When should we use try catch in java with example
When should we use try catch in java with example
I'm happy to respond in English for you!
In Java, the try-catch block is used to handle exceptions that might occur while executing a piece of code. An exception is an event that occurs during the execution of a program that disrupts the normal flow of instructions.
You should use try-catch blocks when:
Handling runtime errors: When your code attempts to divide by zero, access an out-of-bound array element, or perform some other operation that will throw a runtime error. Processing user input: When you're working with user input, such as reading from a file or parsing user-provided data. If the user provides invalid input, you might want to catch and handle that exception. Handling unexpected situations: When your code encounters an unexpected situation, like a network connection failure or a database query timing out.Here's an example of using try-catch in Java:
public class TryCatchExample {
public static void main(String[] args) {
int denominator = 0;
try {
int result = 10 / denominator; // Attempting to divide by zero
System.out.println("The result is: " + result);
} catch (ArithmeticException e) {
System.out.println("Caught an exception: " + e.getMessage());
// Handle the error, perhaps by printing a message or retrying the operation
}
}
}
In this example, we're attempting to divide 10 by zero. The try
block contains the code that might throw an exception (in this case, a ArithmeticException
). If an exception is thrown, it's caught by the catch
block and handled accordingly.
When an exception is thrown in the try
block, Java looks for the nearest matching catch block to handle the exception. In our example, when the division by zero occurs, the ArithmeticException
is caught, and we print a message indicating that an exception was caught.
Remember to always include a finally block if you're performing some cleanup or logging after an exception has been thrown.
Best Practices:
Catch specific exceptions whenever possible. Avoid catching the generalException
class, as it can catch unexpected errors. Instead, catch the most specific exception type that makes sense for your code. Log the caught exception to help with debugging and error reporting. Don't ignore or swallow exceptions; this can lead to unexpected program behavior or data corruption.
I hope this helps clarify when you should use try-catch in Java!
