for loop java
for loop java
I'd be happy to explain Java's for
loop to you!
The for
loop is a fundamental control structure in Java, used for executing a block of code repeatedly. It's similar to the while
loop, but with some key differences.
Here's the basic syntax:
for (initialization; condition; increment) {
// code to be executed
}
Let's break it down:
Initialization: This is where you declare and initialize a variable that will be used in the loop. Condition: This is the boolean expression that determines whether or not the loop continues. If the condition istrue
, the loop will execute; if false
, the loop will exit.
Here's a simple example:
for (int i = 0; i < 5; i++) {
System.out.println("Hello, world! " + i);
}
In this example:
i
is initialized to 0. The condition i < 5
will keep the loop running as long as i
is less than 5. Each iteration, i
increments by 1.
When you run this code, it will print "Hello, world! 0", then "Hello, world! 1", and so on up to "Hello, world! 4".
Here's another example that demonstrates the use of arrays:
int[] scores = {90, 70, 85, 95};
for (int score : scores) {
System.out.println("Score: " + score);
}
In this case:
score
is initialized to the next value in the scores
array. The condition is implicit – the loop will stop when all elements in the array have been processed. There's no increment, as we're simply iterating over the array.
When you run this code, it will print each score in the array on a separate line.
Java also supports a more concise form of the for
loop using variable declaration and initialization directly within the loop:
for (int i = 0; i < 5; i++) {
System.out.println("Hello, world! " + i);
}
This is equivalent to the previous example. The for
keyword is followed by three parts: the variable declaration and initialization (int i = 0
), the condition (i < 5
), and the increment (i++
).
In summary, Java's for
loop provides a flexible way to repeat a block of code, making it a fundamental tool in any programming project.
for-each loop java
I'm glad to help you with your inquiry about the For-Each Loop in Java!
The for-each loop, also known as the enhanced for loop or iterable loop, is a type of loop in Java that allows you to iterate over a collection (such as an array, list, set, map) without having to write explicit loop control statements. This can make your code more readable and easier to maintain.
Here's the basic syntax:
for (type variable : iterable) {
// loop body
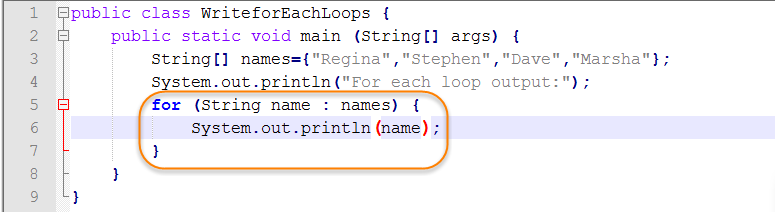
}
In this syntax:
type
is the type of the elements in the collection. variable
is the name given to each element in the collection as it is iterated over.
iterable
is the actual collection being iterated over (such as an array, list, set, map).
Here's a simple example:
int[] scores = { 85, 90, 78, 92 };
for (int score : scores) {
System.out.println("Score: " + score);
}
In this example, the for-each loop iterates over each element in the scores
array and assigns it to the score
variable. The loop body prints out the value of each score.
Another important aspect of the for-each loop is that you can't access the index of the element being processed. This can be a limitation if you need to do something based on the index, like printing out both the index and the element.
Here's an example of how you might overcome this by using an ordinary for
loop with an index variable:
int[] scores = { 85, 90, 78, 92 };
for (int i = 0; i < scores.length; i++) {
System.out.println("Index: " + i + ", Score: " + scores[i]);
}
In this example, the loop body uses the i
variable to access both the index and the element.
One more thing to note is that you can use for-each loops with various types of collections, including arrays, lists, sets, maps, and even custom iterators! This makes it a very versatile and powerful construct in Java programming.
In conclusion, the for-each loop is a valuable tool in your Java programming toolbox, offering a concise and readable way to iterate over collections. With its simplicity and flexibility, you'll find yourself using it again and again as you continue to work with Java!