What is for each loop in Java?
What is for each loop in Java?
For Each Loop in Java:
In programming, a For Each loop (also known as an Enhanced for loop) is used to iterate over arrays or collections and execute some code for each element. It's a concise way to traverse through the elements of a collection without having to manually keep track of the index.
In Java, you can use the "for" keyword followed by the word "each" to create a For Each loop. The general syntax is:
for (type variable : arrayName/collection) {
// code to execute for each element
}
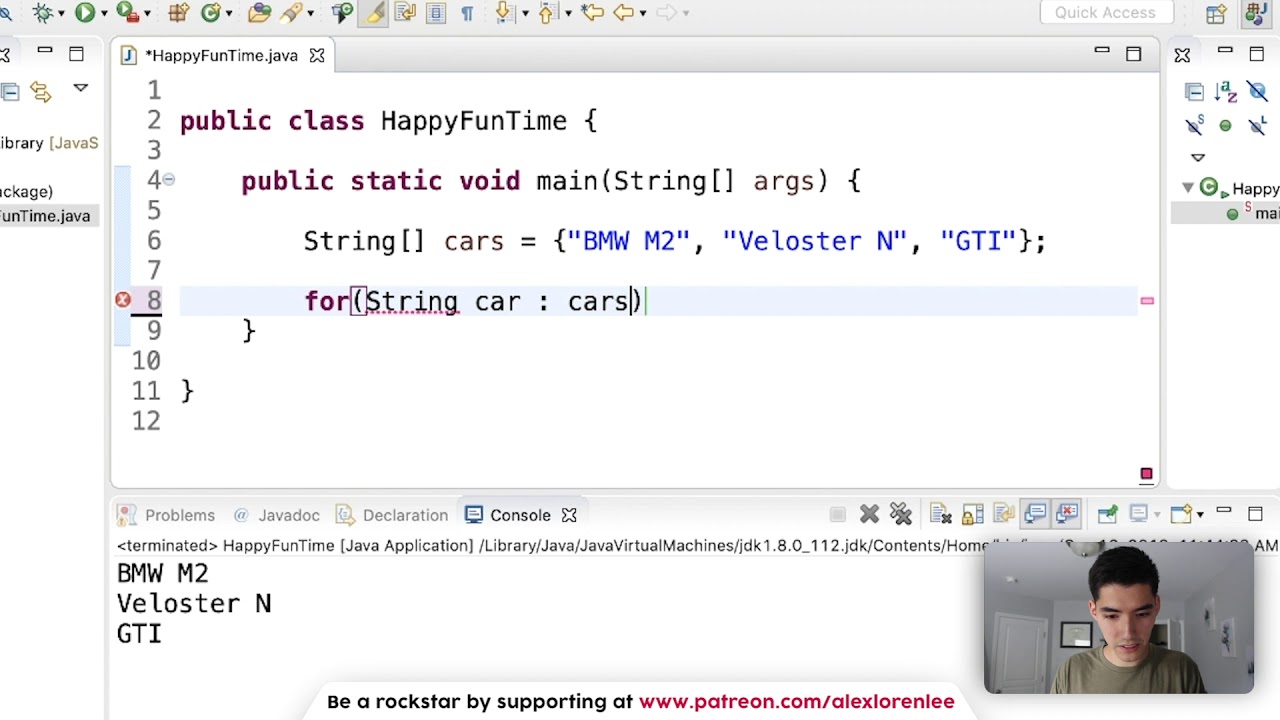
Let's break it down:
Type: This specifies the type of data that will be stored in the variable. Variable: This is the name given to the variable that will take on the value of each element in the array or collection during each iteration. ArrayName/collection: This is the name of the array or collection you want to iterate over.Here's a simple example:
String[] colors = {"Red", "Green", "Blue"};
for (String color : colors) {
System.out.println(color);
}
This will print out each color in the array on a new line. The variable color
takes on the value of each element in the array during each iteration, so you can access and manipulate it as needed.
For Each loops are particularly useful when working with collections or arrays that have many elements. They simplify the process of iterating over each element and performing some action, such as printing out values or updating data structures.
Here are some key benefits:
Concise: For Each loops are often more compact than traditional for loops. Easy to read: The code is straightforward and easy to understand, which makes it perfect for beginners. Flexibility: You can use this loop with arrays, collections (like ArrayList or HashSet), or even iterators.To illustrate this further, let's create a For Each loop that iterates over an ArrayList of integers:
ArrayList numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
for (int number : numbers) {
System.out.println(number * 2); // prints out the doubled value
}
In this example, we create an ArrayList called numbers
and add some integers to it. Then, we use a For Each loop to iterate over each element in the list. We print out each number multiplied by 2, which demonstrates how you can access and manipulate the elements during iteration.
For Each loops are a powerful tool in Java programming that simplify the process of iterating over arrays or collections. They're easy to read, concise, and flexible – making them perfect for beginners and experienced developers alike!
What are the 4 loops in java w3schools
I'm happy to help you with that! According to W3Schools, Java has four types of loops:
1. For Loop
A for loop is used when you want to execute a block of code repeatedly based on a specific condition. The syntax for a for loop in Java is as follows:
for (initialization; condition; increment) {
// code to be executed
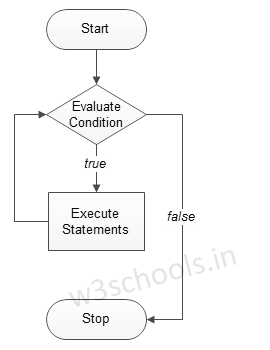
}
The initialization statement sets the starting value, the condition statement checks whether to continue or not, and the increment statement updates the variable.
Example: Print numbers from 1 to 5 using a for loop.
for (int i = 1; i <= 5; i++) {
System.out.println(i);
}
2. While Loop
A while loop is used when you want to execute a block of code repeatedly based on a specific condition. The syntax for a while loop in Java is as follows:
while (condition) {
// code to be executed
}
The condition statement checks whether to continue or not.
Example: Print numbers from 1 to 5 using a while loop.
int i = 1;
while (i <= 5) {
System.out.println(i);
i++;
}
3. Do-While Loop
A do-while loop is used when you want to execute a block of code repeatedly based on a specific condition, but the condition statement is evaluated after executing the body of the loop.
The syntax for a do-while loop in Java is as follows:
do {
// code to be executed
} while (condition);
Example: Print numbers from 1 to 5 using a do-while loop.
int i = 1;
do {
System.out.println(i);
i++;
} while (i <= 5);
4. Enhanced for Loop
An enhanced for loop is used when you want to execute a block of code repeatedly based on a specific iterable object, such as an array or collection.
The syntax for an enhanced for loop in Java is as follows:
for (variable : iterable) {
// code to be executed
}
Example: Print elements from an array using an enhanced for loop.
String[] colors = {"Red", "Green", "Blue"};
for (String color : colors) {
System.out.println(color);
}
In summary, Java provides four types of loops that allow you to execute a block of code repeatedly based on specific conditions. The choice of which type of loop to use depends on the nature of your problem and the requirements of your program.