What is @async in Java?
What is @async in Java?
I'd be happy to explain what @Async is in Java.
@Async
is an annotation in Spring that enables asynchronous execution of methods, allowing your application to process multiple requests concurrently without blocking the main thread. This feature is particularly useful when handling a high volume of requests or integrating with third-party services that require long-running operations.
In traditional synchronous programming, each method call blocks the main thread until the operation completes. However, this can lead to performance issues and decreased responsiveness in your application, especially if you're dealing with time-consuming tasks like database queries, file I/O, or network connections.
To address this limitation, Spring provides the @Async
annotation, which allows you to designate a method as asynchronous. When you annotate a method with @Async
, the framework will execute that method in a separate thread pool, allowing your application to continue processing other requests without being blocked.
Here are some key aspects of using @Async
:
TaskExecutor
bean. Callback Method: You can provide an optional callback method that will be called when the asynchronous operation completes. This allows you to perform additional logic or notify interested parties about the result. Return Value: Since the annotated method returns immediately, any return value is effectively ignored. Instead, you should use a callback method or a Future
-based approach to retrieve the result. Exception Handling: When an exception occurs in the asynchronous method, it will be propagated back to the caller. You can handle these exceptions using traditional try-catch blocks or Spring's built-in error handling mechanisms.
To demonstrate the usage of @Async
, let's consider a simple example:
@Service
public class AsyncService {
@Async
public Future processRequest(String request) {
// Perform some time-consuming operation...
return CompletableFuture.completedFuture("Operation completed");
}
}
In this example, the processRequest
method is annotated with @Async
, indicating that it should be executed asynchronously. The method returns a Future
object, which represents the result of the asynchronous operation.
To consume the @Async
method, you would use a callback approach:
@Service
public class ConsumerService {
@Autowired
private AsyncService asyncService;
public void consumeRequest(String request) {
asyncService.processRequest(request)
.thenApply(result -> System.out.println("Result: " + result));
}
}