When to use try catch vs if in Java?
When it comes to choosing between using try-catch
blocks and simple if-else
statements in Java, it's essential to understand the fundamental differences between these two constructs.
If-Else Statements:
In Java, an if-else
statement is used to execute different code paths based on a specific condition. It's like asking the computer "Should I do this or that?" If the condition is true, the code inside the if
block will be executed; otherwise, the code in the else
block (if present) will run.
Here's an example:
int x = 5;
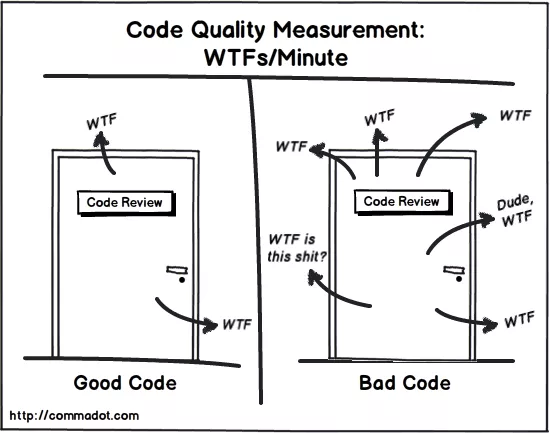
if (x > 10) {
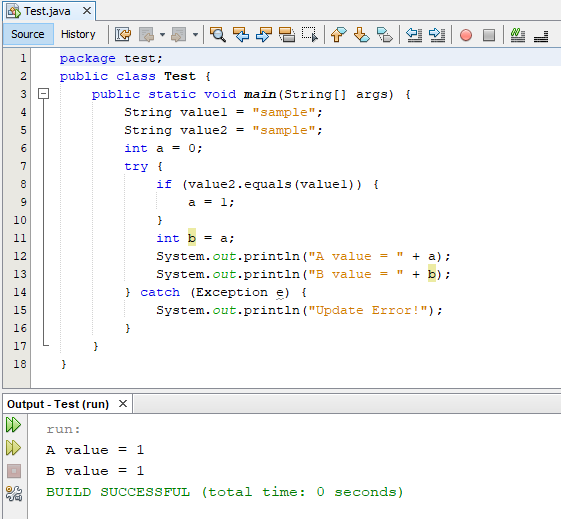
System.out.println("X is greater than 10");
} else {
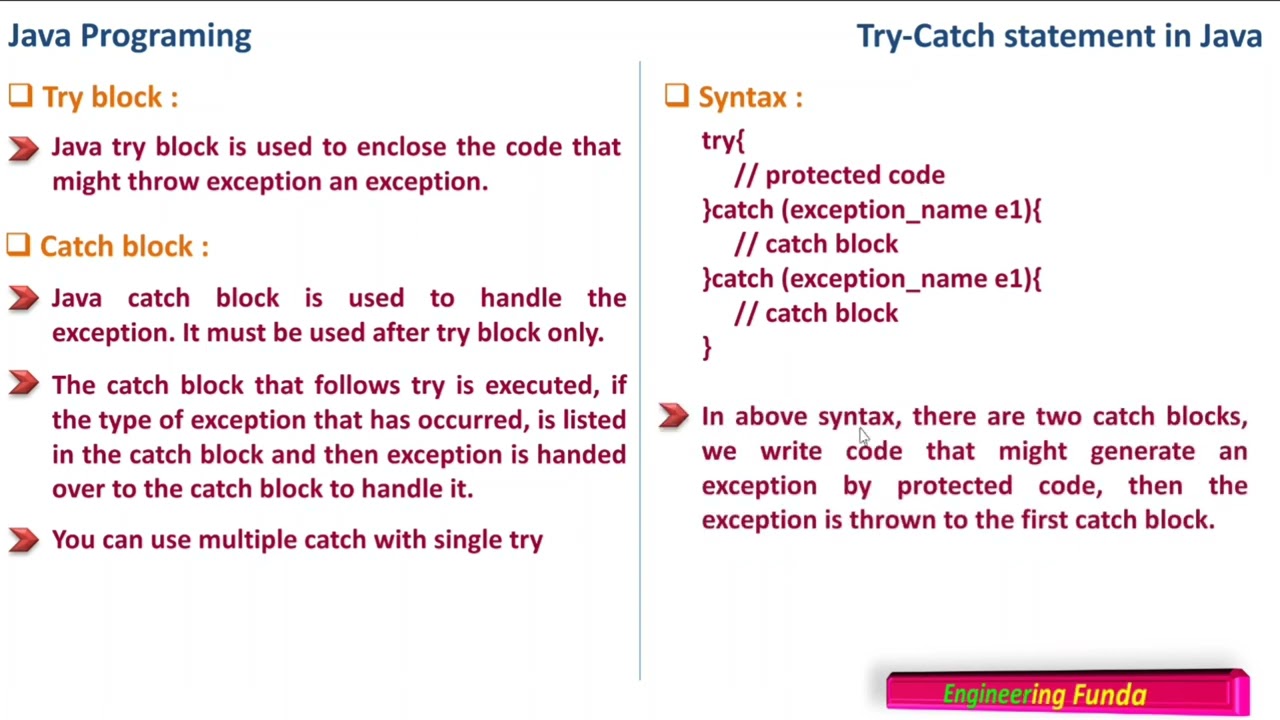
System.out.println("X is less than or equal to 10");
}
In this example, the condition x > 10
is evaluated. Since it's false, the code in the else
block will be executed.
Try-Catch Blocks:
A try-catch
block is used to handle exceptions that might occur during the execution of a piece of code. It's like saying "Hey, I know something unexpected might happen here, so let me prepare for it."
Here's an example:
try {
int x = 5;
System.out.println(1 / x);
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero!");
}
In this example, the code inside the try
block attempts to perform a division operation. However, since x
is zero, an ArithmeticException
is thrown. The catch
block will handle this exception and print a friendly message.
When to Use Try-Catch vs If:
So, when should you use try-catch
blocks instead of simple if-else
statements?
try-catch
block to handle these situations. Uncertainty: When the condition for execution is uncertain or prone to errors, consider using a try-catch
block to catch unexpected issues. Code robustness: Using try-catch
blocks helps make your code more robust by anticipating potential problems and providing a plan for handling them.
On the other hand, use if-else
statements when:
if-else
statement is sufficient. No exceptions: If the code inside your if
block won't throw any exceptions or errors, a simple if-else
statement will do.
In summary, use try-catch
blocks when working with exceptions, uncertain conditions, or wanting to add robustness to your code. For simple, predictable conditional logic, stick with if-else
statements.
I hope this helps clarify the differences between these two constructs in Java!