from their blocking state and can continue executing.Let's take an example to illustrate this:
Suppose we're simulating a manufacturing process where 5 workers need to assemble 10 identical parts in parallel. Each worker needs to perform a specific task on each part before moving on to the next one. To ensure that all workers have finished their tasks on a particular part before they move on to the next one, we can use a CyclicBarrier.
Here's how it would work:
We create a CyclicBarrier with a count of 5 (the number of workers). Each worker threads its task until it reaches the barrier point. When a worker thread reaches the barrier, it will block until all other worker threads have reached the barrier too (i.e., when all workers have finished their tasks on that particular part). Once all workers are at the barrier, they're released from their blocking state and can move on to work on the next part.Using CyclicBarrier can be beneficial in certain scenarios:
Synchronizing independent threads: When you have multiple threads performing unrelated tasks, a CyclicBarrier ensures that none of them starts until all others have finished. Grouping parallel processing: When you have a set of tasks that need to be performed concurrently, but some tasks require others to complete before they can start, CyclicBarrier helps ensure this synchronization. Flow control in parallel algorithms: In certain algorithms where threads need to synchronize and then proceed, a CyclicBarrier provides the necessary flow control.Here's an example code snippet using Java:
import java.util.concurrent.CyclicBarrier;
public class WorkerThread {
private static final int NUM_WORKERS = 5;
private static final int PARTS_TO_ASSEMBLE = 10;
public static void main(String[] args) throws InterruptedException {
CyclicBarrier barrier = new CyclicBarrier(NUM_WORKERS);
for (int i = 0; i < NUM_WORKERS; i++) {
new Worker(i, barrier).start();
}
// Wait for all workers to finish
barrier.await();
}
}
class Worker extends Thread {
private int id;
private CyclicBarrier barrier;
public Worker(int id, CyclicBarrier barrier) {
this.id = id;
this.barrier = barrier;
}
@Override
public void run() {
for (int i = 0; i < WorkerThread.PARTS_TO_ASSEMBLE; i++) {
// Perform task on part
System.out.println("Worker " + id + " is working on part " + i);
barrier.await();
}
}
}
In this example, we create a CyclicBarrier with a count of 5 (the number of workers). Each worker thread performs tasks until it reaches the barrier point. When a worker reaches the barrier, it blocks until all other worker threads have reached the barrier too. Once all workers are at the barrier, they're released from their blocking state and can move on to work on the next part.
I hope this explanation helps you understand how CyclicBarrier works in Java!