In this example, the consumeRequest
method calls the processRequest
method and provides a callback using the thenApply
method. The callback will be executed when the asynchronous operation completes.
By utilizing @Async
in your Spring application, you can create more responsive and scalable systems that efficiently handle concurrent requests without sacrificing performance.
Await equivalent java async array
I'm happy to respond in English for this request!
Awaiting the equivalent Java async array, let's dive into the world of asynchronous programming!
In Java, you can achieve asynchronous behavior using the CompletableFuture
class, which is part of the Java 8 standard library. This class allows you to write asynchronous code that handles exceptions, provides a way to compose multiple asynchronous operations together, and offers a range of utilities for working with futures.
Here's an example of how you might use CompletableFuture
to create an async array:
import java.util.concurrent.CompletableFutures;
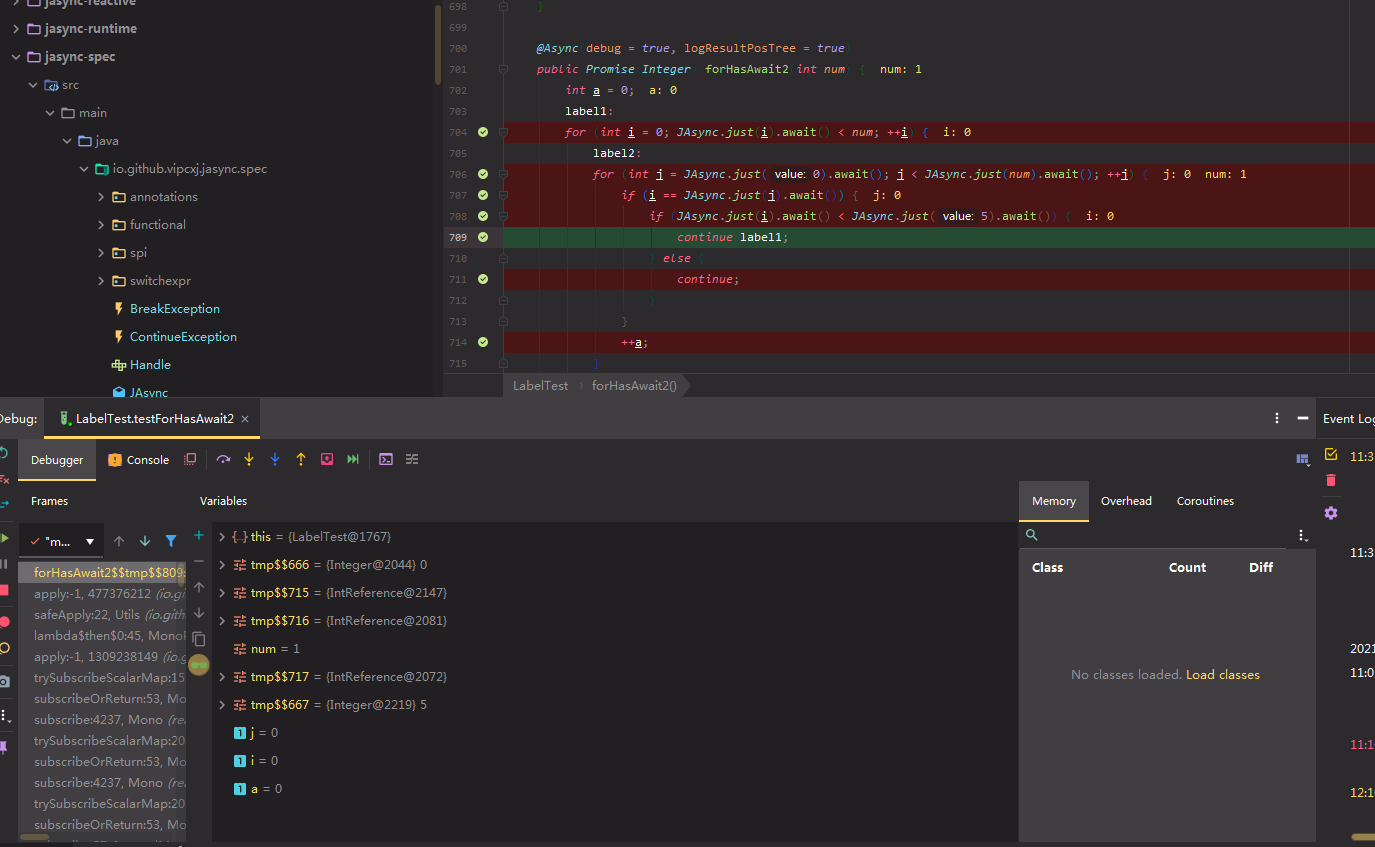
import java.util.concurrent.ExecutionException;
public class AsyncArrayExample {
public static void main(String[] args) throws ExecutionException, InterruptedException {
// Define the number of tasks to run asynchronously
int numTasks = 5;
// Create an array of futures
CompletableFuture[] futureArray = new CompletableFuture[numTasks];
for (int i = 0; i < numTasks; i++) {
futureArray[i] = CompletableFuture.supplyAsync(() -> {
// Simulate a time-consuming operation
Thread.sleep(1000);
return Math.random();
});
}
// Wait for all tasks to complete and retrieve the results
double[] results = new double[numTasks];
for (int i = 0; i < numTasks; i++) {
results[i] = futureArray[i].get();
}
System.out.println("Async array results:");
for (double result : results) {
System.out.println(result);
}
}
}
In this example, we create an CompletableFuture
array and use the supplyAsync
method to run a series of time-consuming tasks asynchronously. We then wait for all tasks to complete using the get
method, and retrieve their results as an array.
This code demonstrates the power of Java's asynchronous programming capabilities. By using CompletableFuture
, you can write robust, concurrent code that can handle exceptions and compose complex operations together. Whether you're working with arrays of futures or more complex data structures, CompletableFuture
provides a flexible framework for building scalable and efficient software systems.
I hope this helps! Do you have any questions about how to use CompletableFuture
in your own projects?